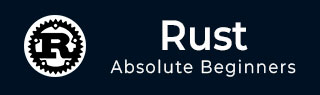
- Rust 教程
- 铁锈 - 主页
- Rust - 简介
- Rust - 环境设置
- Rust - HelloWorld 示例
- Rust - 数据类型
- Rust - 变量
- 铁锈 - 恒定
- Rust - 字符串
- Rust - 运算符
- Rust - 决策
- 铁锈 - 循环
- Rust - 函数
- Rust - 元组
- Rust - 数组
- Rust - 所有权
- Rust - 借用
- 铁锈 - 切片
- Rust - 结构
- Rust - 枚举
- Rust - 模块
- Rust - 收藏
- Rust - 错误处理
- Rust - 泛型类型
- Rust - 输入输出
- Rust - 文件输入/输出
- Rust - 包管理器
- Rust - 迭代器和闭包
- Rust - 智能指针
- Rust - 并发
- Rust 有用的资源
- Rust - 快速指南
- Rust - 有用的资源
- Rust - 讨论
铁锈 - 切片
切片是指向内存块的指针。切片可用于访问存储在连续内存块中的部分数据。它可以与数组、向量和字符串等数据结构一起使用。切片使用索引号来访问部分数据。切片的大小在运行时确定。
切片是指向实际数据的指针。它们通过函数引用传递,这也称为借用。
例如,切片可用于获取字符串值的一部分。切片字符串是指向实际字符串对象的指针。因此,我们需要指定字符串的起始和结束索引。索引和数组一样从0开始。
句法
let sliced_value = &data_structure[start_index..end_index]
最小索引值为0,最大索引值为数据结构的大小。请注意,end_index 将不会包含在最终字符串中。
下图显示了一个示例字符串Tutorials,它有 9 个字符。第一个字符的索引为 0,最后一个字符的索引为 8。

以下代码从字符串中获取 5 个字符(从索引 4 开始)。
fn main() { let n1 = "Tutorials".to_string(); println!("length of string is {}",n1.len()); let c1 = &n1[4..9]; // fetches characters at 4,5,6,7, and 8 indexes println!("{}",c1); }
输出
length of string is 9 rials
插图 - 切片整数数组
main() 函数声明一个包含 5 个元素的数组。它调用use_slice()函数并向其传递一个由三个元素组成的切片(指向数据数组)。切片通过引用传递。use_slice() 函数打印切片的值及其长度。
fn main(){ let data = [10,20,30,40,50]; use_slice(&data[1..4]); //this is effectively borrowing elements for a while } fn use_slice(slice:&[i32]) { // is taking a slice or borrowing a part of an array of i32s println!("length of slice is {:?}",slice.len()); println!("{:?}",slice); }
输出
length of slice is 3 [20, 30, 40]
可变切片
&mut关键字可用于将切片标记为可变。
fn main(){ let mut data = [10,20,30,40,50]; use_slice(&mut data[1..4]); // passes references of 20, 30 and 40 println!("{:?}",data); } fn use_slice(slice:&mut [i32]) { println!("length of slice is {:?}",slice.len()); println!("{:?}",slice); slice[0] = 1010; // replaces 20 with 1010 }
输出
length of slice is 3 [20, 30, 40] [10, 1010, 30, 40, 50]
上面的代码将可变切片传递给use_slice()函数。该函数修改原始数组的第二个元素。