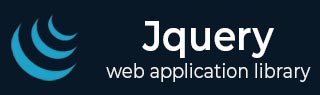
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 删除元素
jQuery 提供了remove()和empty()方法来从HTML 文档中删除现有的HTML 元素。
jQuery的remove()方法
jQuery的remove()方法从文档中删除选定的元素及其子元素。
以下是remove()方法的语法:
$(selector).remove();
当您想要删除元素本身及其内部的所有内容时,应该使用remove()方法。
概要
考虑以下 HTML 内容:
<div class="container"> <h2>jQuery remove() Method</h2> <div class="hello">Hello</div> <div class="goodbye">Goodbye</div> </div>
现在,如果我们按如下方式应用remove()方法:
$( ".hello" ).remove();
它将产生以下结果:
<div class="container"> <h2>jQuery remove() Method</h2> <div class="goodbye">Goodbye</div> </div>
如果 <div class="hello"> 内有任意数量的嵌套元素,它们也会被删除。
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".hello" ).remove(); }); }); </script> </head> <body> <div class="container"> <h2>jQuery remove() Method</h2> <div class="hello">Hello</div> <div class="goodbye">Goodbye</div> </div> <br> <button>Remove Text</button> </body> </html>
用过滤器去除
我们还可以包含一个选择器作为remove()方法的可选参数。例如,我们可以将之前的 DOM 删除代码重写如下:
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("div").remove(".hello"); }); }); </script> </head> <body> <div class="container"> <h2>jQuery remove(selector) Method</h2> <div class="hello">Hello</div> <div class="goodbye">Goodbye</div> </div> <br> <button>Remove Text</button> </body> </html>
jQueryempty() 方法
jQuery的empty()方法与remove()非常相似,它从文档中删除选定的元素及其子元素。此方法不接受任何参数。
以下是empty()方法的语法:
$(selector).empty();
当您想要删除元素本身及其内部的所有内容时,应该使用empty()方法。
概要
考虑以下 HTML 内容:
<div class="container"> <h2>jQuery empty() Method</h2> <div class="hello">Hello</div> <div class="goodbye">Goodbye</div> </div>
现在,如果我们应用empty()方法,如下所示:
$( ".hello" ).empty();
它将产生以下结果:
<div class="container"> <h2>jQuery empty() Method</h2> <div class="goodbye">Goodbye</div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".hello" ).empty(); }); }); </script> </head> <body> <div class="container"> <h2>jQuery empty() Method</h2> <div class="hello">Hello</div> <div class="goodbye">Goodbye</div> </div> <br> <button>Remove Text</button> </body> </html>
jQuery HTML/CSS 参考
您可以在以下页面获取操作 CSS 和 HTML 内容的所有 jQuery 方法的完整参考:jQuery HTML/CSS 参考。