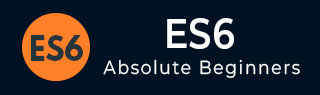
- ES6 教程
- ES6 - 主页
- ES6 - 概述
- ES6 - 环境
- ES6 - 语法
- ES6 - 变量
- ES6 - 运算符
- ES6 - 决策
- ES6 - 循环
- ES6 - 函数
- ES6 - 事件
- ES6 - Cookie
- ES6 - 页面重定向
- ES6 - 对话框
- ES6 - 无效关键字
- ES6 - 页面打印
- ES6 - 对象
- ES6 - 数字
- ES6 - 布尔值
- ES6 - 字符串
- ES6 - 符号
- ES6 - 新的字符串方法
- ES6 - 数组
- ES6 - 日期
- ES6 - 数学
- ES6 - 正则表达式
- ES6 - HTML DOM
- ES6 - 迭代器
- ES6 - 集合
- ES6 - 类
- ES6 - 地图和集合
- ES6 - 承诺
- ES6 - 模块
- ES6 - 错误处理
- ES6 - 对象扩展
- ES6 - 反射 API
- ES6 - 代理 API
- ES6 - 验证
- ES6 - 动画
- ES6 - 多媒体
- ES6 - 调试
- ES6 - 图像映射
- ES6 - 浏览器
- ES7 - 新特性
- ES8 - 新特性
- ES9 - 新特性
- ES6 有用资源
- ES6 - 快速指南
- ES6 - 有用的资源
- ES6 - 讨论
ES7 - 新特性
本章提供有关 ES7 新特性的知识。
求幂运算符
ES7 引入了一种新的数学运算符,称为幂运算符。该运算符类似于使用 Math.pow() 方法。求幂运算符由双星号 ** 表示。该运算符只能与数值一起使用。使用幂运算符的语法如下 -
句法
求幂运算符的语法如下 -
base_value ** exponent_value
例子
以下示例使用Math.pow()方法和指数运算符 计算数字的指数。
<script> let base = 2 let exponent = 3 console.log('using Math.pow()',Math.pow(base,exponent)) console.log('using exponentiation operator',base**exponent) </script>
上述代码片段的输出如下所示 -
using Math.pow() 8 using exponentiation operator 8
数组包含
ES7 中引入的 Array.includes() 方法有助于检查数组中的元素是否可用。在 ES7 之前,Array 类的 indexof() 方法可用于验证数组中是否存在某个值。如果找到数据,indexof() 返回数组中第一次出现的元素的索引,如果数据不存在,则返回 -1。
Array.includes() 方法接受一个参数,检查作为参数传递的值是否存在于数组中。如果找到该值,则此方法返回 true;如果该值不存在,则返回 false。使用 Array.includes() 方法的语法如下 -
句法
Array.includes(value)
或者
Array.includes(value,start_index)
第二种语法检查指定索引中是否存在该值。
例子
以下示例声明一个数组标记并使用 Array.includes() 方法来验证数组中是否存在值。
<script> let marks = [50,60,70,80] //check if 50 is included in array if(marks.includes(50)){ console.log('found element in array') }else{ console.log('could not find element') } // check if 50 is found from index 1 if(marks.includes(50,1)){ //search from index 1 console.log('found element in array') }else{ console.log('could not find element') } //check Not a Number(NaN) in an array console.log([NaN].includes(NaN)) //create an object array let user1 = {name:'kannan'}, user2 = {name:'varun'}, user3={name:'prijin'} let users = [user1,user2] //check object is available in array console.log(users.includes(user1)) console.log(users.includes(user3)) </script>
上述代码的输出如下 -
found element in array could not find element true true false