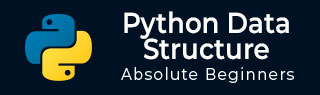
- Python 数据结构和算法教程
- Python-DS 主页
- Python-DS简介
- Python-DS 环境
- Python-数组
- Python - 列表
- Python - 元组
- Python-字典
- Python - 二维数组
- Python-矩阵
- Python - 集合
- Python - 地图
- Python - 链表
- Python-堆栈
- Python-队列
- Python-出队
- Python - 高级链表
- Python-哈希表
- Python - 二叉树
- Python - 搜索树
- Python - 堆
- Python - 图表
- Python - 算法设计
- Python——分而治之
- Python - 递归
- Python-回溯
- Python - 排序算法
- Python - 搜索算法
- Python - 图算法
- Python-算法分析
- Python - 大 O 表示法
- Python - 算法类
- Python - 摊销分析
- Python - 算法论证
- Python 数据结构和算法有用资源
- Python - 快速指南
- Python - 有用的资源
- Python - 讨论
Python - 高级链表
我们已经在前面的章节中看到了链表,其中只能向前移动。在本章中,我们将看到另一种类型的链表,其中可以向前和向后移动。这样的链表称为双向链表。以下是双向链表的特点。
双向链表包含一个称为第一个和最后一个的链接元素。
每个链接都带有一个数据字段和两个称为下一个和上一个的链接字段。
每个链接都使用其下一个链接与其下一个链接链接。
每个链接都使用其先前的链接与其先前的链接链接。
最后一个链接带有一个空链接来标记列表的结尾。
创建双向链表
我们使用 Node 类创建一个双向链表。现在我们使用与单链表中使用的相同的方法,但头指针和下一个指针将用于正确分配,以除了节点中存在的数据之外在每个节点中创建两个链接。
例子
class Node: def __init__(self, data): self.data = data self.next = None self.prev = None class doubly_linked_list: def __init__(self): self.head = None # Adding data elements def push(self, NewVal): NewNode = Node(NewVal) NewNode.next = self.head if self.head is not None: self.head.prev = NewNode self.head = NewNode # Print the Doubly Linked list def listprint(self, node): while (node is not None): print(node.data), last = node node = node.next dllist = doubly_linked_list() dllist.push(12) dllist.push(8) dllist.push(62) dllist.listprint(dllist.head)
输出
执行上述代码时,会产生以下结果 -
62 8 12
插入双向链表
在这里,我们将了解如何使用以下程序将节点插入到双向链表中。该程序使用名为 insert 的方法,该方法将新节点插入到双向链表头部的第三个位置。
例子
# Create the Node class class Node: def __init__(self, data): self.data = data self.next = None self.prev = None # Create the doubly linked list class doubly_linked_list: def __init__(self): self.head = None # Define the push method to add elements def push(self, NewVal): NewNode = Node(NewVal) NewNode.next = self.head if self.head is not None: self.head.prev = NewNode self.head = NewNode # Define the insert method to insert the element def insert(self, prev_node, NewVal): if prev_node is None: return NewNode = Node(NewVal) NewNode.next = prev_node.next prev_node.next = NewNode NewNode.prev = prev_node if NewNode.next is not None: NewNode.next.prev = NewNode # Define the method to print the linked list def listprint(self, node): while (node is not None): print(node.data), last = node node = node.next dllist = doubly_linked_list() dllist.push(12) dllist.push(8) dllist.push(62) dllist.insert(dllist.head.next, 13) dllist.listprint(dllist.head)
输出
执行上述代码时,会产生以下结果 -
62 8 13 12
追加到双向链表
追加到双向链表将在末尾添加元素。
例子
# Create the node class class Node: def __init__(self, data): self.data = data self.next = None self.prev = None # Create the doubly linked list class class doubly_linked_list: def __init__(self): self.head = None # Define the push method to add elements at the begining def push(self, NewVal): NewNode = Node(NewVal) NewNode.next = self.head if self.head is not None: self.head.prev = NewNode self.head = NewNode # Define the append method to add elements at the end def append(self, NewVal): NewNode = Node(NewVal) NewNode.next = None if self.head is None: NewNode.prev = None self.head = NewNode return last = self.head while (last.next is not None): last = last.next last.next = NewNode NewNode.prev = last return # Define the method to print def listprint(self, node): while (node is not None): print(node.data), last = node node = node.next dllist = doubly_linked_list() dllist.push(12) dllist.append(9) dllist.push(8) dllist.push(62) dllist.append(45) dllist.listprint(dllist.head)
输出
执行上述代码时,会产生以下结果 -
62 8 12 9 45
请注意追加操作中元素 9 和 45 的位置。