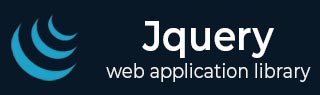
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 链接
在我们讨论jQuery方法链接之前,请考虑一下您想要对 HTML 元素执行以下操作的情况:
- 1 - 选择一个段落元素。
- 2 - 更改段落的颜色。
- 3 - 对段落应用淡出效果。
- 4 - 对段落应用淡入效果。
以下是执行上述操作的 jQuery 程序:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("p").css("color", "#fb7c7c"); $("p").fadeOut(1000); $("p").fadeIn(1000); }); }); </script> <style> button{width:100px;cursor:pointer;} </style> </head> <body> <p>Click the below button to see the result:</p> <button>Click Me</button> </body> </html>
jQuery 方法链接
jQuery 方法链接允许我们在同一元素上使用单个语句来调用多个 jQuery 方法。这提供了更好的性能,因为在使用链接时,我们不需要每次解析整个页面来查找元素。
要链接不同的方法,我们只需将该方法附加到前一个方法即可。例如我们上面的程序可以写成如下:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("p").css("color", "#fb7c7c").fadeOut(1000).fadeIn(1000); }); }); </script> <style> button{width:100px;cursor:pointer;} </style> </head> <body> <p>Click the below button to see the result:</p> <button>Click Me</button> </body> </html>
链接动画
我们在编写 jQuery 动画程序时可以利用 jQuery 方法链接。以下是一个借助链接编写的简单动画程序:
<html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("div").animate({left: '250px'}) .animate({top: '100px'}) .animate({left: '0px'}) .animate({top: '0px'}); }); }); </script> <style> button {width:125px;cursor:pointer} #box{position:relative;margin:3px;padding:10px;height:20px; width:100px;background-color:#9c9cff;} </style> </head> <body> <p>Click on Start Animation button to see the result:</p> <div id="box">This is Box</div> <button>Start Animation</button> </body> </html>