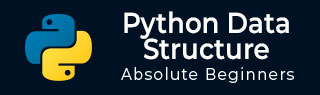
- Python 数据结构和算法教程
- Python-DS 主页
- Python-DS简介
- Python-DS 环境
- Python-数组
- Python - 列表
- Python - 元组
- Python-字典
- Python - 二维数组
- Python-矩阵
- Python - 集合
- Python - 地图
- Python - 链表
- Python-堆栈
- Python-队列
- Python-出队
- Python - 高级链表
- Python-哈希表
- Python - 二叉树
- Python - 搜索树
- Python - 堆
- Python - 图表
- Python - 算法设计
- Python——分而治之
- Python - 递归
- Python-回溯
- Python - 排序算法
- Python - 搜索算法
- Python - 图算法
- Python-算法分析
- Python - 大 O 表示法
- Python - 算法类
- Python - 摊销分析
- Python - 算法论证
- Python 数据结构和算法有用资源
- Python - 快速指南
- Python - 有用的资源
- Python - 讨论
Python-字典
在 Dictionary 中,每个键与其值之间用冒号 (:) 分隔,项目之间用逗号分隔,整个内容用大括号括起来。没有任何项目的空字典仅用两个大括号编写,如下所示 - {}。
键在字典中是唯一的,而值可能不是。字典的值可以是任何类型,但键必须是不可变的数据类型,例如字符串、数字或元组。
访问字典中的值
要访问字典元素,您可以使用熟悉的方括号和键来获取其值。
例子
一个简单的例子如下 -
#!/usr/bin/python dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'} print ("dict['Name']: ", dict['Name']) print ("dict['Age']: ", dict['Age'])
输出
执行上述代码时,会产生以下结果 -
dict['Name']: Zara dict['Age']: 7
如果我们尝试使用不属于字典的键访问数据项,我们会收到如下错误 -
例子
#!/usr/bin/python dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'} print ("dict['Alice']: ", dict['Alice'])
输出
执行上述代码时,会产生以下结果 -
dict['Alice']: Traceback (most recent call last): File "test.py", line 4, in <module> print "dict['Alice']: ", dict['Alice']; KeyError: 'Alice'
更新词典
您可以通过添加新条目或键值对、修改现有条目或删除现有条目来更新字典,如下面的简单示例所示 -
例子
#!/usr/bin/python dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'} dict['Age'] = 8; # update existing entry dict['School'] = "DPS School"; # Add new entry print ("dict['Age']: ", dict['Age']) print ("dict['School']: ", dict['School'])
输出
执行上述代码时,会产生以下结果 -
dict['Age']: 8 dict['School']: DPS School
删除字典元素
您可以删除单个字典元素或清除字典的全部内容。您还可以通过一次操作删除整个字典。
例子
要显式删除整个字典,只需使用del语句。一个简单的例子如下 -
#!/usr/bin/python dict = {'Name': 'Zara', 'Age': 7, 'Class': 'First'} del dict['Name']; # remove entry with key 'Name' dict.clear(); # remove all entries in dict del dict ; # delete entire dictionary print ("dict['Age']: ", dict['Age']) print ("dict['School']: ", dict['School'])
注意- 会引发异常,因为del dict字典不再存在 -
输出
这会产生以下结果 -
dict['Age']: dict['Age'] dict['School']: dict['School']
注意- del() 方法将在后续部分中讨论。
字典键的属性
字典值没有限制。它们可以是任意 Python 对象,可以是标准对象,也可以是用户定义的对象。然而,对于按键来说却并非如此。
关于字典键有两点需要记住 -
每个键不允许有多个条目。这意味着不允许有重复的密钥。当分配过程中遇到重复键时,最后一次分配获胜。
例如
#!/usr/bin/python dict = {'Name': 'Zara', 'Age': 7, 'Name': 'Manni'} print ("dict['Name']: ", dict['Name'])
输出
执行上述代码时,会产生以下结果 -
dict['Name']: Manni
键必须是不可变的。这意味着您可以使用字符串、数字或元组作为字典键,但不允许使用 ['key'] 之类的内容。
例子
一个例子如下 -
#!/usr/bin/python dict = {['Name']: 'Zara', 'Age': 7} print ("dict['Name']: ", dict['Name'])
输出
执行上述代码时,会产生以下结果 -
Traceback (most recent call last): File "test.py", line 3, in <module> dict = {['Name']: 'Zara', 'Age': 7}; TypeError: list objects are unhashable