
- Arduino教程
- Arduino - 主页
- Arduino - 概述
- Arduino - 板描述
- Arduino - 安装
- Arduino - 程序结构
- Arduino - 数据类型
- Arduino - 变量和常量
- Arduino - 操作员
- Arduino - 控制语句
- Arduino - 循环
- Arduino - 功能
- Arduino - 字符串
- Arduino - 字符串对象
- Arduino - 时间
- Arduino - 数组
- Arduino 函数库
- Arduino - I/O 函数
- Arduino - 高级 I/O 功能
- Arduino - 字符函数
- Arduino - 数学库
- Arduino - 三角函数
- Arduino高级版
- Arduino - 到期与归零
- Arduino - 脉宽调制
- Arduino - 随机数
- Arduino - 中断
- Arduino - 通信
- Arduino - 内部集成电路
- Arduino - 串行外设接口
- Arduino 项目
- Arduino - LED 闪烁
- Arduino - LED 褪色
- Arduino - 读取模拟电压
- Arduino - LED 条形图
- Arduino - 键盘注销
- Arduino - 键盘消息
- Arduino - 鼠标按钮控制
- Arduino - 键盘串口
- Arduino 传感器
- Arduino - 湿度传感器
- Arduino - 温度传感器
- Arduino - 水检测器/传感器
- Arduino - PIR 传感器
- Arduino - 超声波传感器
- Arduino - 连接开关
- 电机控制
- Arduino - 直流电机
- Arduino - 伺服电机
- Arduino - 步进电机
- Arduino 和声音
- Arduino - 音调库
- Arduino - 无线通信
- Arduino - 网络通信
- Arduino 有用资源
- Arduino - 快速指南
- Arduino - 有用的资源
- Arduino - 讨论
Arduino - 键盘注销
当 ARDUINO UNO 上的引脚 2 接地时,此示例使用键盘库让您退出计算机上的用户会话。该草图同时模拟按顺序按下两个或三个键,并在短暂延迟后释放它们。
警告- 当您使用Keyboard.print()命令时,Arduino 会接管您计算机的键盘。为了确保在使用此函数运行草图时不会失去对计算机的控制,请在调用 Keyboard.print() 之前设置可靠的控制系统。该草图旨在仅在引脚接地后发送键盘命令。
所需组件
您将需要以下组件 -
- 1 × 面包板
- 1 × Arduino Leonardo、Micro 或 Due 板
- 1 × 按钮
- 1 × 跳线
程序
按照电路图并将组件连接到面包板上,如下图所示。

草图
在计算机上打开 Arduino IDE 软件。使用 Arduino 语言进行编码将控制您的电路。单击“新建”打开一个新的草图文件。
对于本示例,您需要使用 Arduino IDE 1.6.7
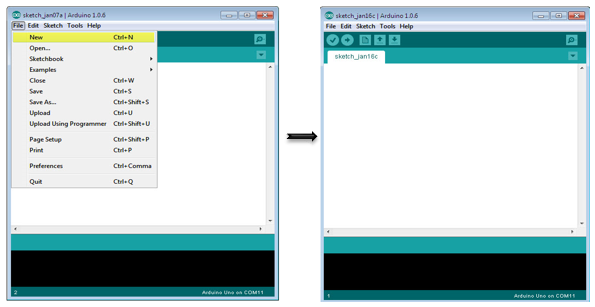
注意- 您必须在 Arduino 库文件中包含键盘库。将键盘库文件复制并粘贴到名称为库(突出显示)的文件中,如以下屏幕截图所示。

Arduino代码
/* Keyboard logout This sketch demonstrates the Keyboard library. When you connect pin 2 to ground, it performs a logout. It uses keyboard combinations to do this, as follows: On Windows, CTRL-ALT-DEL followed by ALT-l On Ubuntu, CTRL-ALT-DEL, and ENTER On OSX, CMD-SHIFT-q To wake: Spacebar. Circuit: * Arduino Leonardo or Micro * wire to connect D2 to ground. */ #define OSX 0 #define WINDOWS 1 #define UBUNTU 2 #include "Keyboard.h" // change this to match your platform: int platform = WINDOWS; void setup() { // make pin 2 an input and turn on the // pullup resistor so it goes high unless // connected to ground: pinMode(2, INPUT_PULLUP); Keyboard.begin(); } void loop() { while (digitalRead(2) == HIGH) { // do nothing until pin 2 goes low delay(500); } delay(1000); switch (platform) { case OSX: Keyboard.press(KEY_LEFT_GUI); // Shift-Q logs out: Keyboard.press(KEY_LEFT_SHIFT); Keyboard.press('Q'); delay(100); // enter: Keyboard.write(KEY_RETURN); break; case WINDOWS: // CTRL-ALT-DEL: Keyboard.press(KEY_LEFT_CTRL); Keyboard.press(KEY_LEFT_ALT); Keyboard.press(KEY_DELETE); delay(100); Keyboard.releaseAll(); //ALT-l: delay(2000); Keyboard.press(KEY_LEFT_ALT); Keyboard.press('l'); Keyboard.releaseAll(); break; case UBUNTU: // CTRL-ALT-DEL: Keyboard.press(KEY_LEFT_CTRL); Keyboard.press(KEY_LEFT_ALT); Keyboard.press(KEY_DELETE); delay(1000); Keyboard.releaseAll(); // Enter to confirm logout: Keyboard.write(KEY_RETURN); break; } // do nothing: while (true); } Keyboard.releaseAll(); // enter: Keyboard.write(KEY_RETURN); break; case WINDOWS: // CTRL-ALT-DEL: Keyboard.press(KEY_LEFT_CTRL); Keyboard.press(KEY_LEFT_ALT); Keyboard.press(KEY_DELETE); delay(100); Keyboard.releaseAll(); //ALT-l: delay(2000); Keyboard.press(KEY_LEFT_ALT); Keyboard.press('l'); Keyboard.releaseAll(); break; case UBUNTU: // CTRL-ALT-DEL: Keyboard.press(KEY_LEFT_CTRL); Keyboard.press(KEY_LEFT_ALT); Keyboard.press(KEY_DELETE); delay(1000); Keyboard.releaseAll(); // Enter to confirm logout: Keyboard.write(KEY_RETURN); break; } // do nothing: while (true); }
注意事项代码
在将程序上传到主板之前,请确保将当前使用的正确操作系统分配给平台变量。
当程序运行时,按下按钮会将引脚 2 连接到地面,并且开发板会将注销序列发送到 USB 连接的 PC。
结果
当您将引脚 2 连接到地时,它会执行注销操作。
它使用以下键盘组合来注销 -
在Windows上,按 CTRL-ALT-DEL,然后按 ALT-l
在Ubuntu上,CTRL-ALT-DEL 和 ENTER
在OSX上,CMD-SHIFT-q