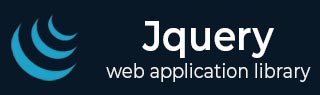
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 属性操作
jQuery 被大量用于操作与 HTML 元素相关的各种属性。每个 HTML 元素都可以有各种标准和自定义属性(即属性),用于定义该 HTML 元素的特征。
jQuery 为我们提供了轻松操作(获取和设置)元素属性的方法。首先让我们尝试了解一些有关 HTML 标准和自定义属性的知识。
标准属性
一些更常见的属性是 -
- 班级名称
- 标签名
- ID
- 链接地址
- 标题
- 相对
- 源代码
- 风格
例子
让我们看一下以下图像元素 HTML 标记的代码片段 -
<img id="id" src="image.gif" alt="Image" class="class" title="This is an image"/>
在此元素的标记中,标记名称为 img,id、src、alt、class 和 title 标记表示元素的属性,每个属性都由名称和值组成。
自定义数据-* 属性
HTML 规范允许我们向 DOM 元素添加我们自己的自定义属性,以提供有关该元素的其他详细信息。这些属性名称以data-开头。
例子
下面是一个示例,其中我们使用自定义属性data-copyright提供有关图像版权的信息-
<img data-copyright="Tutorials Point" id="imageid" src="image.gif" alt="Image"/>
jQuery - 获取标准属性
jQuery attr()方法用于从匹配的 HTML 元素中获取任何标准属性的值。我们将使用jQuery 选择器来匹配所需的元素,然后应用attr()方法来获取元素的属性值。
如果给定的选择器匹配多个元素,那么它会返回一个值列表,您可以使用 jQuery 数组方法对其进行迭代。
例子
以下是一个获取锚 <a> 元素的 href 和 title 属性的 jQuery 程序:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ alert( "Href = " + $("#home").attr("href")); alert( "Title = " + $("#home").attr("title")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <p><a id="home" href="index.htm" title="Tutorials Point">Home</a></p> <button>Get Attribute</button> </body> </html>
jQuery - 获取数据属性
jQuery data()方法用于从匹配的 HTML 元素中获取任何自定义数据属性的值。我们将使用jQuery 选择器来匹配所需的元素,然后应用data()方法来获取元素的属性值。
例子
以下是一个获取 <div> 元素的作者姓名和年份属性的 jQuery 程序:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ alert( "Author = " + $("#home").data("author-name")); alert( "Year = " + $("#home").data("year")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <div id="home" data-author-name="Zara Ali" data-year="2022"> Just an Example Content </div> <br> <button>Get Attribute</button> </body> </html>
jQuery - 设置标准属性
jQuery attr(name, value)方法用于设置匹配的 HTML 元素的任何标准属性的值。我们将使用jQuery 选择器来匹配所需的元素,然后应用attr(key, value)方法来设置元素的属性值。
如果给定的选择器匹配多个元素,那么它将为所有匹配的元素设置属性值。
例子
以下是一个用于设置锚点 <a> 元素的 title 属性的 jQuery 程序:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("#home").attr("title", "New Anchor Title"); /* Let's get and display changed title */ alert( "Changed Title = " + $("#home").attr("title")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <p><a id="home" href="index.htm" title="Tutorials Point">Home</a></p> <button>Set Attribute</button> <p>You can hover the Home link to verify the title before and after the change.</p> </body> </html>
jQuery - 设置自定义属性
jQuery data(name, value)方法用于设置匹配的 HTML 元素的任何自定义属性的值。我们将使用jQuery 选择器来匹配所需的元素,然后应用attr(key, value)方法来设置元素的属性值。
如果给定的选择器匹配多个元素,那么它将为所有匹配的元素设置属性值。
例子
以下是一个用于设置 <div> 元素的author-name 属性的 jQuery 程序:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("#home").data("author-name", "Nuha Ali"); /* Let's get and display changed author name */ alert( "Changed Name = " + $("#home").data("author-name")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <div id="home" data-author-name="Zara Ali" data-year="2022"> Just an Example Content </div> <br> <button>Set Attribute</button> </body> </html>
jQuery HTML/CSS 参考
您可以在以下页面获取操作 HTML 和 CSS 内容的所有 jQuery 方法的完整参考:jQuery HTML/CSS 参考。