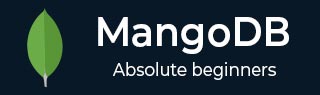
- MongoDB 教程
- MongoDB - 主页
- MongoDB - 概述
- MongoDB - 优点
- MongoDB - 环境
- MongoDB - 数据建模
- MongoDB - 创建数据库
- MongoDB - 删除数据库
- MongoDB - 创建集合
- MongoDB - 删除集合
- MongoDB - 数据类型
- MongoDB - 插入文档
- MongoDB - 查询文档
- MongoDB - 更新文档
- MongoDB - 删除文档
- MongoDB - 投影
- MongoDB - 限制记录
- MongoDB - 记录排序
- MongoDB - 索引
- MongoDB - 聚合
- MongoDB - 复制
- MongoDB - 分片
- MongoDB - 创建备份
- MongoDB - 部署
- MongoDB-Java
- MongoDB-PHP
- 高级 MongoDB
- MongoDB - 关系
- MongoDB - 数据库参考
- MongoDB - 涵盖查询
- MongoDB - 分析查询
- MongoDB - 原子操作
- MongoDB - 高级索引
- MongoDB - 索引限制
- MongoDB - 对象 ID
- MongoDB - 映射减少
- MongoDB - 文本搜索
- MongoDB - 正则表达式
- 与 Rockmongo 合作
- MongoDB-GridFS
- MongoDB - 上限集合
- 自动递增序列
- MongoDB 有用资源
- MongoDB - 问题与解答
- MongoDB - 快速指南
- MongoDB - 有用的资源
- MongoDB - 讨论
MongoDB - 查询文档
在本章中,我们将学习如何从 MongoDB 集合中查询文档。
find() 方法
要从 MongoDB 集合中查询数据,需要使用 MongoDB 的find()方法。
句法
find()方法的基本语法如下 -
>db.COLLECTION_NAME.find()
find()方法将以非结构化方式显示所有文档。
例子
假设我们创建了一个名为 mycol 的集合:
> use sampleDB switched to db sampleDB > db.createCollection("mycol") { "ok" : 1 } >
并使用 insert() 方法在其中插入 3 个文档,如下所示 -
> db.mycol.insert([ { title: "MongoDB Overview", description: "MongoDB is no SQL database", by: "tutorials point", url: "http://www.tutorialspoint.com", tags: ["mongodb", "database", "NoSQL"], likes: 100 }, { title: "NoSQL Database", description: "NoSQL database doesn't have tables", by: "tutorials point", url: "http://www.tutorialspoint.com", tags: ["mongodb", "database", "NoSQL"], likes: 20, comments: [ { user:"user1", message: "My first comment", dateCreated: new Date(2013,11,10,2,35), like: 0 } ] } ])
以下方法检索集合中的所有文档 -
> db.mycol.find() { "_id" : ObjectId("5dd4e2cc0821d3b44607534c"), "title" : "MongoDB Overview", "description" : "MongoDB is no SQL database", "by" : "tutorials point", "url" : "http://www.tutorialspoint.com", "tags" : [ "mongodb", "database", "NoSQL" ], "likes" : 100 } { "_id" : ObjectId("5dd4e2cc0821d3b44607534d"), "title" : "NoSQL Database", "description" : "NoSQL database doesn't have tables", "by" : "tutorials point", "url" : "http://www.tutorialspoint.com", "tags" : [ "mongodb", "database", "NoSQL" ], "likes" : 20, "comments" : [ { "user" : "user1", "message" : "My first comment", "dateCreated" : ISODate("2013-12-09T21:05:00Z"), "like" : 0 } ] } >
Pretty() 方法
要以格式化的方式显示结果,可以使用 Pretty() 方法。
句法
>db.COLLECTION_NAME.find().pretty()
例子
以下示例从名为 mycol 的集合中检索所有文档,并以易于阅读的格式排列它们。
> db.mycol.find().pretty() { "_id" : ObjectId("5dd4e2cc0821d3b44607534c"), "title" : "MongoDB Overview", "description" : "MongoDB is no SQL database", "by" : "tutorials point", "url" : "http://www.tutorialspoint.com", "tags" : [ "mongodb", "database", "NoSQL" ], "likes" : 100 } { "_id" : ObjectId("5dd4e2cc0821d3b44607534d"), "title" : "NoSQL Database", "description" : "NoSQL database doesn't have tables", "by" : "tutorials point", "url" : "http://www.tutorialspoint.com", "tags" : [ "mongodb", "database", "NoSQL" ], "likes" : 20, "comments" : [ { "user" : "user1", "message" : "My first comment", "dateCreated" : ISODate("2013-12-09T21:05:00Z"), "like" : 0 } ] }
findOne() 方法
除了 find() 方法之外,还有findOne()方法,该方法仅返回一个文档。
句法
>db.COLLECTIONNAME.findOne()
例子
以下示例检索标题为 MongoDB Overview 的文档。
> db.mycol.findOne({title: "MongoDB Overview"}) { "_id" : ObjectId("5dd6542170fb13eec3963bf0"), "title" : "MongoDB Overview", "description" : "MongoDB is no SQL database", "by" : "tutorials point", "url" : "http://www.tutorialspoint.com", "tags" : [ "mongodb", "database", "NoSQL" ], "likes" : 100 }
MongoDB 中的 RDBMSWhere 子句等效项
要根据某些条件查询文档,可以使用以下操作。
手术 | 句法 | 例子 | 关系型数据库管理系统等效项 |
---|---|---|---|
平等 | {<键>:{$例如;<值>}} | db.mycol.find({"by":"教程点"}).pretty() | 其中 by = '教程点' |
少于 | {<键>:{$lt:<值>}} | db.mycol.find({"likes":{$lt:50}}).pretty() | 其中喜欢 < 50 |
小于等于 | {<键>:{$lte:<值>}} | db.mycol.find({"likes":{$lte:50}}).pretty() | 其中喜欢 <= 50 |
比...更棒 | {<键>:{$gt:<值>}} | db.mycol.find({"likes":{$gt:50}}).pretty() | 哪里喜欢> 50 |
大于等于 | {<键>:{$gte:<值>}} | db.mycol.find({"likes":{$gte:50}}).pretty() | 其中喜欢 >= 50 |
不等于 | {<键>:{$ne:<值>}} | db.mycol.find({"likes":{$ne:50}}).pretty() | 哪里喜欢!= 50 |
数组中的值 | {<键>:{$in:[<值1>, <值2>,……<值N>]}} | db.mycol.find({"name":{$in:["Raj", "Ram", "Raghu"]}}).pretty() | 其中 name 与 :["Raj", "Ram", "Raghu"] 中的任何值匹配 |
值不在数组中 | {<键>:{$nin:<值>}} | db.mycol.find({"name":{$nin:["Ramu", "Raghav"]}}).pretty() | 其中名称值不在数组中:["Ramu", "Raghav"] 或者根本不存在 |
MongoDB 中的 AND
句法
如果要根据AND条件查询文档,需要使用$and关键字。以下是 AND 的基本语法 -
>db.mycol.find({ $and: [ {<key1>:<value1>}, { <key2>:<value2>} ] })
例子
以下示例将显示“tutorials point”编写的标题为“MongoDB Overview”的所有教程。
> db.mycol.find({$and:[{"by":"tutorials point"},{"title": "MongoDB Overview"}]}).pretty() { "_id" : ObjectId("5dd4e2cc0821d3b44607534c"), "title" : "MongoDB Overview", "description" : "MongoDB is no SQL database", "by" : "tutorials point", "url" : "http://www.tutorialspoint.com", "tags" : [ "mongodb", "database", "NoSQL" ], "likes" : 100 } >
对于上面给出的示例,等效的 where 子句将是' where by = 'tutorials point' AND title = 'MongoDB Overview' '。您可以在 find 子句中传递任意数量的键、值对。
或在 MongoDB 中
句法
如果要根据OR条件查询文档,需要使用$or关键字。以下是OR的基本语法-
>db.mycol.find( { $or: [ {key1: value1}, {key2:value2} ] } ).pretty()
例子
以下示例将显示“tutorials point”编写的或标题为“MongoDB Overview”的所有教程。
>db.mycol.find({$or:[{"by":"tutorials point"},{"title": "MongoDB Overview"}]}).pretty() { "_id": ObjectId(7df78ad8902c), "title": "MongoDB Overview", "description": "MongoDB is no sql database", "by": "tutorials point", "url": "http://www.tutorialspoint.com", "tags": ["mongodb", "database", "NoSQL"], "likes": "100" } >
一起使用 AND 和 OR
例子
以下示例将显示点赞数超过 10 且标题为“MongoDB Overview”或“tutorials point”的文档。等效的 SQL where 子句为'where likes>10 AND (by = '教程点' OR title = 'MongoDB 概述')'
>db.mycol.find({"likes": {$gt:10}, $or: [{"by": "tutorials point"}, {"title": "MongoDB Overview"}]}).pretty() { "_id": ObjectId(7df78ad8902c), "title": "MongoDB Overview", "description": "MongoDB is no sql database", "by": "tutorials point", "url": "http://www.tutorialspoint.com", "tags": ["mongodb", "database", "NoSQL"], "likes": "100" } >
MongoDB 中的 NOR
句法
要根据NOT条件查询文档,需要使用$not关键字。以下是NOT的基本语法-
>db.COLLECTION_NAME.find( { $not: [ {key1: value1}, {key2:value2} ] } )
例子
假设我们在empDetails集合中插入了 3 个文档,如下所示 -
db.empDetails.insertMany( [ { First_Name: "Radhika", Last_Name: "Sharma", Age: "26", e_mail: "radhika_sharma.123@gmail.com", phone: "9000012345" }, { First_Name: "Rachel", Last_Name: "Christopher", Age: "27", e_mail: "Rachel_Christopher.123@gmail.com", phone: "9000054321" }, { First_Name: "Fathima", Last_Name: "Sheik", Age: "24", e_mail: "Fathima_Sheik.123@gmail.com", phone: "9000054321" } ] )
以下示例将检索名字不是“Radhika”且姓氏不是“Christopher”的文档
> db.empDetails.find( { $nor:[ 40 {"First_Name": "Radhika"}, {"Last_Name": "Christopher"} ] } ).pretty() { "_id" : ObjectId("5dd631f270fb13eec3963bef"), "First_Name" : "Fathima", "Last_Name" : "Sheik", "Age" : "24", "e_mail" : "Fathima_Sheik.123@gmail.com", "phone" : "9000054321" }
不在 MongoDB 中
句法
要基于 NOT 条件查询文档,您需要使用 $not 关键字,以下是NOT的基本语法-
>db.COLLECTION_NAME.find( { $NOT: [ {key1: value1}, {key2:value2} ] } ).pretty()
例子
以下示例将检索年龄不大于 25 的文档
> db.empDetails.find( { "Age": { $not: { $gt: "25" } } } ) { "_id" : ObjectId("5dd6636870fb13eec3963bf7"), "First_Name" : "Fathima", "Last_Name" : "Sheik", "Age" : "24", "e_mail" : "Fathima_Sheik.123@gmail.com", "phone" : "9000054321" }