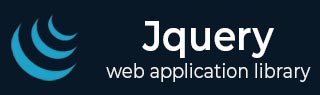
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 选择器
jQuery最重要的功能是由它的Selectors提供的。本教程将通过涵盖所有三个标准选择器的简单示例来解释 jQuery 选择器。
jQuery 选择器
jQuery 选择器用于从 HTML 文档中选择 HTML 元素。假设给定了一个 HTML 文档,您需要从该文档中选择所有 <div>。这就是 jQuery 选择器可以提供帮助的地方。
jQuery 选择器可以根据以下内容查找 HTML 元素(即选择 HTML 元素):
HTML 元素名称
元素编号
元素类
元素属性名称
元素属性值
更多标准
jQuery 库利用级联样式表(CSS) 选择器的强大功能,让我们能够快速轻松地访问文档对象模型 (DOM) 中的元素或元素组。
jQuery 选择器在 HTML 文档上的工作方式非常相似,就像SQL Select 语句在数据库上选择记录一样。
jQuery 选择器语法
以下是用于选择 HTML 元素的 jQuery 选择器语法:
$(document).ready(function(){ $(selector) });
jQuery 选择器以美元符号$开头,然后我们将选择器放在大括号()内。这里$()被称为工厂函数,它在选择给定文档中的元素时使用以下三个构建块:
选择器名称 | 描述 |
---|---|
元素选择器 | 表示 DOM 中可用的 HTML 元素名称。例如$('p')选择文档中的所有段落 <p>。 |
#id选择器 | 表示 DOM 中具有给定 ID 的可用 HTML 元素。例如$('#some-id')选择文档中以some-id作为元素 Id 的单个元素。 |
.class选择器 | 表示 DOM 中给定类可用的 HTML 元素。例如$('.some-class')选择文档中具有some-class类的所有元素。 |
上述所有选择器都可以单独使用,也可以与其他选择器结合使用。除了一些调整之外,所有 jQuery 选择器都基于相同的原理。
元素选择器
jQuery元素选择器根据元素名称选择 HTML 元素。以下是元素选择器的简单语法:
$(document).ready(function(){ $("Html Element Name") });
请注意,在使用元素名称作为 jQuery 选择器时,我们不会随元素一起提供尖括号。例如,我们只给出普通的p而不是<p>。
例子
以下是从 HTML 文档中选择所有<p>元素,然后更改这些段落的背景颜色的示例。您不会在此示例生成的输出中看到任何<p>元素。您还可以更改代码以使用不同的元素名称作为选择器,然后单击图标来验证结果。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("p").css("background-color", "yellow"); }); </script> </head> <body> <h1>jQuery element Selector</h1> <p>This is p tag</p> <span>This is span tag</span> <div>This is div tag</div> </body> </html>
#id 选择器
jQuery #id选择器根据元素id属性选择 HTML 元素。以下是#id选择器的简单语法:
$(document).ready(function(){ $("#id of the element") });
要使用 jQuery #id选择器,您需要确保id属性应唯一分配给所有 DOM 元素。如果你的元素有相似的 id 那么它不会产生正确的结果。
例子
以下是选择id为foo的<p>元素并更改这些段落的背景颜色的示例。您还可以更改代码以使用不同的元素 id 属性作为选择器,然后单击图标来验证结果。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#foo").css("background-color", "yellow"); }); </script> </head> <body> <h1>jQuery #id Selector</h1> <p id="foo">This is foo p tag</p> <span id="bar">This is bar span tag</span> <div id="bill">This is bill div tag</div> </body> </html>
.class 选择器
jQuery .class选择器根据元素类属性选择 HTML 元素。以下是.class选择器的简单语法:
$(document).ready(function(){ $(".class of the element") });
由于一个类可以在 HTML 文档中分配给多个 HTML 元素,因此很有可能使用单个.class选择器语句找到多个元素。
例子
以下是选择所有类为foo 的元素并更改这些元素的背景颜色的示例。您还可以更改代码以使用不同的元素类名称作为选择器,然后单击图标来验证结果。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $(".foo").css("background-color", "yellow"); }); </script> </head> <body> <h1>jQuery .class Selector</h1> <p class="foo">This is foo p tag</p> <p class="foo">This is one more foo p tag</p> <span class="bar">This is bar span tag</span> <div class="bill">This is bill div tag</div> </body> </html>
到目前为止,我们只介绍了三个标准 jQuery 选择器。有关所有这些 jQuery 选择器的完整详细信息,您可以访问jQuery 选择器参考。