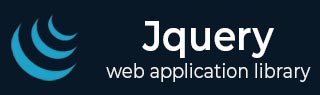
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - 遍历祖先
jQuery 提供了在 DOM 树内部向上遍历以查找给定元素的祖先的方法。这些方法可用于查找 DOM 内给定元素的父元素、祖父母、曾祖父母等。
在DOM树内部向上遍历有以下三种方法:
Parent() - 返回每个匹配元素的直接父元素。
parents() - 返回匹配元素的所有祖先元素。
parentUntil() - 返回所有祖先元素,直到找到作为选择器参数给出的元素。
jQuery的parent()方法
jQuery Parent()方法返回每个匹配元素的直接父元素。以下是该方法的简单语法:
$(selector).parent([filter])
我们可以选择在方法中提供一个过滤器选择器。如果提供了过滤器,将通过测试元素是否匹配来过滤元素。
概要
考虑以下 HTML 内容:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
现在,如果我们使用parent()方法,如下所示:
$( ".child-two" ).parent().css( "border", "2px solid red" );
它将产生以下结果:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent" style="border:2px solid red"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".child-two" ).parent().css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div class="great-grand-parent"> <div style="width:500px;" class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div> <br> <button>Mark Parent</button> </body> </html>
您可以通过创建另一个具有相同类的父元素和子元素块来尝试相同的示例,然后验证parent()是否会将给定的CSS应用于所有匹配的元素。
jQueryparents() 方法
jQueryparents ()方法返回匹配元素的所有祖先元素。以下是该方法的简单语法:
$(selector).parents([filter])
我们可以选择在方法中提供一个过滤器选择器。如果提供了过滤器,将通过测试元素是否匹配来过滤元素。
parent ()和parent()方法类似,只是parent()方法仅在DOM 树中向上移动一级。此外, $( "html" ).parent() 方法返回一个包含文档的集合,而 $( "html" ).parents() 返回一个空集合。
概要
考虑以下 HTML 内容:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
现在,如果我们使用parents()方法,如下所示:
$( ".child-two" ).parents().css( "border", "2px solid red" );
它将产生以下结果:
<div class="great-grand-parent" style="border:2px solid red"> <div class="grand-parent" style="border:2px solid red"> <ul class="parent" style="border:2px solid red"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
例子
让我们尝试下面的示例并验证结果。为了清楚起见,这里我们将仅过滤 <div> 元素:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".child-two" ).parents("div").css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div style="width:525px;" class="great-grand-parent"> <div style="width:500px;" class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div> <br> <button>Mark Parents</button> </body> </html>
jQueryparentUntil() 方法
jQueryparentUntil ()方法返回两个选择器之间可用的所有祖先元素。以下是该方法的简单语法:
$(selector1).parentsUntil([selector2][,filter])
我们可以选择在方法中提供一个过滤器选择器。如果提供了过滤器,将通过测试元素是否匹配来过滤元素。
概要
考虑以下 HTML 内容:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
现在,如果我们使用parentUntil()方法,如下所示:
$( ".child-two" ).parentsUntil(".great-grand-parent").css( "border", "2px solid red" );
它将产生以下结果:
<div class="great-grand-parent"> <div class="grand-parent" style="border:2px solid red"> <ul class="parent" style="border:2px solid red"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
例子
让我们尝试以下示例并验证结果:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".child-two" ).parentsUntil(".great-grand-parent").css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent, .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div style="width:525px;" class="great-grand-parent"> <div style="width:500px;" class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div> <br> <button>Mark Parents</button> </body> </html>
jQuery 遍历参考
您可以在以下页面获取遍历 DOM 的所有 jQuery 方法的完整参考:jQuery 遍历参考。