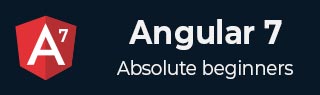
- Angular7 教程
- Angular7 - 主页
- Angular7 - 概述
- Angular7 - 环境设置
- Angular7 - 项目设置
- Angular7 - 组件
- Angular7 - 模块
- Angular7 - 数据绑定
- Angular7 - 事件绑定
- Angular7 - 模板
- Angular7 - 指令
- Angular7 - 管道
- Angular7 - 路由
- Angular7 - 服务
- Angular7 - Http 客户端
- Angular7 - CLI 提示
- Angular7 - 表单
- 材料/CDK-虚拟滚动
- Angular7 - 材质/CDK - 拖放
- Angular7 - 动画
- Angular7 - 材料
- 测试和构建 Angular7 项目
- Angular7 有用资源
- Angular7 - 快速指南
- Angular7 - 有用的资源
- Angular7 - 讨论
Angular7 - 动画
动画在 html 元素之间添加了大量交互。Angular 2 提供了动画功能,从 Angular 4 开始,动画不再是 @angular/core 库的一部分,而是一个单独的包,需要在 app.module.ts 中导入。
首先,我们需要使用以下代码行导入库 -
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
BrowserAnimationsModule需要添加到app.module.ts中的导入数组中,如下所示 -
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule , RoutingComponent} from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { ChangeTextDirective } from './change-text.directive'; import { SqrtPipe } from './app.sqrt'; import { MyserviceService } from './myservice.service'; import { HttpClientModule } from '@angular/common/http'; import { ScrollDispatchModule } from '@angular/cdk/scrolling'; import { DragDropModule } from '@angular/cdk/drag-drop'; import { ReactiveFormsModule } from '@angular/forms'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; @NgModule({ declarations: [ SqrtPipe, AppComponent, NewCmpComponent, ChangeTextDirective, RoutingComponent ], imports: [ BrowserModule, AppRoutingModule, HttpClientModule, ScrollDispatchModule, DragDropModule, ReactiveFormsModule, BrowserAnimationsModule ], providers: [MyserviceService], bootstrap: [AppComponent] }) export class AppModule { }
在app.component.html中,我们添加了要进行动画处理的 html 元素。
<div> <button (click) = "animate()">Click Me</button> <div [@myanimation] = "state" class = "rotate"> <img src = "assets/images/img.png" width = "100" height = "100"> </div> </div>
对于主 div,我们添加了一个按钮和一个带有图像的 div。有一个单击事件,将调用 animate 函数。对于 div,添加了 @myanimation 指令并给出状态值。
现在让我们看看定义动画的app.component.ts 。
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators} from '@angular/forms'; import { trigger, state, style, transition, animate } from '@angular/animations'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], styles:[` div { margin: 0 auto; text-align: center; width:200px; } .rotate { width:100px; height:100px; border:solid 1px red; } `], animations: [ trigger('myanimation',[ state('smaller',style({ transform : 'translateY(100px)' })), state('larger',style({ transform : 'translateY(0px)' })), transition('smaller <=> larger',animate('300ms ease-in')) ]) ] }) export class AppComponent { state: string = "smaller"; animate() { this.state= this.state == 'larger' ? 'smaller' : 'larger'; } }
我们必须导入要在 .ts 文件中使用的动画函数,如上所示。
import { trigger, state, style, transition, animate } from '@angular/animations';
在这里,我们从 @angular/animations 导入了触发器、状态、样式、过渡和动画。
现在,我们将动画属性添加到 @Component () 装饰器 -
animations: [ trigger('myanimation',[ state('smaller',style({ transform : 'translateY(100px)' })), state('larger',style({ transform : 'translateY(0px)' })), transition('smaller <=> larger',animate('300ms ease-in')) ]) ]
触发器定义动画的开始。它的第一个参数是动画的名称,该名称将赋予需要应用动画的 html 标签。第二个参数是我们导入的函数 - 状态、转换等。
状态函数涉及动画步骤,元素将在这些动画步骤之间进行转换。现在我们已经定义了两个状态,较小的和较大的。对于较小的状态,我们给出了样式translate:translateY(100px)和translate:translateY(100px)。
转换函数向 html 元素添加动画。第一个参数接受开始和结束状态,第二个参数接受 animate 函数。animate 函数允许您定义过渡的长度、延迟和难易程度。
现在让我们查看 .html 文件以了解转换函数是如何工作的 -
<div> <button (click) = "animate()">Click Me</button> <div [@myanimation] = "state" class = "rotate"> <img src = "assets/images/img.png" width = "100" height = "100"> </div> </div>
@component 指令中添加了一个 style 属性,它使 div 居中对齐。让我们考虑以下示例来理解相同的内容 -
styles:[` div{ margin: 0 auto; text-align: center; width:200px; } .rotate{ width:100px; height:100px; border:solid 1px red; } `],
这里,特殊字符 [``] 用于向 html 元素添加样式(如果有)。对于 div,我们给出了app.component.ts文件中定义的动画名称。
单击按钮时,它会调用 animate 函数,该函数在app.component.ts文件中定义如下 -
export class AppComponent { state: string = "smaller"; animate() { this.state = this.state == ‘larger’? 'smaller' : 'larger'; } }
状态变量被定义并被赋予较小的默认值。动画函数会在单击时更改状态。如果状态较大,则转换为较小;如果更小,它将转换为更大。
浏览器(http://localhost:4200/)中的输出如下所示:

单击“Click Me”按钮后,图像的位置将发生变化,如下图所示 -

变换函数应用于 y 方向,当我们单击 Click Me 按钮时,y 方向从 0 变为 100px。图像存储在asset/images文件夹中。