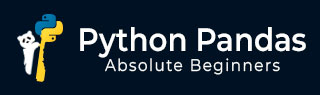
- Python pandas教程
- Python Pandas - 主页
- Python Pandas - 简介
- Python Pandas - 环境设置
- 数据结构简介
- Python pandas - 系列
- Python Pandas - 数据帧
- Python Pandas - 面板
- Python Pandas - 基本功能
- 描述性统计
- 功能应用
- Python Pandas - 重新索引
- Python Pandas - 迭代
- Python Pandas - 排序
- 处理文本数据
- 选项和定制
- 索引和选择数据
- 统计功能
- Python Pandas - 窗口函数
- Python Pandas - 聚合
- Python Pandas - 缺失数据
- Python Pandas - GroupBy
- Python Pandas - 合并/连接
- Python Pandas - 连接
- Python Pandas - 日期功能
- Python Pandas - Timedelta
- Python Pandas - 分类数据
- Python Pandas - 可视化
- Python Pandas - IO 工具
- Python Pandas - 稀疏数据
- Python Pandas - 注意事项和陷阱
- 与SQL的比较
- Python Pandas 有用资源
- Python Pandas - 快速指南
- Python Pandas - 有用的资源
- Python Pandas - 讨论
Python Pandas - 处理文本数据
在本章中,我们将讨论基本系列/索引的字符串操作。在后续章节中,我们将学习如何在 DataFrame 上应用这些字符串函数。
Pandas 提供了一组字符串函数,可以轻松地对字符串数据进行操作。最重要的是,这些函数忽略(或排除)缺失/NaN 值。
几乎所有这些方法都适用于 Python 字符串函数(请参阅:https ://docs.python.org/3/library/stdtypes.html#string-methods )。因此,将Series对象转换为String对象,然后执行操作。
现在让我们看看每个操作的执行情况。
先生编号 | 功能说明 |
---|---|
1 |
降低() 将系列/索引中的字符串转换为小写。 |
2 |
上() 将系列/索引中的字符串转换为大写。 |
3 |
长度() 计算字符串长度()。 |
4 |
条() 帮助从两侧去除系列/索引中每个字符串中的空格(包括换行符)。 |
5 |
分裂(' ') 使用给定的模式分割每个字符串。 |
6 |
猫(九月='') 使用给定的分隔符连接系列/索引元素。 |
7 |
get_dummies() 返回具有 One-Hot 编码值的 DataFrame。 |
8 |
包含(模式) 如果子字符串包含在元素中,则为每个元素返回布尔值 True,否则返回 False。 |
9 |
替换(a,b) 将值a替换为值b。 |
10 |
重复(值) 将每个元素重复指定的次数。 |
11 |
计数(模式) 返回每个元素中模式出现的计数。 |
12 |
以(模式)开头 如果系列/索引中的元素以模式开头,则返回 true。 |
13 |
以(模式)结尾 如果系列/索引中的元素以模式结尾,则返回 true。 |
14 |
查找(模式) 返回模式第一次出现的第一个位置。 |
15 |
查找全部(模式) 返回所有出现的模式的列表。 |
16 |
交换箱 交换外壳下/上。 |
17 号 |
islower() 检查系列/索引中每个字符串中的所有字符是否均为小写。返回布尔值 |
18 |
isupper() 检查系列/索引中每个字符串中的所有字符是否均为大写。返回布尔值。 |
19 |
是数字() 检查系列/索引中每个字符串中的所有字符是否都是数字。返回布尔值。 |
现在让我们创建一个系列并看看上述所有功能是如何工作的。
import pandas as pd import numpy as np s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t', np.nan, '1234','SteveSmith']) print s
其输出如下 -
0 Tom 1 William Rick 2 John 3 Alber@t 4 NaN 5 1234 6 Steve Smith dtype: object
降低()
import pandas as pd import numpy as np s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t', np.nan, '1234','SteveSmith']) print s.str.lower()
其输出如下 -
0 tom 1 william rick 2 john 3 alber@t 4 NaN 5 1234 6 steve smith dtype: object
上()
import pandas as pd import numpy as np s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t', np.nan, '1234','SteveSmith']) print s.str.upper()
其输出如下 -
0 TOM 1 WILLIAM RICK 2 JOHN 3 ALBER@T 4 NaN 5 1234 6 STEVE SMITH dtype: object
长度()
import pandas as pd import numpy as np s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t', np.nan, '1234','SteveSmith']) print s.str.len()
其输出如下 -
0 3.0 1 12.0 2 4.0 3 7.0 4 NaN 5 4.0 6 10.0 dtype: float64
条()
import pandas as pd import numpy as np s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s print ("After Stripping:") print s.str.strip()
其输出如下 -
0 Tom 1 William Rick 2 John 3 Alber@t dtype: object After Stripping: 0 Tom 1 William Rick 2 John 3 Alber@t dtype: object
分割(图案)
import pandas as pd import numpy as np s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s print ("Split Pattern:") print s.str.split(' ')
其输出如下 -
0 Tom 1 William Rick 2 John 3 Alber@t dtype: object Split Pattern: 0 [Tom, , , , , , , , , , ] 1 [, , , , , William, Rick] 2 [John] 3 [Alber@t] dtype: object
猫(sep=模式)
import pandas as pd import numpy as np s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s.str.cat(sep='_')
其输出如下 -
Tom _ William Rick_John_Alber@t
get_dummies()
import pandas as pd import numpy as np s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s.str.get_dummies()
其输出如下 -
William Rick Alber@t John Tom 0 0 0 0 1 1 1 0 0 0 2 0 0 1 0 3 0 1 0 0
包含 ()
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s.str.contains(' ')
其输出如下 -
0 True 1 True 2 False 3 False dtype: bool
替换(a,b)
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s print ("After replacing @ with $:") print s.str.replace('@','$')
其输出如下 -
0 Tom 1 William Rick 2 John 3 Alber@t dtype: object After replacing @ with $: 0 Tom 1 William Rick 2 John 3 Alber$t dtype: object
重复(值)
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s.str.repeat(2)
其输出如下 -
0 Tom Tom 1 William Rick William Rick 2 JohnJohn 3 Alber@tAlber@t dtype: object
计数(模式)
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print ("The number of 'm's in each string:") print s.str.count('m')
其输出如下 -
The number of 'm's in each string: 0 1 1 1 2 0 3 0
以(模式)开头
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print ("Strings that start with 'T':") print s.str. startswith ('T')
其输出如下 -
0 True 1 False 2 False 3 False dtype: bool
以(模式)结尾
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print ("Strings that end with 't':") print s.str.endswith('t')
其输出如下 -
Strings that end with 't': 0 False 1 False 2 False 3 True dtype: bool
查找(模式)
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s.str.find('e')
其输出如下 -
0 -1 1 -1 2 -1 3 3 dtype: int64
“-1”表示元素中没有可用的模式。
查找全部(模式)
import pandas as pd s = pd.Series(['Tom ', ' William Rick', 'John', 'Alber@t']) print s.str.findall('e')
其输出如下 -
0 [] 1 [] 2 [] 3 [e] dtype: object
空列表([ ]) 表示元素中没有可用的此类模式。
交换案例()
import pandas as pd s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t']) print s.str.swapcase()
其输出如下 -
0 tOM 1 wILLIAM rICK 2 jOHN 3 aLBER@T dtype: object
islower()
import pandas as pd s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t']) print s.str.islower()
其输出如下 -
0 False 1 False 2 False 3 False dtype: bool
isupper()
import pandas as pd s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t']) print s.str.isupper()
其输出如下 -
0 False 1 False 2 False 3 False dtype: bool
是数字()
import pandas as pd s = pd.Series(['Tom', 'William Rick', 'John', 'Alber@t']) print s.str.isnumeric()
其输出如下 -
0 False 1 False 2 False 3 False dtype: bool