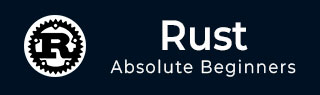
- Rust 教程
- 铁锈 - 主页
- Rust - 简介
- Rust - 环境设置
- Rust - HelloWorld 示例
- Rust - 数据类型
- Rust - 变量
- 铁锈 - 恒定
- Rust - 字符串
- Rust - 运算符
- Rust - 决策
- 铁锈 - 循环
- Rust - 函数
- Rust - 元组
- Rust - 数组
- Rust - 所有权
- Rust - 借用
- 铁锈 - 切片
- Rust - 结构
- Rust - 枚举
- Rust - 模块
- Rust - 收藏
- Rust - 错误处理
- Rust - 泛型类型
- Rust - 输入输出
- Rust - 文件输入/输出
- Rust - 包管理器
- Rust - 迭代器和闭包
- Rust - 智能指针
- Rust - 并发
- Rust 有用的资源
- Rust - 快速指南
- Rust - 有用的资源
- Rust - 讨论
Rust - 泛型类型
泛型是为不同类型的多个上下文编写代码的工具。在 Rust 中,泛型是指数据类型和特征的参数化。泛型允许通过减少代码重复和提供类型安全来编写更简洁和干净的代码。泛型的概念可以应用于方法、函数、结构、枚举、集合和特征。
<T> 语法称为类型参数,用于声明泛型构造。T代表任何数据类型。
插图:通用集合
以下示例声明一个只能存储整数的向量。
fn main(){ let mut vector_integer: Vec<i32> = vec![20,30]; vector_integer.push(40); println!("{:?}",vector_integer); }
输出
[20, 30, 40]
考虑以下片段 -
fn main() { let mut vector_integer: Vec<i32> = vec![20,30]; vector_integer.push(40); vector_integer.push("hello"); //error[E0308]: mismatched types println!("{:?}",vector_integer); }
上面的例子表明,整数类型的向量只能存储整数值。因此,如果我们尝试将字符串值推入集合中,编译器将返回错误。泛型使集合更加类型安全。
插图:通用结构
type参数代表一个类型,编译器稍后会填充该类型。
struct Data<T> { value:T, } fn main() { //generic type of i32 let t:Data<i32> = Data{value:350}; println!("value is :{} ",t.value); //generic type of String let t2:Data<String> = Data{value:"Tom".to_string()}; println!("value is :{} ",t2.value); }
上面的示例声明了一个名为Data的通用结构。<T>类型表示某种数据类型。main ()函数创建该结构的两个实例——一个整数实例和一个字符串实例。
输出
value is :350 value is :Tom
性状
特征可用于跨多个结构实现一组标准Behave(方法)。特征就像面向对象编程中的接口。Trait 的语法如下所示 -
声明一个特质
trait some_trait { //abstract or method which is empty fn method1(&self); // this is already implemented , this is free fn method2(&self){ //some contents of method2 } }
特征可以包含具体方法(带主体的方法)或抽象方法(不带主体的方法)。如果方法定义将由实现该特征的所有结构共享,则使用具体方法。但是,结构可以选择覆盖由特征定义的函数。
如果方法定义因实现结构而异,则使用抽象方法。
语法 - 实现特征
impl some_trait for structure_name { // implement method1() there.. fn method1(&self ){ } }
以下示例使用方法print()定义了一个特征Printable,该方法由结构book实现。
fn main(){ //create an instance of the structure let b1 = Book { id:1001, name:"Rust in Action" }; b1.print(); } //declare a structure struct Book { name:&'static str, id:u32 } //declare a trait trait Printable { fn print(&self); } //implement the trait impl Printable for Book { fn print(&self){ println!("Printing book with id:{} and name {}",self.id,self.name) } }
输出
Printing book with id:1001 and name Rust in Action
通用函数
该示例定义了一个通用函数,该函数显示传递给它的参数。该参数可以是任何类型。参数的类型应该实现 Display 特征,以便它的值可以通过 println! 打印出来。宏。
use std::fmt::Display; fn main(){ print_pro(10 as u8); print_pro(20 as u16); print_pro("Hello TutorialsPoint"); } fn print_pro<T:Display>(t:T){ println!("Inside print_pro generic function:"); println!("{}",t); }
输出
Inside print_pro generic function: 10 Inside print_pro generic function: 20 Inside print_pro generic function: Hello TutorialsPoint