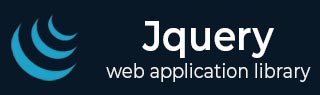
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 添加元素
jQuery 提供了各种方法在现有 HTML 文档中添加新的 DOM 元素。您可以根据您的要求在不同位置添加这些新元素(在任何现有标签之前或之后)。
jQuery的append()方法
jQuery的append()方法将内容添加到匹配的每个元素的末尾。您还可以在单个函数调用中附加多个元素。
以下是append()方法的语法:
$(selector).append(content, [content]);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
概要
考虑以下 HTML 内容:
<div class="container"> <h2>jQuery append() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div>
现在,如果我们应用append()方法,如下所示:
$( ".inner" ).append( "<p>Zara</p>" );
它将产生以下结果:
<div class="container"> <h2>jQuery append() Method</h2> <div class="inner">Hello <p>Zara</p> </div> <div class="inner">Goodbye <p>Zara</p> </div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $(".inner").append("<p>Zara</p>"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery append() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery的appendTo()方法
jQuery的appendTo()方法执行与appendTo()相同的任务。主要区别在于具体的语法、内容和目标的位置。
以下是appendTo()方法的语法:
$(content).appendTo(selector);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("<p>Zara</p>").appendTo(".inner"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery appendTo() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery after() 方法
jQuery after()方法在匹配的每个元素之后添加内容。您还可以在单个函数调用中插入多个元素。
以下是after()方法的语法:
$(selector).after(content, [content]);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
概要
考虑以下 HTML 内容:
<div class="container"> <h2>jQuery after() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div>
现在,如果我们应用after()方法,如下所示:
$( ".inner" ).after( "<p>Zara</p>" );
它将产生以下结果:
<div class="container"> <h2>jQuery after() Method</h2> <div class="inner">Hello</div> <p>Zara</p> <div class="inner">Goodbye</div> <p>Zara</p> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $(".inner").after("<p>Zara</p>"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery after() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery insertAfter() 方法
jQuery insertAfter()方法在匹配的每个元素之后添加内容。after ()和insertAfter()方法执行相同的任务。主要区别在于具体的语法、内容和目标的位置。
以下是after()方法的语法:
$(content).insertAfter(selector);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("<p>Zara</p>").insertAfter(".inner"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery insertAfter() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery prepend() 方法
jQuery prepend()方法将内容添加到匹配的每个元素的开头。您还可以在单个函数调用中添加多个元素。
以下是append()方法的语法:
$(selector).prepend(content, [content]);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
概要
考虑以下 HTML 内容:
<div class="container"> <h2>jQuery prepend() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div>
现在,如果我们应用prepend()方法,如下所示:
$( ".inner" ).prepend( "<p>Zara</p>" );
它将产生以下结果:
<div class="container"> <h2>jQuery prepend() Method</h2> <div class="inner"> <p>Zara</p> Hello </div> <div class="inner"> <p>Zara</p> Goodbye </div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $(".inner").prepend("<p>Zara</p>"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery prepend() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery prependTo() 方法
jQuery prependTo()方法执行与prepend()相同的任务。主要区别在于具体的语法、内容和目标的位置。
以下是prependTo()方法的语法:
$(content).prependTo(selector);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("<p>Zara</p>").prependTo(".inner"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery prependTo() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery before() 方法
jQuery before()方法在匹配的每个元素之前添加内容。您还可以在单个函数调用中插入多个元素。
以下是before()方法的语法:
$(selector).before(content, [content]);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
概要
考虑以下 HTML 内容:
<div class="container"> <h2>jQuery before() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div>
现在,如果我们应用before()方法,如下所示:
$( ".inner" ).before( "<p>Zara</p>" );
它将产生以下结果:
<div class="container"> <h2>jQuery before() Method</h2> <p>Zara</p> <div class="inner">Hello</div> <p>Zara</p> <div class="inner">Goodbye</div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $(".inner").before("<p>Zara</p>"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery before() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery insertBefore() 方法
jQuery insertBefore()方法在匹配的每个元素之前添加内容。before ()和insertBefore()方法执行相同的任务。主要区别在于具体的语法、内容和目标的位置。
以下是after()方法的语法:
$(content).insertBefore(selector);
这里的内容参数可以是一个 HTML 字符串、一个 DOM 元素、文本节点、元素和文本节点数组或插入到匹配元素集中每个元素末尾的 jQuery 对象。
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("<p>Zara</p>").insertBefore(".inner"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery insertBefore() Method</h2> <div class="inner">Hello</div> <div class="inner">Goodbye</div> </div> <br> <button>Add Text</button> </body> </html>
jQuery HTML/CSS 参考
您可以在以下页面获取操作 CSS 和 HTML 内容的所有 jQuery 方法的完整参考:jQuery HTML/CSS 参考。