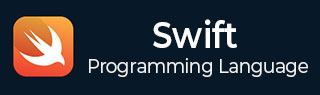
- 快速教程
- 斯威夫特 - 主页
- 斯威夫特 - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 数据类型
- Swift - 变量
- Swift - 可选
- Swift - 元组
- Swift - 常量
- Swift - 文字
- Swift - 运算符
- Swift - 决策
- Swift - 循环
- Swift - 字符串
- 斯威夫特 - 角色
- Swift - 数组
- Swift - 套装
- 斯威夫特 - 字典
- Swift - 函数
- Swift - 闭包
- Swift - 枚举
- Swift - 结构
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 初始化
- Swift - 去初始化
- Swift - ARC 概述
- Swift - 可选链接
- Swift - 类型转换
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- 斯威夫特有用的资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用的资源
- 斯威夫特 - 讨论
Swift - 泛型
Swift 4 语言提供“通用”功能来编写灵活且可重用的函数和类型。泛型用于避免重复并提供抽象。Swift 4 标准库是使用泛型代码构建的。Swift 4 的“数组”和“字典”类型属于泛型集合。在数组和字典的帮助下,数组被定义为保存“Int”值和“String”值或任何其他类型。
func exchange(a: inout Int, b: inout Int) { let temp = a a = b b = temp } var numb1 = 100 var numb2 = 200 print("Before Swapping values are: \(numb1) and \(numb2)") exchange(a: &numb1, b: &numb2) print("After Swapping values are: \(numb1) and \(numb2)")
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
Before Swapping values are: 100 and 200 After Swapping values are: 200 and 100
通用函数:类型参数
通用函数可用于访问任何数据类型,例如“Int”或“String”。
func exchange<T>(a: inout T, b: inout T) { let temp = a a = b b = temp } var numb1 = 100 var numb2 = 200 print("Before Swapping Int values are: \(numb1) and \(numb2)") exchange(a: &numb1, b: &numb2) print("After Swapping Int values are: \(numb1) and \(numb2)") var str1 = "Generics" var str2 = "Functions" print("Before Swapping String values are: \(str1) and \(str2)") exchange(a: &str1, b: &str2) print("After Swapping String values are: \(str1) and \(str2)")
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
Before Swapping Int values are: 100 and 200 After Swapping Int values are: 200 and 100 Before Swapping String values are: Generics and Functions After Swapping String values are: Functions and Generics
函数exchange()用于交换上面程序中描述的值,<T>用作类型参数。第一次调用函数交换()以返回“Int”值,第二次调用函数交换()将返回“字符串”值。尖括号内可以包含多个参数类型,并用逗号分隔。
类型参数按用户定义命名,以了解其所保存的类型参数的用途。Swift 4 提供 <T> 作为泛型类型参数名称。然而,像数组和字典这样的类型参数也可以命名为键、值,以标识它们属于“字典”类型。
struct TOS<T> { var items = [T]() mutating func push(item: T) { items.append(item) } mutating func pop() -> T { return items.removeLast() } } var tos = TOS<String>() tos.push(item: "Swift 4") print(tos.items) tos.push(item: "Generics") print(tos.items) tos.push(item: "Type Parameters") print(tos.items) tos.push(item: "Naming Type Parameters") print(tos.items) let deletetos = tos.pop()
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
[Swift 4] [Swift 4, Generics] [Swift 4, Generics, Type Parameters] [Swift 4, Generics, Type Parameters, Naming Type Parameters]
扩展泛型类型
扩展 stack 属性以了解该项目的顶部包含在“extension”关键字中。
struct TOS<T> { var items = [T]() mutating func push(item: T) { items.append(item) } mutating func pop() -> T { return items.removeLast() } } var tos = TOS<String>() tos.push(item: "Swift 4") print(tos.items) tos.push(item: "Generics") print(tos.items) tos.push(item: "Type Parameters") print(tos.items) tos.push(item: "Naming Type Parameters") print(tos.items) extension TOS { var first: T? { return items.isEmpty ? nil : items[items.count - 1] } } if let first = tos.first { print("The top item on the stack is \(first).") }
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
["Swift 4"] ["Swift 4", "Generics"] ["Swift 4", "Generics", "Type Parameters"] ["Swift 4", "Generics", "Type Parameters", "Naming Type Parameters"] The top item on the stack is Naming Type Parameters.
类型约束
Swift 4 语言允许“类型约束”来指定类型参数是否继承自特定类,或者确保协议符合标准。
func exchange<T>(a: inout T, b: inout T) { let temp = a a = b b = temp } var numb1 = 100 var numb2 = 200 print("Before Swapping Int values are: \(numb1) and \(numb2)") exchange(a: &numb1, b: &numb2) print("After Swapping Int values are: \(numb1) and \(numb2)") var str1 = "Generics" var str2 = "Functions" print("Before Swapping String values are: \(str1) and \(str2)") exchange(a: &str1, b: &str2) print("After Swapping String values are: \(str1) and \(str2)")
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
Before Swapping Int values are: 100 and 200 After Swapping Int values are: 200 and 100 Before Swapping String values are: Generics and Functions After Swapping String values are: Functions and Generics
相关类型
Swift 4 允许在协议定义中通过关键字“linkedtype”声明关联类型。
protocol Container { associatedtype ItemType mutating func append(item: ItemType) var count: Int { get } subscript(i: Int) -> ItemType { get } } struct TOS<T>: Container { // original Stack<T> implementation var items = [T]() mutating func push(item: T) { items.append(item) } mutating func pop() -> T { return items.removeLast() } // conformance to the Container protocol mutating func append(item: T) { self.push(item: item) } var count: Int { return items.count } subscript(i: Int) -> T { return items[i] } } var tos = TOS<String>() tos.push(item: "Swift 4") print(tos.items) tos.push(item: "Generics") print(tos.items) tos.push(item: "Type Parameters") print(tos.items) tos.push(item: "Naming Type Parameters") print(tos.items)
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
[Swift 4] [Swift 4, Generics] [Swift 4, Generics, Type Parameters] [Swift 4, Generics, Type Parameters, Naming Type Parameters]
Where 子句
类型约束使用户能够定义与泛型函数或类型关联的类型参数的要求。为了定义关联类型的要求,“where”子句被声明为类型参数列表的一部分。“where”关键字紧接在类型参数列表之后,后跟关联类型的约束、类型与关联类型之间的相等关系。
protocol Container { associatedtype ItemType mutating func append(item: ItemType) var count: Int { get } subscript(i: Int) -> ItemType { get } } struct Stack<T>: Container { // original Stack<T> implementation var items = [T]() mutating func push(item: T) { items.append(item) } mutating func pop() -> T { return items.removeLast() } // conformance to the Container protocol mutating func append(item: T) { self.push(item: item) } var count: Int { return items.count } subscript(i: Int) -> T { return items[i] } } func allItemsMatch< C1: Container, C2: Container where C1.ItemType == C2.ItemType, C1.ItemType: Equatable> (someContainer: C1, anotherContainer: C2) -> Bool { // check that both containers contain the same number of items if someContainer.count != anotherContainer.count { return false } // check each pair of items to see if they are equivalent for i in 0..<someContainer.count { if someContainer[i] != anotherContainer[i] { return false } } // all items match, so return true return true } var tos = Stack<String>() tos.push(item: "Swift 4") print(tos.items) tos.push(item: "Generics") print(tos.items) tos.push(item: "Where Clause") print(tos.items) var eos = ["Swift 4", "Generics", "Where Clause"] print(eos)
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
[Swift 4] [Swift 4, Generics] [Swift 4, Generics, Where Clause] [Swift 4, Generics, Where Clause]