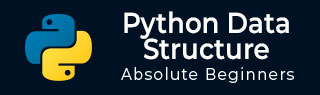
- Python 数据结构和算法教程
- Python-DS 主页
- Python-DS简介
- Python-DS 环境
- Python-数组
- Python - 列表
- Python - 元组
- Python-字典
- Python - 二维数组
- Python-矩阵
- Python - 集合
- Python - 地图
- Python - 链表
- Python-堆栈
- Python-队列
- Python-出队
- Python - 高级链表
- Python-哈希表
- Python - 二叉树
- Python - 搜索树
- Python - 堆
- Python - 图表
- Python - 算法设计
- Python——分而治之
- Python - 递归
- Python-回溯
- Python - 排序算法
- Python - 搜索算法
- Python - 图算法
- Python-算法分析
- Python - 大 O 表示法
- Python - 算法类
- Python - 摊销分析
- Python - 算法论证
- Python 数据结构和算法有用资源
- Python - 快速指南
- Python - 有用的资源
- Python - 讨论
Python-队列
在日常生活中,我们在等待服务时很熟悉排队。队列数据结构也意味着数据元素排列在队列中的情况相同。队列的独特之处在于添加和删除项目的方式。这些物品可以放在一端,但从另一端移走。所以这是一种先进先出的方法。
队列可以使用 python list 来实现,我们可以使用 insert() 和 pop() 方法来添加和删除元素。它们不是插入,因为数据元素总是添加到队列的末尾。
添加元素
在下面的示例中,我们创建一个队列类,在其中实现先进先出方法。我们使用内置的插入方法来添加数据元素。
例子
class Queue: def __init__(self): self.queue = list() def addtoq(self,dataval): # Insert method to add element if dataval not in self.queue: self.queue.insert(0,dataval) return True return False def size(self): return len(self.queue) TheQueue = Queue() TheQueue.addtoq("Mon") TheQueue.addtoq("Tue") TheQueue.addtoq("Wed") print(TheQueue.size())
输出
执行上述代码时,会产生以下结果 -
3
删除元素
在下面的示例中,我们创建一个队列类,在其中插入数据,然后使用内置的 pop 方法删除数据。
例子
class Queue: def __init__(self): self.queue = list() def addtoq(self,dataval): # Insert method to add element if dataval not in self.queue: self.queue.insert(0,dataval) return True return False # Pop method to remove element def removefromq(self): if len(self.queue)>0: return self.queue.pop() return ("No elements in Queue!") TheQueue = Queue() TheQueue.addtoq("Mon") TheQueue.addtoq("Tue") TheQueue.addtoq("Wed") print(TheQueue.removefromq()) print(TheQueue.removefromq())
输出
执行上述代码时,会产生以下结果 -
Mon Tue