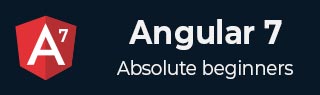
- Angular7 教程
- Angular7 - 主页
- Angular7 - 概述
- Angular7 - 环境设置
- Angular7 - 项目设置
- Angular7 - 组件
- Angular7 - 模块
- Angular7 - 数据绑定
- Angular7 - 事件绑定
- Angular7 - 模板
- Angular7 - 指令
- Angular7 - 管道
- Angular7 - 路由
- Angular7 - 服务
- Angular7 - Http 客户端
- Angular7 - CLI 提示
- Angular7 - 表单
- 材料/CDK-虚拟滚动
- Angular7 - 材质/CDK - 拖放
- Angular7 - 动画
- Angular7 - 材料
- 测试和构建 Angular7 项目
- Angular7 有用资源
- Angular7 - 快速指南
- Angular7 - 有用的资源
- Angular7 - 讨论
Angular7 - 模板
Angular 7 使用 <ng-template> 作为标签,而不是 Angular2 中使用的 <template>。<ng-template> 自 Angular 4 发布以来一直在使用,早期版本(即 Angular 2)也使用 <template> 来实现相同的目的。从 Angular 4 开始使用 <ng-template> 而不是 <template> 的原因是 <template> 标签和 html <template> 标准标签之间存在名称冲突。它将完全弃用。这是 Angular 4 版本中所做的主要更改之一。
现在让我们将模板与if else 条件一起使用并查看输出。
应用程序组件.html
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1>Welcome to {{title}}.</h1> </div> <div> Months : <select (change) = "changemonths($event)" name = "month"> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable;then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid from template</ng-template> <ng-template #condition2>Condition is invalid from template</ng-template> </div> <button (click) = "myClickFunction($event)">Click Me</button>
对于 Span 标记,我们添加了带有else条件的if语句,并将调用模板条件 1 和 else 条件 2。
模板的调用方式如下 -
<ng-template #condition1>Condition is valid from template</ng-template> <ng-template #condition2>Condition is invalid from template</ng-template>
如果条件为真,则调用条件 1模板,否则调用条件 2。
应用程序组件.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // declared array of months. months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = false; // variable is set to true myClickFunction(event) { //just added console.log which will display the event details in browser on click of the button. alert("Button is clicked"); console.log(event); } changemonths(event) { alert("Changed month from the Dropdown"); } }
浏览器中的输出如下 -

变量isavailable为 false,因此打印了 condition2 模板。如果单击该按钮,将调用相应的模板。
应用程序组件.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // declared array of months. months = ["January", "Feburary", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = false; //variable is set to true myClickFunction(event) { this.isavailable = !this.isavailable; // variable is toggled onclick of the button } changemonths(event) { alert("Changed month from the Dropdown"); } }
单击按钮即可切换isavailable变量,如下所示 -
myClickFunction(event) { this.isavailable = !this.isavailable; }
当您根据isavailable变量的值单击按钮时,将显示相应的模板 -


如果你检查浏览器,你会发现你永远不会在 dom 中获得 span 标签。下面的例子将帮助您理解这一点。

尽管在app.component.html中我们添加了 span 标签和<ng-template>条件,如下所示 -
<span *ngIf = "isavailable;then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid from template</ng-template> <ng-template #condition2>Condition is invalid from template</ng-template>
当我们在浏览器中检查时,我们在 dom 结构中看不到 span 标签和 <ng-template> 。
html 中的以下代码行将帮助我们获取 dom 中的 span 标签 -
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select (change) = "changemonths($event)" name = "month"> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid from template </ng-template> <ng-template #condition2>Condition is invalid from template</ng-template> </div> <button (click) = "myClickFunction($event)">Click Me</button>
如果我们删除then条件,我们会在浏览器中收到“条件有效”消息,并且 span 标签在 dom 中也可用。例如,在app.component.ts中,我们将isavailable变量设置为 true。
