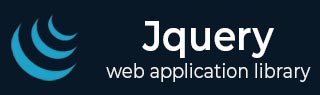
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 动画
让我们学习如何使用 jQuery animate()方法在网页或任何其他 jQuery (Javascript) 应用程序上创建自定义动画。
jQuery animate() 方法
jQuery animate()方法用于通过更改 DOM 元素的 CSS 数字属性(例如宽度、高度、边距、填充、不透明度、顶部、左侧等)来创建自定义动画。
以下是animate()方法的简单语法:
$(selector).animate({ properties }, [speed, callback] );
jQuery animate() 方法不能用于对非数字属性(例如颜色或背景颜色等)进行动画处理。尽管您可以使用 jQuery 插件jQuery.Color对此类属性进行动画处理。
您可以应用任何 jQuery选择器来选择任何 DOM 元素,然后应用 jQuery animate()方法对其进行动画处理。以下是所有参数的描述,使您可以完全控制动画 -
properties - 定义要动画的 CSS 属性的必需参数,这是调用的唯一强制参数。
speed - 一个可选字符串,表示三个预定义速度(“慢”、“正常”或“快”)之一或运行动画的毫秒数(例如 1000)。
回调- 一个可选参数,表示动画完成时要执行的函数。
动画先决条件
(a) - animate()方法不会使隐藏元素作为效果的一部分可见。例如,给定 $(selector).hide().animate({height: "20px"}, 500),动画将运行,但元素将保持隐藏状态。
(b) - 要操纵 DOM 元素的位置作为动画的一部分,首先我们需要将其位置设置为relative、fixed或absolute,因为默认情况下,所有 HTML 元素都具有静态位置,并且无法移动使用animate()方法。
例子
以下示例演示如何使用animate()方法将 <div> 元素向右移动,直到其 left 属性达到 250px。接下来,当我们单击左按钮时,相同的 <div> 元素返回到其初始位置。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#right").click(function(){ $("div").animate({left: '250px'}); }); $("#left").click(function(){ $("div").animate({left: '0px'}); }); }); </script> <style> #left, #right{margin:3px;border:2px solid #666; height:30px; width:100px;cursor:pointer;} #box{position:relative;margin:3px;padding:12px;border:2px solid #666; height:100px; width:180px;} </style> </head> <body> <p>Click on Left or Right button to see the result:</p> <div id="box" style="background-color:#9c9cff;">This is Box</div> <button id="right" style="background-color:#fb7c7c;">Right Move</button> <button id="left" style="background-color:#93ff93;">Left Move</button> </body> </html>
具有自定义速度的动画
我们可以以不同的速度对 DOM 元素的不同 CSS 数值属性(例如,宽度、高度或左侧)进行动画处理。
例子
让我们重写上面的示例,其中我们将使用 1000 毫秒的速度参数为 <div> 的向右移动设置动画,并使用 5000 毫秒的速度参数设置向左移动动画。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#right").click(function(){ $("div").animate({left: '250px'}, 1000); }); $("#left").click(function(){ $("div").animate({left: '0px'}, 5000); }); }); </script> <style> #left, #right{margin:3px;border:2px solid #666; height:30px; width:100px;cursor:pointer;} #box{position:relative;margin:3px;padding:12px;border:2px solid #666; height:100px; width:180px;} </style> </head> <body> <p>Click on Left or Right button to see the result:</p> <div id="box" style="background-color:#9c9cff;">This is Box</div> <button id="right" style="background-color:#fb7c7c;">Right Move</button> <button id="left" style="background-color:#93ff93;">Left Move</button> </body> </html>
具有预定义值的动画
我们可以使用字符串'show'、'hide'和'toggle'作为 CSS 数字属性的值。
例子
以下是一个示例,其中我们借助两个按钮将元素的left属性设置为隐藏或显示。
请注意,使用这些值设置任何数字 CSS 属性都会产生相同的结果。例如,如果您将元素的宽度或高度设置为隐藏,那么无论您设置元素的宽度属性还是高度属性,它都会隐藏该元素。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#right").click(function(){ $("div").animate({left: 'hide'}); }); $("#left").click(function(){ $("div").animate({left: 'show'}); }); }); </script> <style> #left, #right{margin:3px;border:2px solid #666; height:30px; width:100px;cursor:pointer;} #box{position:relative;margin:3px;padding:12px;border:2px solid #666; height:100px; width:180px;} </style> </head> <body> <p>Click on Left or Right button to see the result:</p> <div id="box" style="background-color:#9c9cff;">This is Box</div> <button id="right" style="background-color:#fb7c7c;">Right Move</button> <button id="left" style="background-color:#93ff93;">Left Move</button> </body> </html>
具有多个属性的动画
jQuery animate()允许我们同时对一个元素的多个 CSS 属性进行动画处理。
例子
以下是为 <div> 元素的多个 CSS 属性设置动画的示例。当我们点击Right Move按钮时,这个 <div> 开始向右移动,直到它的 left 属性值达到 250px,同时元素的不透明度减少到 0.2,盒子的宽度和高度减少到 100px。接下来,当我们单击“向左移动”按钮时,该框将返回到其初始位置和大小。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src = "https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#right").click(function(){ $("div").animate({left: '250px', width:'100px', height:'100px', opacity:0.2}); }); $("#left").click(function(){ $("div").animate({left: '0px',width:'180px', height:'100px', opacity:1.0}); }); }); </script> <style> #left, #right{margin:3px;border:2px solid #666; height:30px; width:100px;cursor:pointer;} #box{position:relative;margin:3px;padding:12px;border:2px solid #666; height:100px; width:180px;} </style> </head> <body> <p>Click on Left or Right button to see the result:</p> <div id="box" style="background-color:#9c9cff;">This is Box</div> <button id="right" style="background-color:#fb7c7c;">Right Move</button> <button id="left" style="background-color:#93ff93;">Left Move</button> </body> </html>
具有队列功能的动画
考虑一种情况,您需要应用多个动画,这意味着您需要多次调用animate()方法。在这种情况下,jQuery 通过先进先出 (FIFO) 队列处理这些动画请求,并允许根据您的创造力创建有趣的动画。
例子
下面是一个例子,我们调用animate()方法 4 次,将 <div> 逐个移动到多个方向。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("div").animate({left: '250px'}); $("div").animate({top: '100px'}); $("div").animate({left: '0px'}); $("div").animate({top: '0px'}); }); }); </script> <style> button {margin:3px;border:2px solid #666; height:30px; width:180px;cursor:pointer;} #box{position:relative;margin:3px;padding:2px;border:2px solid #666; height:30px; width:170px;} </style> </head> <body> <p>Click on Start Animation button to see the result:</p> <div id="box" style="background-color:#9c9cff;">This is Box</div> <button style="background-color:#93ff93;">Start Animation</button> </body> </html>
jQuery 效果参考
您可以在以下页面获取所有 jQuery 效果方法的完整参考:jQuery 效果参考。