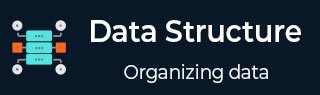
- 数据结构与算法
- DSA - 主页
- DSA - 概述
- DSA - 环境设置
- 数据结构
- DSA - 数据结构基础知识
- DSA - 数据结构和类型
- DSA - 数组数据结构
- 链表
- DSA - 链表基础知识
- DSA - 双向链表
- DSA - 循环链表
- 堆栈和队列
- DSA - 堆栈
- DSA - 表达式解析
- DSA-队列
- 图数据结构
- DSA - 图数据结构
- DSA-深度优先遍历
- DSA-广度优先遍历
- DSA——生成树
- 树数据结构
- DSA - 树数据结构
- DSA - 树遍历
- DSA - 二叉搜索树
- DSA - AVL 树
- DSA - 红黑树
- DSA - B 树
- DSA - B+ 树
- DSA - 八字树
- DSA - 尝试
- DSA-堆
- 递归
- DSA - 递归基础知识
- DSA - 河内塔
- DSA - 斐波那契数列
- DSA 有用资源
- DSA - 问题与解答
- DSA - 快速指南
- DSA - 有用的资源
- DSA - 讨论
数据结构和算法 - 展开树
展开树是二叉搜索树的修改版本,因为它包含 BST 的所有操作,如插入、删除和搜索,后面是另一个称为splaying的扩展操作。
例如,应该将值“A”插入到树中。如果树为空,则将“A”添加到树的根并退出;但如果树不为空,则使用二分查找插入操作插入元素,然后对新节点进行展开。
类似地,在展开树中搜索到元素后,由该元素组成的节点也必须展开。
但是我们如何进行展开呢?简单来说,展开只是将操作节点带到根的过程。它有六种类型的旋转。
之字形旋转
锯齿形旋转
之字形旋转
锯齿形旋转
之字形旋转
之字形旋转
之字形旋转
当操作节点是根节点或根节点的左子节点时,执行之字形旋转。节点向右旋转。

移位后,树将如下所示 -

锯齿形旋转
当操作节点是根节点或根节点的右子节点时,也会执行折线旋转。该节点向左旋转。

操作节点在移位后成为根节点 -

之字形旋转
当操作节点同时具有父节点和祖节点时,会执行之字形旋转。该节点向右旋转两个位置。

第一次旋转会将树向右移动一个位置 -

第二次右旋转将再次将节点移动一个位置。移位后的最终树将如下所示 -

锯齿形旋转
当操作节点同时具有父节点和祖节点时,也会执行锯齿状旋转。该节点向左旋转两个位置。

第一次旋转后,树将如下所示 -

那么第二次旋转后的最终树如下所示。然而,操作节点仍然不是根,因此展开被认为是不完整的。因此,在这种情况下,再次应用其他合适的旋转,直到该节点成为根。

之字形旋转
当操作节点同时具有父节点和祖节点时,执行之字形旋转。但不同的是祖父母、父母和孩子都是LRL格式。该节点首先向右旋转,然后向左旋转。

第一次旋转后,树是 -

第二次旋转后的最终树 -

之字形旋转
当操作节点同时具有父节点和祖节点时,也会执行锯齿形旋转。但不同的是祖父母、父母和孩子都是 RLR 格式。该节点首先向左旋转,然后向右旋转。

执行第一次旋转,得到的树为 -

第二次旋转后,最终的树如下所示。但操作节点还不是根节点,还需要再旋转一次才能使该节点成为根节点。

伸展树的基本操作
展开包含与二叉搜索树提供的相同基本操作:插入、删除和搜索。然而,在每个操作之后都有一个与二叉搜索树操作不同的附加操作:展开。我们已经了解了 Splaying,所以让我们了解其他操作的过程。
插入
Splay 树中的插入操作与二叉搜索树中的插入操作完全相同。在展开树中执行插入的过程如下 -
检查树是否为空;如果是,则添加新节点并退出

如果树不为空,则使用二分搜索插入将新节点添加到现有树中。

然后,选择合适的展开并将其应用于新添加的节点。

Zag(左)旋转应用于新节点

例子
以下是此操作在各种编程语言中的实现 -
#include <stdio.h> #include <stdlib.h> struct node { int data; struct node *leftChild, *rightChild; }; struct node* newNode(int data){ struct node* Node = (struct node*)malloc(sizeof(struct node)); Node->data = data; Node->leftChild = Node->rightChild = NULL; return (Node); } struct node* rightRotate(struct node *x){ struct node *y = x->leftChild; x->leftChild = y->rightChild; y->rightChild = x; return y; } struct node* leftRotate(struct node *x){ struct node *y = x->rightChild; x->rightChild = y->leftChild; y->leftChild = x; return y; } struct node* splay(struct node *root, int data){ if (root == NULL || root->data == data) return root; if (root->data > data) { if (root->leftChild == NULL) return root; if (root->leftChild->data > data) { root->leftChild->leftChild = splay(root->leftChild->leftChild, data); root = rightRotate(root); } else if (root->leftChild->data < data) { root->leftChild->rightChild = splay(root->leftChild->rightChild, data); if (root->leftChild->rightChild != NULL) root->leftChild = leftRotate(root->leftChild); } return (root->leftChild == NULL)? root: rightRotate(root); } else { if (root->rightChild == NULL) return root; if (root->rightChild->data > data) { root->rightChild->leftChild = splay(root->rightChild->leftChild, data); if (root->rightChild->leftChild != NULL) root->rightChild = rightRotate(root->rightChild); } else if (root->rightChild->data < data) { root->rightChild->rightChild = splay(root->rightChild->rightChild, data); root = leftRotate(root); } return (root->rightChild == NULL)? root: leftRotate(root); } } struct node* insert(struct node *root, int k){ if (root == NULL) return newNode(k); root = splay(root, k); if (root->data == k) return root; struct node *newnode = newNode(k); if (root->data > k) { newnode->rightChild = root; newnode->leftChild = root->leftChild; root->leftChild = NULL; } else { newnode->leftChild = root; newnode->rightChild = root->rightChild; root->rightChild = NULL; } return newnode; } void printTree(struct node *root){ if (root == NULL) return; if (root != NULL) { printTree(root->leftChild); printf("%d ", root->data); printTree(root->rightChild); } } int main(){ struct node* root = newNode(34); root->leftChild = newNode(15); root->rightChild = newNode(40); root->leftChild->leftChild = newNode(12); root->leftChild->leftChild->rightChild = newNode(14); root->rightChild->rightChild = newNode(59); printf("The Splay tree is: \n"); printTree(root); return 0; }
输出
The Splay tree is: 12 14 15 34 40 59
#include <iostream> struct node { int data; struct node *leftChild, *rightChild; }; struct node* newNode(int data){ struct node* Node = (struct node*)malloc(sizeof(struct node)); Node->data = data; Node->leftChild = Node->rightChild = NULL; return (Node); } struct node* rightRotate(struct node *x){ struct node *y = x->leftChild; x->leftChild = y->rightChild; y->rightChild = x; return y; } struct node* leftRotate(struct node *x){ struct node *y = x->rightChild; x->rightChild = y->leftChild; y->leftChild = x; return y; } struct node* splay(struct node *root, int data){ if (root == NULL || root->data == data) return root; if (root->data > data) { if (root->leftChild == NULL) return root; if (root->leftChild->data > data) { root->leftChild->leftChild = splay(root->leftChild->leftChild, data); root = rightRotate(root); } else if (root->leftChild->data < data) { root->leftChild->rightChild = splay(root->leftChild->rightChild, data); if (root->leftChild->rightChild != NULL) root->leftChild = leftRotate(root->leftChild); } return (root->leftChild == NULL)? root: rightRotate(root); } else { if (root->rightChild == NULL) return root; if (root->rightChild->data > data) { root->rightChild->leftChild = splay(root->rightChild->leftChild, data); if (root->rightChild->leftChild != NULL) root->rightChild = rightRotate(root->rightChild); } else if (root->rightChild->data < data) { root->rightChild->rightChild = splay(root->rightChild->rightChild, data); root = leftRotate(root); } return (root->rightChild == NULL)? root: leftRotate(root); } } struct node* insert(struct node *root, int k){ if (root == NULL) return newNode(k); root = splay(root, k); if (root->data == k) return root; struct node *newnode = newNode(k); if (root->data > k) { newnode->rightChild = root; newnode->leftChild = root->leftChild; root->leftChild = NULL; } else { newnode->leftChild = root; newnode->rightChild = root->rightChild; root->rightChild = NULL; } return newnode; } void printTree(struct node *root){ if (root == NULL) return; if (root != NULL) { printTree(root->leftChild); printf("%d ", root->data); printTree(root->rightChild); } } int main(){ struct node* root = newNode(34); root->leftChild = newNode(15); root->rightChild = newNode(40); root->leftChild->leftChild = newNode(12); root->leftChild->leftChild->rightChild = newNode(14); root->rightChild->rightChild = newNode(59); printf("The Splay tree is: \n"); printTree(root); return 0; }
输出
The Splay tree is: 12 14 15 34 40 59
import java.io.*; public class SplayTree { static class node { int data; node leftChild, rightChild; }; static node newNode(int data) { node Node = new node(); Node.data = data; Node.leftChild = Node.rightChild = null; return (Node); } static node rightRotate(node x) { node y = x.leftChild; x.leftChild = y.rightChild; y.rightChild = x; return y; } static node leftRotate(node x) { node y = x.rightChild; x.rightChild = y.leftChild; y.leftChild = x; return y; } static node splay(node root, int data) { if (root == null || root.data == data) return root; if (root.data > data) { if (root.leftChild == null) return root; if (root.leftChild.data > data) { root.leftChild.leftChild = splay(root.leftChild.leftChild, data); root = rightRotate(root); } else if (root.leftChild.data < data) { root.leftChild.rightChild = splay(root.leftChild.rightChild, data); if (root.leftChild.rightChild != null) root.leftChild = leftRotate(root.leftChild); } return (root.leftChild == null)? root: rightRotate(root); } else { if (root.rightChild == null) return root; if (root.rightChild.data > data) { root.rightChild.leftChild = splay(root.rightChild.leftChild, data); if (root.rightChild.leftChild != null) root.rightChild = rightRotate(root.rightChild); } else if (root.rightChild.data < data) { root.rightChild.rightChild = splay(root.rightChild.rightChild, data); root = leftRotate(root); } return (root.rightChild == null)? root: leftRotate(root); } } static node insert(node root, int k) { if (root == null) return newNode(k); root = splay(root, k); if (root.data == k) return root; node newnode = newNode(k); if (root.data > k) { newnode.rightChild = root; newnode.leftChild = root.leftChild; root.leftChild = null; } else { newnode.leftChild = root; newnode.rightChild = root.rightChild; root.rightChild = null; } return newnode; } static void printTree(node root) { if (root == null) return; if (root != null) { printTree(root.leftChild); System.out.print(root.data + " "); printTree(root.rightChild); } } public static void main(String args[]) { node root = newNode(34); root.leftChild = newNode(15); root.rightChild = newNode(40); root.leftChild.leftChild = newNode(12); root.leftChild.leftChild.rightChild = newNode(14); root.rightChild.rightChild = newNode(59); System.out.println("The Splay tree is: "); printTree(root); } }
输出
The Splay tree is: 12 14 15 34 40 59
#Python Code for Insertion Operation of Splay Trees class Node: def __init__(self, data): self.data = data self.leftChild = None self.rightChild = None def newNode(data): return Node(data) def rightRotate(x): y = x.leftChild x.leftChild = y.rightChild y.rightChild = x return y def leftRotate(x): y = x.rightChild x.rightChild = y.leftChild y.leftChild = x return y def splay(root, data): if root is None or root.data == data: return root if root.data > data: if root.leftChild is None: return root if root.leftChild.data > data: root.leftChild.leftChild = splay(root.leftChild.leftChild, data) root = rightRotate(root) elif root.leftChild.data < data: root.leftChild.rightChild = splay(root.leftChild.rightChild, data) if root.leftChild.rightChild is not None: root.leftChild = leftRotate(root.leftChild) return root if root.leftChild is None else rightRotate(root) else: if root.rightChild is None: return root if root.rightChild.data > data: root.rightChild.leftChild = splay(root.rightChild.leftChild, data) if root.rightChild.leftChild is not None: root.rightChild = rightRotate(root.rightChild) elif root.rightChild.data < data: root.rightChild.rightChild = splay(root.rightChild.rightChild, data) root = leftRotate(root) return root if root.rightChild is None else leftRotate(root) def insert(root, k): if root is None: return newNode(k) root = splay(root, k) if root.data == k: return root newnode = newNode(k) if root.data > k: newnode.rightChild = root newnode.leftChild = root.leftChild root.leftChild = None else: newnode.leftChild = root newnode.rightChild = root.rightChild root.rightChild = None return newnode def printTree(root): if root is None: return if root is not None: printTree(root.leftChild) print(root.data, end=" ") printTree(root.rightChild) if __name__ == "__main__": root = newNode(34) root.leftChild = newNode(15) root.rightChild = newNode(40) root.leftChild.leftChild = newNode(12) root.leftChild.leftChild.rightChild = newNode(14) root.rightChild.rightChild = newNode(59) print("The Splay tree is: ") printTree(root)
输出
The Splay tree is: 12 14 15 34 40 59
删除
展开树中的删除操作执行如下 -
对要删除的节点应用展开操作。
一旦该节点成为根节点,就删除该节点。
现在,树被分成两棵树,左子树和右子树;将它们各自的第一个节点作为根节点:例如 root_left 和 root_right。

如果 root_left 为 NULL 值,则 root_right 将成为树的根。反之亦然。
但如果 root_left 和 root_right 都不为 NULL 值,则从左子树中选择最大值,并通过连接子树将其作为新的根。

例子
以下是此操作在各种编程语言中的实现 -
#include <stdio.h> #include <stdlib.h> struct node { int data; struct node *leftChild, *rightChild; }; struct node* newNode(int data){ struct node* Node = (struct node*)malloc(sizeof(struct node)); Node->data = data; Node->leftChild = Node->rightChild = NULL; return (Node); } struct node* rightRotate(struct node *x){ struct node *y = x->leftChild; x->leftChild = y->rightChild; y->rightChild = x; return y; } struct node* leftRotate(struct node *x){ struct node *y = x->rightChild; x->rightChild = y->leftChild; y->leftChild = x; return y; } struct node* splay(struct node *root, int data){ if (root == NULL || root->data == data) return root; if (root->data > data) { if (root->leftChild == NULL) return root; if (root->leftChild->data > data) { root->leftChild->leftChild = splay(root->leftChild->leftChild, data); root = rightRotate(root); } else if (root->leftChild->data < data) { root->leftChild->rightChild = splay(root->leftChild->rightChild, data); if (root->leftChild->rightChild != NULL) root->leftChild = leftRotate(root->leftChild); } return (root->leftChild == NULL)? root: rightRotate(root); } else { if (root->rightChild == NULL) return root; if (root->rightChild->data > data) { root->rightChild->leftChild = splay(root->rightChild->leftChild, data); if (root->rightChild->leftChild != NULL) root->rightChild = rightRotate(root->rightChild); } else if (root->rightChild->data < data) { root->rightChild->rightChild = splay(root->rightChild->rightChild, data); root = leftRotate(root); } return (root->rightChild == NULL)? root: leftRotate(root); } } struct node* insert(struct node *root, int k){ if (root == NULL) return newNode(k); root = splay(root, k); if (root->data == k) return root; struct node *newnode = newNode(k); if (root->data > k) { newnode->rightChild = root; newnode->leftChild = root->leftChild; root->leftChild = NULL; } else { newnode->leftChild = root; newnode->rightChild = root->rightChild; root->rightChild = NULL; } return newnode; } struct node* deletenode(struct node* root, int data){ struct node* temp; if (root == NULL) return NULL; root = splay(root, data); if (data != root->data) return root; if (!root->leftChild) { temp = root; root = root->rightChild; } else { temp = root; root = splay(root->leftChild, data); root->rightChild = temp->rightChild; } free(temp); return root; } void printTree(struct node *root){ if (root == NULL) return; if (root != NULL) { printTree(root->leftChild); printf("%d ", root->data); printTree(root->rightChild); } } int main(){ struct node* root = newNode(34); root->leftChild = newNode(15); root->rightChild = newNode(40); printf("The Splay tree is \n"); printTree(root); root = deletenode(root, 40); printf("\nThe Splay tree after deletion is \n"); printTree(root); return 0; }
输出
The Splay tree is 15 34 40 The Splay tree after deletion is 15 34
#include <iostream> struct node { int data; struct node *leftChild, *rightChild; }; struct node* newNode(int data){ struct node* Node = (struct node*)malloc(sizeof(struct node)); Node->data = data; Node->leftChild = Node->rightChild = NULL; return (Node); } struct node* rightRotate(struct node *x){ struct node *y = x->leftChild; x->leftChild = y->rightChild; y->rightChild = x; return y; } struct node* leftRotate(struct node *x){ struct node *y = x->rightChild; x->rightChild = y->leftChild; y->leftChild = x; return y; } struct node* splay(struct node *root, int data){ if (root == NULL || root->data == data) return root; if (root->data > data) { if (root->leftChild == NULL) return root; if (root->leftChild->data > data) { root->leftChild->leftChild = splay(root->leftChild->leftChild, data); root = rightRotate(root); } else if (root->leftChild->data < data) { root->leftChild->rightChild = splay(root->leftChild->rightChild, data); if (root->leftChild->rightChild != NULL) root->leftChild = leftRotate(root->leftChild); } return (root->leftChild == NULL)? root: rightRotate(root); } else { if (root->rightChild == NULL) return root; if (root->rightChild->data > data) { root->rightChild->leftChild = splay(root->rightChild->leftChild, data); if (root->rightChild->leftChild != NULL) root->rightChild = rightRotate(root->rightChild); } else if (root->rightChild->data < data) { root->rightChild->rightChild = splay(root->rightChild->rightChild, data); root = leftRotate(root); } return (root->rightChild == NULL)? root: leftRotate(root); } } struct node* insert(struct node *root, int k){ if (root == NULL) return newNode(k); root = splay(root, k); if (root->data == k) return root; struct node *newnode = newNode(k); if (root->data > k) { newnode->rightChild = root; newnode->leftChild = root->leftChild; root->leftChild = NULL; } else { newnode->leftChild = root; newnode->rightChild = root->rightChild; root->rightChild = NULL; } return newnode; } struct node* deletenode(struct node* root, int data){ struct node* temp; if (root == NULL) return NULL; root = splay(root, data); if (data != root->data) return root; if (!root->leftChild) { temp = root; root = root->rightChild; } else { temp = root; root = splay(root->leftChild, data); root->rightChild = temp->rightChild; } free(temp); return root; } void printTree(struct node *root){ if (root == NULL) return; if (root != NULL) { printTree(root->leftChild); printf("%d ", root->data); printTree(root->rightChild); } } int main(){ struct node* root = newNode(34); root->leftChild = newNode(15); root->rightChild = newNode(40); printf("The Splay tree is \n"); printTree(root); root = deletenode(root, 40); printf("\nThe Splay tree after deletion is \n"); printTree(root); return 0; }
输出
The Splay tree is 15 34 40 The Splay tree after deletion is 15 34
import java.io.*; public class SplayTree { static class node { int data; node leftChild, rightChild; }; static node newNode(int data) { node Node = new node(); Node.data = data; Node.leftChild = Node.rightChild = null; return (Node); } static node rightRotate(node x) { node y = x.leftChild; x.leftChild = y.rightChild; y.rightChild = x; return y; } static node leftRotate(node x) { node y = x.rightChild; x.rightChild = y.leftChild; y.leftChild = x; return y; } static node splay(node root, int data) { if (root == null || root.data == data) return root; if (root.data > data) { if (root.leftChild == null) return root; if (root.leftChild.data > data) { root.leftChild.leftChild = splay(root.leftChild.leftChild, data); root = rightRotate(root); } else if (root.leftChild.data < data) { root.leftChild.rightChild = splay(root.leftChild.rightChild, data); if (root.leftChild.rightChild != null) root.leftChild = leftRotate(root.leftChild); } return (root.leftChild == null)? root: rightRotate(root); } else { if (root.rightChild == null) return root; if (root.rightChild.data > data) { root.rightChild.leftChild = splay(root.rightChild.leftChild, data); if (root.rightChild.leftChild != null) root.rightChild = rightRotate(root.rightChild); } else if (root.rightChild.data < data) { root.rightChild.rightChild = splay(root.rightChild.rightChild, data); root = leftRotate(root); } return (root.rightChild == null)? root: leftRotate(root); } } static node insert(node root, int k) { if (root == null) return newNode(k); root = splay(root, k); if (root.data == k) return root; node newnode = newNode(k); if (root.data > k) { newnode.rightChild = root; newnode.leftChild = root.leftChild; root.leftChild = null; } else { newnode.leftChild = root; newnode.rightChild = root.rightChild; root.rightChild = null; } return newnode; } static node deletenode(node root, int data) { node temp; if (root == null) return null; root = splay(root, data); if (data != root.data) return root; if (root.leftChild == null) { temp = root; root = root.rightChild; } else { temp = root; root = splay(root.leftChild, data); root.rightChild = temp.rightChild; } return root; } static void printTree(node root) { if (root == null) return; if (root != null) { printTree(root.leftChild); System.out.print(root.data + " "); printTree(root.rightChild); } } public static void main(String args[]) { node root = newNode(34); root.leftChild = newNode(15); root.rightChild = newNode(40); System.out.println("The Splay tree is: "); printTree(root); root = deletenode(root, 40); System.out.println("\nThe Splay tree after deletion is: "); printTree(root); } }
输出
The Splay tree is: 15 34 40 The Splay tree after deletion is: 15 34
#Python Code for Deletion operation of Splay Trees class Node: def __init__(self, data): self.data = data self.leftChild = None self.rightChild = None def newNode(data): node = Node(data) return node def rightRotate(x): y = x.leftChild x.leftChild = y.rightChild y.rightChild = x return y def leftRotate(x): y = x.rightChild x.rightChild = y.leftChild y.leftChild = x return y def splay(root, data): if root is None or root.data == data: return root if root.data > data: if root.leftChild is None: return root if root.leftChild.data > data: root.leftChild.leftChild = splay(root.leftChild.leftChild, data) root = rightRotate(root) elif root.leftChild.data < data: root.leftChild.rightChild = splay(root.leftChild.rightChild, data) if root.leftChild.rightChild is not None: root.leftChild = leftRotate(root.leftChild) return root if root.leftChild is None else rightRotate(root) else: if root.rightChild is None: return root if root.rightChild.data > data: root.rightChild.leftChild = splay(root.rightChild.leftChild, data) if root.rightChild.leftChild is not None: root.rightChild = rightRotate(root.rightChild) elif root.rightChild.data < data: root.rightChild.rightChild = splay(root.rightChild.rightChild, data) root = leftRotate(root) return root if root.rightChild is None else leftRotate(root) def insert(root, k): if root is None: return newNode(k) root = splay(root, k) if root.data == k: return root newnode = newNode(k) if root.data > k: newnode.rightChild = root newnode.leftChild = root.leftChild root.leftChild = None else: newnode.leftChild = root newnode.rightChild = root.rightChild root.rightChild = None return newnode def deletenode(root, data): temp = None if root is None: return None root = splay(root, data) if data != root.data: return root if root.leftChild is None: temp = root root = root.rightChild else: temp = root root = splay(root.leftChild, data) root.rightChild = temp.rightChild del temp return root def printTree(root): if root is None: return if root is not None: printTree(root.leftChild) print(root.data, end=" ") printTree(root.rightChild) root = newNode(34) root.leftChild = newNode(15) root.rightChild = newNode(40) print("The Splay tree is:") printTree(root) root = deletenode(root, 40) print("\nThe Splay tree after deletion is:") printTree(root)
输出
The Splay tree is: 15 34 40 The Splay tree after deletion is: 15 34
搜索
展开树中的搜索操作遵循与二叉搜索树操作相同的过程。但是,在搜索完成并找到元素后,将对搜索到的节点应用展开。如果没有找到该元素,则提示搜索失败。
例子
以下是此操作在各种编程语言中的实现 -
#include <stdio.h> #include <stdlib.h> struct node { int data; struct node *leftChild, *rightChild; }; struct node* newNode(int data){ struct node* Node = (struct node*)malloc(sizeof(struct node)); Node->data = data; Node->leftChild = Node->rightChild = NULL; return (Node); } struct node* rightRotate(struct node *x){ struct node *y = x->leftChild; x->leftChild = y->rightChild; y->rightChild = x; return y; } struct node* leftRotate(struct node *x){ struct node *y = x->rightChild; x->rightChild = y->leftChild; y->leftChild = x; return y; } struct node* splay(struct node *root, int data){ if (root == NULL || root->data == data) return root; if (root->data > data) { if (root->leftChild == NULL) return root; if (root->leftChild->data > data) { root->leftChild->leftChild = splay(root->leftChild->leftChild, data); root = rightRotate(root); } else if (root->leftChild->data < data) { root->leftChild->rightChild = splay(root->leftChild->rightChild, data); if (root->leftChild->rightChild != NULL) root->leftChild = leftRotate(root->leftChild); } return (root->leftChild == NULL)? root: rightRotate(root); } else { if (root->rightChild == NULL) return root; if (root->rightChild->data > data) { root->rightChild->leftChild = splay(root->rightChild->leftChild, data); if (root->rightChild->leftChild != NULL) root->rightChild = rightRotate(root->rightChild); } else if (root->rightChild->data < data) { root->rightChild->rightChild = splay(root->rightChild->rightChild, data); root = leftRotate(root); } return (root->rightChild == NULL)? root: leftRotate(root); } } struct node* insert(struct node *root, int k){ if (root == NULL) return newNode(k); root = splay(root, k); if (root->data == k) return root; struct node *newnode = newNode(k); if (root->data > k) { newnode->rightChild = root; newnode->leftChild = root->leftChild; root->leftChild = NULL; } else { newnode->leftChild = root; newnode->rightChild = root->rightChild; root->rightChild = NULL; } return newnode; } struct node* deletenode(struct node* root, int data){ struct node* temp; if (root == NULL) return NULL; root = splay(root, data); if (data != root->data) return root; if (!root->leftChild) { temp = root; root = root->rightChild; } else { temp = root; root = splay(root->leftChild, data); root->rightChild = temp->rightChild; } free(temp); return root; } void printTree(struct node *root){ if (root == NULL) return; if (root != NULL) { printTree(root->leftChild); printf("%d ", root->data); printTree(root->rightChild); } } int main(){ struct node* root = newNode(34); root->leftChild = newNode(15); root->rightChild = newNode(40); root->leftChild->leftChild = newNode(12); root->leftChild->leftChild->rightChild = newNode(14); root->rightChild->rightChild = newNode(59); printf("The Splay tree is \n"); printTree(root); root = deletenode(root, 40); printf("\nThe Splay tree after deletion is \n"); printTree(root); return 0; }
输出
The Splay tree is 12 14 15 34 40 59 The Splay tree after deletion is 12 14 15 34 59
#include <bits/stdc++.h> using namespace std; class node{ public: int data; node *leftChild, *rightChild; }; node* newNode(int data){ node* Node = new node(); Node->data = data; Node->leftChild = Node->rightChild = NULL; return (Node); } node *rightRotate(node *x){ node *y = x->leftChild; x->leftChild = y->rightChild; y->rightChild = x; return y; } node *leftRotate(node *x){ node *y = x->rightChild; x->rightChild = y->leftChild; y->leftChild = x; return y; } node *splay(node *root, int data){ if (root == NULL || root->data == data) return root; if (root->data > data) { if (root->leftChild == NULL) return root; if (root->leftChild->data > data) { root->leftChild->leftChild = splay(root->leftChild->leftChild, data); root = rightRotate(root); } else if (root->leftChild->data < data) { root->leftChild->rightChild = splay(root->leftChild->rightChild, data); if (root->leftChild->rightChild != NULL) root->leftChild = leftRotate(root->leftChild); } return (root->leftChild == NULL)? root: rightRotate(root); } else { if (root->rightChild == NULL) return root; if (root->rightChild->data > data) { root->rightChild->leftChild = splay(root->rightChild->leftChild, data); if (root->rightChild->leftChild != NULL) root->rightChild = rightRotate(root->rightChild); } else if (root->rightChild->data < data) { root->rightChild->rightChild = splay(root->rightChild->rightChild, data); root = leftRotate(root); } return (root->rightChild == NULL)? root: leftRotate(root); } } node* insert(node *root, int k) { if (root == NULL) return newNode(k); root = splay(root, k); if (root->data == k) return root; node *newnode = newNode(k); if (root->data > k) { newnode->rightChild = root; newnode->leftChild = root->leftChild; root->leftChild = NULL; } else { newnode->leftChild = root; newnode->rightChild = root->rightChild; root->rightChild = NULL; } return newnode; } node* deletenode(struct node* root, int data){ struct node* temp; if (root == NULL) return NULL; root = splay(root, data); if (data != root->data) return root; if (!root->leftChild) { temp = root; root = root->rightChild; } else { temp = root; root = splay(root->leftChild, data); root->rightChild = temp->rightChild; } free(temp); return root; } void printTree(node *root){ if (root == NULL) return; if (root != NULL) { printTree(root->leftChild); cout<<root->data<<" "; printTree(root->rightChild); } } int main(){ node* root = newNode(34); root->leftChild = newNode(15); root->rightChild = newNode(40); root->leftChild->leftChild = newNode(12); root->leftChild->leftChild->rightChild = newNode(14); root->rightChild->rightChild = newNode(59); cout<<"The Splay tree is \n"; printTree(root); root = deletenode(root, 40); cout<<"\nThe Splay tree after deletion is \n"; printTree(root); return 0; }
输出
The Splay tree is 12 14 15 34 40 59 The Splay tree after deletion is 12 14 15 34 59
import java.io.*; public class SplayTree { static class node { int data; node leftChild, rightChild; }; static node newNode(int data) { node Node = new node(); Node.data = data; Node.leftChild = Node.rightChild = null; return (Node); } static node rightRotate(node x) { node y = x.leftChild; x.leftChild = y.rightChild; y.rightChild = x; return y; } static node leftRotate(node x) { node y = x.rightChild; x.rightChild = y.leftChild; y.leftChild = x; return y; } static node splay(node root, int data) { if (root == null || root.data == data) return root; if (root.data > data) { if (root.leftChild == null) return root; if (root.leftChild.data > data) { root.leftChild.leftChild = splay(root.leftChild.leftChild, data); root = rightRotate(root); } else if (root.leftChild.data < data) { root.leftChild.rightChild = splay(root.leftChild.rightChild, data); if (root.leftChild.rightChild != null) root.leftChild = leftRotate(root.leftChild); } return (root.leftChild == null)? root: rightRotate(root); } else { if (root.rightChild == null) return root; if (root.rightChild.data > data) { root.rightChild.leftChild = splay(root.rightChild.leftChild, data); if (root.rightChild.leftChild != null) root.rightChild = rightRotate(root.rightChild); } else if (root.rightChild.data < data) { root.rightChild.rightChild = splay(root.rightChild.rightChild, data); root = leftRotate(root); } return (root.rightChild == null)? root: leftRotate(root); } } static node insert(node root, int k) { if (root == null) return newNode(k); root = splay(root, k); if (root.data == k) return root; node newnode = newNode(k); if (root.data > k) { newnode.rightChild = root; newnode.leftChild = root.leftChild; root.leftChild = null; } else { newnode.leftChild = root; newnode.rightChild = root.rightChild; root.rightChild = null; } return newnode; } static node deletenode(node root, int data) { node temp; if (root == null) return null; root = splay(root, data); if (data != root.data) return root; if (root.leftChild == null) { temp = root; root = root.rightChild; } else { temp = root; root = splay(root.leftChild, data); root.rightChild = temp.rightChild; } return root; } static void printTree(node root) { if (root == null) return; if (root != null) { printTree(root.leftChild); System.out.print(root.data + " "); printTree(root.rightChild); } } public static void main(String args[]) { node root = newNode(34); root.leftChild = newNode(15); root.rightChild = newNode(40); root.leftChild.leftChild = newNode(12); root.leftChild.leftChild.rightChild = newNode(14); root.rightChild.rightChild = newNode(59); System.out.println("The Splay tree is: "); printTree(root); root = deletenode(root, 40); System.out.println("\nThe Splay tree after deletion is: "); printTree(root); } }
输出
The Splay tree is: 12 14 15 34 40 59 The Splay tree after deletion is: 12 14 15 34 59
#Python Code for Search Operation of splay Trees class Node: def __init__(self, data): self.data = data self.leftChild = None self.rightChild = None def newNode(data): newNode = Node(data) newNode.leftChild = newNode.rightChild = None return newNode def rightRotate(x): y = x.leftChild x.leftChild = y.rightChild y.rightChild = x return y def leftRotate(x): y = x.rightChild x.rightChild = y.leftChild y.leftChild = x return y def splay(root, data): if root is None or root.data == data: return root if root.data > data: if root.leftChild is None: return root if root.leftChild.data > data: root.leftChild.leftChild = splay(root.leftChild.leftChild, data) root = rightRotate(root) elif root.leftChild.data < data: root.leftChild.rightChild = splay(root.leftChild.rightChild, data) if root.leftChild.rightChild is not None: root.leftChild = leftRotate(root.leftChild) return root if root.leftChild is None else rightRotate(root) else: if root.rightChild is None: return root if root.rightChild.data > data: root.rightChild.leftChild = splay(root.rightChild.leftChild, data) if root.rightChild.leftChild is not None: root.rightChild = rightRotate(root.rightChild) elif root.rightChild.data < data: root.rightChild.rightChild = splay(root.rightChild.rightChild, data) root = leftRotate(root) return root if root.rightChild is None else leftRotate(root) def insert(root, k): if root is None: return newNode(k) root = splay(root, k) if root.data == k: return root newnode = newNode(k) if root.data > k: newnode.rightChild = root newnode.leftChild = root.leftChild root.leftChild = None else: newnode.leftChild = root newnode.rightChild = root.rightChild root.rightChild = None return newnode def deletenode(root, data): temp = None if root is None: return None root = splay(root, data) if data != root.data: return root if root.leftChild is None: temp = root root = root.rightChild else: temp = root root = splay(root.leftChild, data) root.rightChild = temp.rightChild del temp return root def printTree(root): if root is None: return if root is not None: printTree(root.leftChild) print(root.data, end=" ") printTree(root.rightChild) root = newNode(34) root.leftChild = newNode(15) root.rightChild = newNode(40) root.leftChild.leftChild = newNode(12) root.leftChild.leftChild.rightChild = newNode(14) root.rightChild.rightChild = newNode(59) print("The Splay tree is") printTree(root) root = deletenode(root, 40) print("\nThe Splay tree after deletion is") printTree(root)
输出
The Splay tree is 12 14 15 34 40 59 The Splay tree after deletion is 12 14 15 34 59