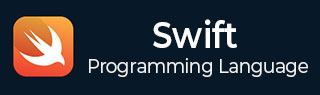
- 快速教程
- 斯威夫特 - 主页
- 斯威夫特 - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 数据类型
- Swift - 变量
- Swift - 可选
- Swift - 元组
- Swift - 常量
- Swift - 文字
- Swift - 运算符
- Swift - 决策
- Swift - 循环
- Swift - 字符串
- 斯威夫特 - 角色
- Swift - 数组
- Swift - 套装
- 斯威夫特 - 字典
- Swift - 函数
- Swift - 闭包
- Swift - 枚举
- Swift - 结构
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 初始化
- Swift - 去初始化
- Swift - ARC 概述
- Swift - 可选链接
- Swift - 类型转换
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- 斯威夫特有用的资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用的资源
- 斯威夫特 - 讨论
Swift - 方法
在 Swift 4 语言中,与特定类型关联的函数称为方法。在 Objective C 中,类用于定义方法,而 Swift 4 语言为用户提供了灵活性,可以为类、结构体和枚举提供方法。
实例方法
在 Swift 4 语言中,类、结构体和枚举实例是通过实例方法访问的。
实例方法提供功能
- 访问和修改实例属性
- 与实例需求相关的功能
实例方法可以写在{}大括号内。它可以隐式访问类型实例的方法和属性。当调用该类型的特定实例时,它将访问该特定实例。
句法
func funcname(Parameters) -> returntype { Statement1 Statement2 --- Statement N return parameters }
例子
class calculations { let a: Int let b: Int let res: Int init(a: Int, b: Int) { self.a = a self.b = b res = a + b } func tot(c: Int) -> Int { return res - c } func result() { print("Result is: \(tot(c: 20))") print("Result is: \(tot(c: 50))") } } let pri = calculations(a: 600, b: 300) pri.result()
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
Result is: 880 Result is: 850
类计算定义了两个实例方法 -
- init() 被定义为将两个数字 a 和 b 相加并将其存储在结果“res”中
- tot() 用于从传递的“c”值中减去“res”
最后,调用打印带有 a 和 b 值的计算方法。实例方法通过“.”访问 点语法
本地和外部参数名称
Swift 4 函数描述了其变量的本地和全局声明。同样,Swift 4 方法的命名约定也与 Objective C 类似。但是函数和方法的局部和全局参数名称声明的特征是不同的。Swift 4 中的第一个参数通过介词名称引用为“with”、“for”和“by”,以便于访问命名约定。
Swift 4 通过将第一个参数名称声明为本地参数名称,并将其余参数名称声明为全局参数名称,提供了方法的灵活性。这里 'no1' 被 Swift 4 方法声明为本地参数名称。“no2”用于全局声明并在整个程序中访问。
class division { var count: Int = 0 func incrementBy(no1: Int, no2: Int) { count = no1 / no2 print(count) } } let counter = division() counter.incrementBy(no1: 1800, no2: 3) counter.incrementBy(no1: 1600, no2: 5) counter.incrementBy(no1: 11000, no2: 3)
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
600 320 3666
带 # 和 _ 符号的外部参数名称
尽管 Swift 4 方法为本地声明提供了第一个参数名称,但用户可以将参数名称从本地声明修改为全局声明。这可以通过在第一个参数名称前添加“#”符号来完成。通过这样做,可以在整个模块中全局访问第一个参数。
当用户需要使用外部名称访问后续参数名称时,方法名称将在“_”符号的帮助下被覆盖。
class multiplication { var count: Int = 0 func incrementBy(no1: Int, no2: Int) { count = no1 * no2 print(count) } } let counter = multiplication() counter.incrementBy(no1: 800, no2: 3) counter.incrementBy(no1: 100, no2: 5) counter.incrementBy(no1: 15000, no2: 3)
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
2400 500 45000
方法中的自属性
方法对于其所有定义的类型实例都有一个称为“self”的隐式属性。“Self”属性用于引用当前实例的已定义方法。
class calculations { let a: Int let b: Int let res: Int init(a: Int, b: Int) { self.a = a self.b = b res = a + b print("Inside Self Block: \(res)") } func tot(c: Int) -> Int { return res - c } func result() { print("Result is: \(tot(c: 20))") print("Result is: \(tot(c: 50))") } } let pri = calculations(a: 600, b: 300) let sum = calculations(a: 1200, b: 300) pri.result() sum.result()
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
Inside Self Block: 900 Inside Self Block: 1500 Result is: 880 Result is: 850 Result is: 1480 Result is: 1450
从实例方法修改值类型
在 Swift 4 语言中,结构和枚举属于值类型,不能通过其实例方法进行更改。然而,Swift 4 语言提供了通过“变异”Behave来修改值类型的灵活性。Mutate 会对实例方法进行任何更改,并在方法执行后返回到原始形式。此外,通过“self”属性为其隐式函数创建新实例,并将在执行后替换现有方法
struct area { var length = 1 var breadth = 1 func area() -> Int { return length * breadth } mutating func scaleBy(res: Int) { length *= res breadth *= res print(length) print(breadth) } } var val = area(length: 3, breadth: 5) val.scaleBy(res: 3) val.scaleBy(res: 30) val.scaleBy(res: 300)
当我们使用 Playground 运行上述程序时,我们得到以下结果 -
9 15 270 450 81000 135000
变异方法的自属性
改变方法与“self”属性相结合,将一个新实例分配给定义的方法。
struct area { var length = 1 var breadth = 1 func area() -> Int { return length * breadth } mutating func scaleBy(res: Int) { self.length *= res self.breadth *= res print(length) print(breadth) } } var val = area(length: 3, breadth: 5) val.scaleBy(res: 13)
当我们使用 Playground 运行上述程序时,我们得到以下结果。-
39 65
类型方法
当调用某个方法的特定实例时,它被称为实例方法;当该方法调用特定类型的方法时,它被称为“类型方法”。“类”的类型方法由“func”关键字定义,结构和枚举类型方法由“func”关键字之前的“static”关键字定义。
类型方法通过“.”调用和访问。语法中,不是调用特定实例,而是调用整个方法。
class Math { class func abs(number: Int) -> Int { if number < 0 { return (-number) } else { return number } } } struct absno { static func abs(number: Int) -> Int { if number < 0 { return (-number) } else { return number } } } let no = Math.abs(number: -35) let num = absno.abs(number: -5) print(no) print(num)
当我们使用 Playground 运行上述程序时,我们得到以下结果。-
35 5