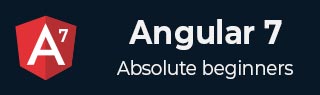
- Angular7 教程
- Angular7 - 主页
- Angular7 - 概述
- Angular7 - 环境设置
- Angular7 - 项目设置
- Angular7 - 组件
- Angular7 - 模块
- Angular7 - 数据绑定
- Angular7 - 事件绑定
- Angular7 - 模板
- Angular7 - 指令
- Angular7 - 管道
- Angular7 - 路由
- Angular7 - 服务
- Angular7 - Http 客户端
- Angular7 - CLI 提示
- Angular7 - 表单
- 材料/CDK-虚拟滚动
- Angular7 - 材质/CDK - 拖放
- Angular7 - 动画
- Angular7 - 材料
- 测试和构建 Angular7 项目
- Angular7 有用资源
- Angular7 - 快速指南
- Angular7 - 有用的资源
- Angular7 - 讨论
Angular7 - 事件绑定
在本章中,我们将讨论事件绑定在 Angular 7 中的工作原理。当用户以键盘移动、鼠标单击或鼠标悬停的形式与应用程序交互时,它会生成一个事件。需要处理这些事件才能执行某种操作。这就是事件绑定发挥作用的地方。
让我们考虑一个例子来更好地理解这一点。
应用程序组件.html
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1>Welcome to {{title}}.</h1> </div> <div> Months : <select> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid</ng-template> <ng-template #condition2>Condition is invalid</ng-template> </div> <button (click) = "myClickFunction($event)"> Click Me </button>
在app.component.html文件中,我们定义了一个按钮并使用单击事件向其添加了一个函数。
以下是定义按钮并向其添加功能的语法。
(click) = "myClickFunction($event)"
该函数定义在:app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // declared array of months. months = ["January", "February", "March", "April", "May","June", "July", "August", "September", "October", "November", "December"]; isavailable = true; //variable is set to true myClickFunction(event) { //just added console.log which will display the event details in browser on click of the button. alert("Button is clicked"); console.log(event); } }
单击按钮后,控件将转到函数myClickFunction并出现一个对话框,其中显示按钮被单击,如下图所示 -

按钮的样式添加在 add.component.css 中 -
button { background-color: #2B3BCF; border: none; color: white; padding: 10px 10px; text-align: center; text-decoration: none; display: inline-block; font-size: 20px; }
现在让我们将 onchange 事件添加到下拉列表中。
以下代码行将帮助您将更改事件添加到下拉列表中 -
应用程序组件.html
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1>Welcome to {{title}}.</h1> </div> <div> Months : <select (change) = "changemonths($event)"> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid</ng-template> <ng-template #condition2>Condition is invalid</ng-template> </div> <br/> <button (click) = "myClickFunction($event)"> Click Me </button>
该函数在app.component.ts文件中声明-
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // declared array of months. months = ["January", "Feburary", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; //variable is set to true myClickFunction(event) { //just added console.log which will display the event details in browser on click of the button. alert("Button is clicked"); console.log(event); } changemonths(event) { console.log("Changed month from the Dropdown"); console.log(event); } }
从下拉列表中选择月份,您会看到控制台消息“已从下拉列表中更改月份”与事件一起显示在控制台中。

当下拉列表中的值发生更改时,让我们在app.component.ts中添加一条警报消息,如下所示 -
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // declared array of months. months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; //variable is set to true myClickFunction(event) { //just added console.log which will display the event details in browser on click of the button. alert("Button is clicked"); console.log(event); } changemonths(event) { alert("Changed month from the Dropdown"); } }
当下拉列表中的值更改时,将出现一个对话框并显示以下消息 -
“从下拉菜单更改月份”。
