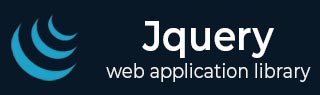
- jQuery 教程
- jQuery - 主页
- jQuery - 概述
- jQuery - 基础知识
- jQuery - 语法
- jQuery - 选择器
- jQuery - 事件
- jQuery - 属性
- jQuery-AJAX
- jQuery DOM 操作
- jQuery-DOM
- jQuery - 添加元素
- jQuery - 删除元素
- jQuery - 替换元素
- jQuery CSS 操作
- jQuery - CSS 类
- jQuery - 尺寸
- jQuery - CSS 属性
- jQuery 效果
- jQuery - 效果
- jQuery - 动画
- jQuery - 链接
- jQuery - 回调函数
- jQuery 遍历
- jQuery - 遍历
- jQuery - 遍历祖先
- jQuery - 遍历后代
- jQuery用户界面
- jQuery - 交互
- jQuery - 小部件
- jQuery - 主题
- jQuery 参考
- jQuery - 实用程序
- jQuery 插件
- jQuery - 插件
- jQuery - PagePiling.js
- jQuery-Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery 有用资源
- jQuery - 问题与解答
- jQuery - 快速指南
- jQuery - 有用的资源
- jQuery - 讨论
jQuery - DOM 操作
jQuery 提供了许多有效地操作 DOM 的方法。您不需要编写庞大而复杂的代码来设置或获取任何 HTML 元素的内容。
jQuery DOM 操作
jQuery 提供了attr()、html()、text()和val()等方法,它们充当 getter 和 setter 来操作 HTML 文档的内容。
文档对象模型 (DOM) - 是一种 W3C(万维网联盟)标准,允许我们创建、更改或删除 HTML 或 XML 文档中的元素。
以下是您可以借助 jQuery 标准库方法对 DOM 元素执行的一些基本操作 -
提取元素的内容
更改元素的内容
在现有元素下添加子元素
在现有元素上方添加父元素
在现有元素之前或之后添加元素
将现有元素替换为另一个元素
删除现有元素
将内容包装在元素内
我们在讨论jQuery 属性时已经介绍了attr()方法,剩下的 DOM 内容操作方法html()、text()和val()将在本章中讨论。
jQuery - 获取内容
jQuery 提供了html()和text()方法来提取匹配的 HTML 元素的内容。以下是这两个方法的语法:
$(selector).html(); $(selector).text();
jQuery text()方法返回内容的纯文本值,而html()返回带有 HTML 标签的内容。您将需要使用 jQuery 选择器来选择目标元素。
例子
以下示例展示了如何使用 jQuery text()和html()方法获取内容:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#text").click(function(){ alert($("p").text()); }); $("#html").click(function(){ alert($("p").html()); }); }); </script> </head> <body> <p>The quick <b>brown fox</b> jumps over the <b>lazy dog</b></p> <button id="text">Get Text</button> <button id="html">Get HTML</button> </body> </html>
获取表单字段
jQuery val()方法用于从任何表单字段获取值。以下是该方法的简单语法。
$(selector).val();
例子
以下是另一个示例,展示如何使用 jQuery 方法val()获取输入字段的值-
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#b1").click(function(){ alert($("#name").val()); }); $("#b2").click(function(){ alert($("#class").val()); }); }); </script> </head> <body> <p>Name: <input type="text" id="name" value="Zara Ali"/></p> <p>Class: <input type="text" id="class" value="Class 12th"/></p> <button id="b1">Get Name</button> <button id="b2">Get Class</button> </body> </html>
jQuery - 设置内容
jQuery html()和text()方法可用于设置匹配的 HTML 元素的内容。以下是这两个方法用于设置值时的语法:
$(selector).html(val, [function]); $(selector).text(val, [function]);
这里val是要为元素设置的文本内容的 HTML。我们可以为这些方法提供一个可选的回调函数,当设置元素的值时将调用该函数。
jQuery text()方法设置内容的纯文本值,而html()方法设置带有 HTML 标签的内容。
例子
以下示例展示了如何使用 jQuery text()和html()方法设置元素的内容:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#text").click(function(){ $("p").text("The quick <b>brown fox</b> jumps over the <b>lazy dog</b>"); }); $("#html").click(function(){ $("p").html("The quick <b>brown fox</b> jumps over the <b>lazy dog</b>"); }); }); </script> </head> <body> <p>Click on any of the two buttons to see the result</p> <button id="text">Set Text</button> <button id="html">Set HTML</button> </body> </html>
设置表单字段
jQuery val()方法还用于设置任何表单字段的值。以下是该方法用于设置值时的简单语法。
$(selector).val(val);
这里val是要为输入字段设置的值。我们可以提供一个可选的回调函数,当设置字段的值时将调用该函数。
例子
以下是另一个示例,展示如何使用 jQuery 方法val()设置输入字段的值-
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#b1").click(function(){ $("#name").val("Zara Ali"); }); $("#b2").click(function(){ $("#class").val("Class 12th"); }); }); </script> </head> <body> <p>Name: <input type="text" id="name" value=""/></p> <p>Class: <input type="text" id="class" value=""/></p> <button id="b1">Set Name</button> <button id="b2">Set Class</button> </body> </html>
jQuery - 替换元素
jQuery的replaceWith()方法可用于将完整的DOM元素替换为另一个HTML或DOM元素。以下是该方法的语法:
$(selector).replaceWith(val);
这里val是你想要的而不是原始元素。这可以是 HTML 或简单文本。
例子
以下是一个示例,其中我们将 <p> 元素替换为 <h1> 元素,然后进一步将 <h1> 元素替换为 <h2> 元素。
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#b1").click(function(){ $("p").replaceWith("<h1>This is new heading</h1>"); }); $("#b2").click(function(){ $("h1").replaceWith("<h2>This is another heading</h2>"); }); }); </script> </head> <body> <p>Click below button to replace me</p> <button id="b1">Replace Paragraph</button> <button id="b2">Replace Heading</button> </body> </html>
jQuery HTML/CSS 参考
您可以在以下页面获取操作 CSS 和 HTML 内容的所有 jQuery 方法的完整参考:jQuery CSS/HTML 参考。