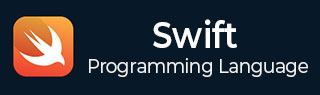
- 快速教程
- 斯威夫特 - 主页
- 斯威夫特 - 概述
- Swift - 环境
- Swift - 基本语法
- Swift - 数据类型
- Swift - 变量
- Swift - 可选
- Swift - 元组
- Swift - 常量
- Swift - 文字
- Swift - 运算符
- Swift - 决策
- Swift - 循环
- Swift - 字符串
- 斯威夫特 - 角色
- Swift - 数组
- Swift - 套装
- 斯威夫特 - 字典
- Swift - 函数
- Swift - 闭包
- Swift - 枚举
- Swift - 结构
- Swift - 类
- Swift - 属性
- Swift - 方法
- Swift - 下标
- Swift - 继承
- Swift - 初始化
- Swift - 去初始化
- Swift - ARC 概述
- Swift - 可选链接
- Swift - 类型转换
- Swift - 扩展
- Swift - 协议
- Swift - 泛型
- Swift - 访问控制
- 斯威夫特有用的资源
- Swift - 在线编译
- Swift - 快速指南
- Swift - 有用的资源
- 斯威夫特 - 讨论
Swift - Switch 语句
Swift 4 中的 switch 语句会在第一个匹配的 case 完成后立即完成执行,而不是像 C 和 C++ 编程语言中那样从后续 case 的底部掉下来。以下是 C 和 C++ 中 switch 语句的通用语法 -
switch(expression){ case constant-expression : statement(s); break; /* optional */ case constant-expression : statement(s); break; /* optional */ /* you can have any number of case statements */ default : /* Optional */ statement(s); }
这里我们需要使用break语句来跳出case语句,否则执行控制将通过下面可用的后续case语句来匹配case语句。
句法
以下是 Swift 4 中可用的 switch 语句的通用语法 -
switch expression { case expression1 : statement(s) fallthrough /* optional */ case expression2, expression3 : statement(s) fallthrough /* optional */ default : /* Optional */ statement(s); }
如果我们不使用fallthrough语句,那么程序在执行完匹配的case语句后就会跳出switch语句。我们将通过以下两个示例来清楚地说明其功能。
实施例1
以下是 Swift 4 编程中不使用 Fallthrough 的 switch 语句的示例 -
var index = 10 switch index { case 100 : print( "Value of index is 100") case 10,15 : print( "Value of index is either 10 or 15") case 5 : print( "Value of index is 5") default : print( "default case") }
当上面的代码被编译并执行时,它会产生以下结果 -
Value of index is either 10 or 15
实施例2
以下是 Swift 4 编程中带有 Fallthrough 的 switch 语句的示例 -
var index = 10 switch index { case 100 : print( "Value of index is 100") fallthrough case 10,15 : print( "Value of index is either 10 or 15") fallthrough case 5 : print( "Value of index is 5") default : print( "default case") }
当上面的代码被编译并执行时,它会产生以下结果 -
Value of index is either 10 or 15 Value of index is 5
swift_decision_making.htm