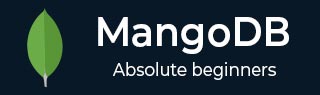
- MongoDB 教程
- MongoDB - 主页
- MongoDB - 概述
- MongoDB - 优点
- MongoDB - 环境
- MongoDB - 数据建模
- MongoDB - 创建数据库
- MongoDB - 删除数据库
- MongoDB - 创建集合
- MongoDB - 删除集合
- MongoDB - 数据类型
- MongoDB - 插入文档
- MongoDB - 查询文档
- MongoDB - 更新文档
- MongoDB - 删除文档
- MongoDB - 投影
- MongoDB - 限制记录
- MongoDB - 记录排序
- MongoDB - 索引
- MongoDB - 聚合
- MongoDB - 复制
- MongoDB - 分片
- MongoDB - 创建备份
- MongoDB - 部署
- MongoDB-Java
- MongoDB-PHP
- 高级 MongoDB
- MongoDB - 关系
- MongoDB - 数据库参考
- MongoDB - 涵盖查询
- MongoDB - 分析查询
- MongoDB - 原子操作
- MongoDB - 高级索引
- MongoDB - 索引限制
- MongoDB - 对象 ID
- MongoDB - 映射减少
- MongoDB - 文本搜索
- MongoDB - 正则表达式
- 与 Rockmongo 合作
- MongoDB-GridFS
- MongoDB - 上限集合
- 自动递增序列
- MongoDB 有用资源
- MongoDB - 问题与解答
- MongoDB - 快速指南
- MongoDB - 有用的资源
- MongoDB - 讨论
MongoDB-PHP
要将 MongoDB 与 PHP 结合使用,您需要使用 MongoDB PHP 驱动程序。从 URL下载 PHP 驱动程序下载驱动程序。请务必下载它的最新版本。现在解压缩存档并将 php_mongo.dll 放入 PHP 扩展目录(默认情况下为“ext”),并将以下行添加到 php.ini 文件中 -
extension = php_mongo.dll
建立连接并选择数据库
要建立连接,您需要指定数据库名称,如果数据库不存在,MongoDB 会自动创建它。
以下是连接到数据库的代码片段 -
<?php // connect to mongodb $m = new MongoClient(); echo "Connection to database successfully"; // select a database $db = $m->mydb; echo "Database mydb selected"; ?>
当程序执行时,将产生以下结果 -
Connection to database successfully Database mydb selected
创建收藏
以下是创建集合的代码片段 -
<?php // connect to mongodb $m = new MongoClient(); echo "Connection to database successfully"; // select a database $db = $m->mydb; echo "Database mydb selected"; $collection = $db->createCollection("mycol"); echo "Collection created succsessfully"; ?>
当程序执行时,将产生以下结果 -
Connection to database successfully Database mydb selected Collection created succsessfully
插入文档
要将文档插入 MongoDB,请使用insert()方法。
以下是插入文档的代码片段 -
<?php // connect to mongodb $m = new MongoClient(); echo "Connection to database successfully"; // select a database $db = $m->mydb; echo "Database mydb selected"; $collection = $db->mycol; echo "Collection selected succsessfully"; $document = array( "title" => "MongoDB", "description" => "database", "likes" => 100, "url" => "http://www.tutorialspoint.com/mongodb/", "by" => "tutorials point" ); $collection->insert($document); echo "Document inserted successfully"; ?>
当程序执行时,将产生以下结果 -
Connection to database successfully Database mydb selected Collection selected succsessfully Document inserted successfully
查找所有文档
要从集合中选择所有文档,请使用 find() 方法。
以下是选择所有文档的代码片段 -
<?php // connect to mongodb $m = new MongoClient(); echo "Connection to database successfully"; // select a database $db = $m->mydb; echo "Database mydb selected"; $collection = $db->mycol; echo "Collection selected succsessfully"; $cursor = $collection->find(); // iterate cursor to display title of documents foreach ($cursor as $document) { echo $document["title"] . "\n"; } ?>
当程序执行时,将产生以下结果 -
Connection to database successfully Database mydb selected Collection selected succsessfully { "title": "MongoDB" }
更新文档
要更新文档,您需要使用 update() 方法。
在下面的示例中,我们将插入文档的标题更新为MongoDB Tutorial。以下是更新文档的代码片段 -
<?php // connect to mongodb $m = new MongoClient(); echo "Connection to database successfully"; // select a database $db = $m->mydb; echo "Database mydb selected"; $collection = $db->mycol; echo "Collection selected succsessfully"; // now update the document $collection->update(array("title"=>"MongoDB"), array('$set'=>array("title"=>"MongoDB Tutorial"))); echo "Document updated successfully"; // now display the updated document $cursor = $collection->find(); // iterate cursor to display title of documents echo "Updated document"; foreach ($cursor as $document) { echo $document["title"] . "\n"; } ?>
当程序执行时,将产生以下结果 -
Connection to database successfully Database mydb selected Collection selected succsessfully Document updated successfully Updated document { "title": "MongoDB Tutorial" }
删除文档
要删除文档,您需要使用remove()方法。
在以下示例中,我们将删除标题为MongoDB Tutorial的文档。以下是删除文档的代码片段 -
<?php // connect to mongodb $m = new MongoClient(); echo "Connection to database successfully"; // select a database $db = $m->mydb; echo "Database mydb selected"; $collection = $db->mycol; echo "Collection selected succsessfully"; // now remove the document $collection->remove(array("title"=>"MongoDB Tutorial"),false); echo "Documents deleted successfully"; // now display the available documents $cursor = $collection->find(); // iterate cursor to display title of documents echo "Updated document"; foreach ($cursor as $document) { echo $document["title"] . "\n"; } ?>
当程序执行时,将产生以下结果 -
Connection to database successfully Database mydb selected Collection selected successfully Documents deleted successfully
在上面的例子中,第二个参数是布尔类型,用于remove()方法的justOne字段。
其余 MongoDB 方法findOne()、save()、limit()、skip()、sort()等的工作原理与上面解释的相同。