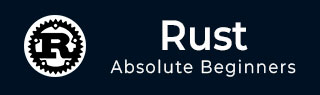
- Rust 教程
- 铁锈 - 主页
- Rust - 简介
- Rust - 环境设置
- Rust - HelloWorld 示例
- Rust - 数据类型
- Rust - 变量
- 铁锈 - 恒定
- Rust - 字符串
- Rust - 运算符
- Rust - 决策
- 铁锈 - 循环
- Rust - 函数
- Rust - 元组
- Rust - 数组
- Rust - 所有权
- Rust - 借用
- 铁锈 - 切片
- Rust - 结构
- Rust - 枚举
- Rust - 模块
- Rust - 收藏
- Rust - 错误处理
- Rust - 泛型类型
- Rust - 输入输出
- Rust - 文件输入/输出
- Rust - 包管理器
- Rust - 迭代器和闭包
- Rust - 智能指针
- Rust - 并发
- Rust 有用的资源
- Rust - 快速指南
- Rust - 有用的资源
- Rust - 讨论
Rust - 结构
数组用于表示同构值的集合。类似地,结构是 Rust 中可用的另一种用户定义的数据类型,它允许我们组合不同类型的数据项,包括另一种结构。结构将数据定义为键值对。
语法 - 声明结构
struct关键字用于声明结构。由于结构是静态类型的,因此结构中的每个字段都必须与数据类型相关联。结构体的命名规则和约定类似于变量的命名规则和约定。结构块必须以分号结尾。
struct Name_of_structure { field1:data_type, field2:data_type, field3:data_type }
语法 - 初始化结构
声明结构后,应为每个字段分配一个值。这称为初始化。
let instance_name = Name_of_structure { field1:value1, field2:value2, field3:value3 }; //NOTE the semicolon Syntax: Accessing values in a structure Use the dot notation to access value of a specific field. instance_name.field1 Illustration struct Employee { name:String, company:String, age:u32 } fn main() { let emp1 = Employee { company:String::from("TutorialsPoint"), name:String::from("Mohtashim"), age:50 }; println!("Name is :{} company is {} age is {}",emp1.name,emp1.company,emp1.age); }
上面的示例声明了一个具有三个字段的 Employee 结构体:姓名、公司和年龄类型。main() 初始化该结构。它使用 println! 用于打印结构中定义的字段值的宏。
输出
Name is :Mohtashim company is TutorialsPoint age is 50
修改结构体实例
要修改实例,实例变量应标记为可变的。下面的示例声明并初始化一个名为Employee的结构,然后将age字段的值从 50 修改为 40。
let mut emp1 = Employee { company:String::from("TutorialsPoint"), name:String::from("Mohtashim"), age:50 }; emp1.age = 40; println!("Name is :{} company is {} age is {}",emp1.name,emp1.company,emp1.age);
输出
Name is :Mohtashim company is TutorialsPoint age is 40
将结构传递给函数
以下示例显示如何将结构体实例作为参数传递。display 方法将 Employee 实例作为参数并打印详细信息。
fn display( emp:Employee) { println!("Name is :{} company is {} age is {}",emp.name,emp.company,emp.age); }
这是完整的程序 -
//declare a structure struct Employee { name:String, company:String, age:u32 } fn main() { //initialize a structure let emp1 = Employee { company:String::from("TutorialsPoint"), name:String::from("Mohtashim"), age:50 }; let emp2 = Employee{ company:String::from("TutorialsPoint"), name:String::from("Kannan"), age:32 }; //pass emp1 and emp2 to display() display(emp1); display(emp2); } // fetch values of specific structure fields using the // operator and print it to the console fn display( emp:Employee){ println!("Name is :{} company is {} age is {}",emp.name,emp.company,emp.age); }
输出
Name is :Mohtashim company is TutorialsPoint age is 50 Name is :Kannan company is TutorialsPoint age is 32
从函数返回结构体
让我们考虑一个函数who_is_elder(),它比较两个员工的年龄并返回年长的那个。
fn who_is_elder (emp1:Employee,emp2:Employee)->Employee { if emp1.age>emp2.age { return emp1; } else { return emp2; } }
这是完整的程序 -
fn main() { //initialize structure let emp1 = Employee{ company:String::from("TutorialsPoint"), name:String::from("Mohtashim"), age:50 }; let emp2 = Employee { company:String::from("TutorialsPoint"), name:String::from("Kannan"), age:32 }; let elder = who_is_elder(emp1,emp2); println!("elder is:"); //prints details of the elder employee display(elder); } //accepts instances of employee structure and compares their age fn who_is_elder (emp1:Employee,emp2:Employee)->Employee { if emp1.age>emp2.age { return emp1; } else { return emp2; } } //display name, comapny and age of the employee fn display( emp:Employee) { println!("Name is :{} company is {} age is {}",emp.name,emp.company,emp.age); } //declare a structure struct Employee { name:String, company:String, age:u32 }
输出
elder is: Name is :Mohtashim company is TutorialsPoint age is 50
结构中的方法
方法就像函数。它们是一组逻辑编程指令。方法是用fn关键字声明的。方法的范围在结构块内。
方法在结构块之外声明。impl关键字用于在结构上下文中定义方法。方法的第一个参数始终是self,它表示结构的调用实例。方法对结构的数据成员进行操作。
要调用方法,我们需要首先实例化结构。可以使用结构的实例来调用该方法。
句法
struct My_struct {} impl My_struct { //set the method's context fn method_name() { //define a method } }
插图
以下示例定义了一个结构体Rectangle,其字段为width和height。方法区是在结构的上下文中定义的。area 方法通过self关键字访问结构体的字段并计算矩形的面积。
//define dimensions of a rectangle struct Rectangle { width:u32, height:u32 } //logic to calculate area of a rectangle impl Rectangle { fn area(&self)->u32 { //use the . operator to fetch the value of a field via the self keyword self.width * self.height } } fn main() { // instanatiate the structure let small = Rectangle { width:10, height:20 }; //print the rectangle's area println!("width is {} height is {} area of Rectangle is {}",small.width,small.height,small.area()); }
输出
width is 10 height is 20 area of Rectangle is 200
结构中的静态方法
静态方法可以用作实用方法。这些方法甚至在结构实例化之前就存在。静态方法是使用结构名称调用的,并且无需实例即可访问。与普通方法不同,静态方法不会采用&self参数。
语法 - 声明静态方法
类似函数和其他方法的静态方法可以选择包含参数。
impl Structure_Name { //static method that creates objects of the Point structure fn method_name(param1: datatype, param2: datatype) -> return_type { // logic goes here } }
语法 - 调用静态方法
Structure_name ::语法用于访问静态方法。
structure_name::method_name(v1,v2)
插图
以下示例使用getInstance方法作为工厂类,用于创建并返回结构Point的实例。
//declare a structure struct Point { x: i32, y: i32, } impl Point { //static method that creates objects of the Point structure fn getInstance(x: i32, y: i32) -> Point { Point { x: x, y: y } } //display values of the structure's field fn display(&self){ println!("x ={} y={}",self.x,self.y ); } } fn main(){ // Invoke the static method let p1 = Point::getInstance(10,20); p1.display(); }
输出
x =10 y=20