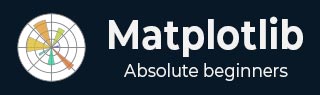
- Matplotlib 教程
- Matplotlib - 主页
- Matplotlib - 简介
- Matplotlib - 环境设置
- Matplotlib - Anaconda 分布
- Matplotlib - Jupyter 笔记本
- Matplotlib - Pyplot API
- Matplotlib - 简单绘图
- Matplotlib - PyLab 模块
- 面向对象的接口
- Matplotlib - 图形类
- Matplotlib - 轴类
- Matplotlib - 多图
- Matplotlib - Subplots() 函数
- Matplotlib - Subplot2grid() 函数
- Matplotlib - 网格
- Matplotlib - 格式化轴
- Matplotlib - 设置限制
- 设置刻度和刻度标签
- Matplotlib - 双轴
- Matplotlib - 条形图
- Matplotlib - 直方图
- Matplotlib - 饼图
- Matplotlib - 散点图
- Matplotlib - 等值线图
- Matplotlib - 箭袋图
- Matplotlib - 箱线图
- Matplotlib - 小提琴图
- 三维绘图
- Matplotlib - 3D 等高线图
- Matplotlib - 3D 线框图
- Matplotlib - 3D 曲面图
- Matplotlib - 处理文本
- 数学表达式
- Matplotlib - 处理图像
- Matplotlib - 变换
- Matplotlib 有用资源
- Matplotlib - 快速指南
- Matplotlib - 有用的资源
- Matplotlib - 讨论
Matplotlib - 简单绘图
在本章中,我们将学习如何使用 Matplotlib 创建简单的绘图。
现在,我们将在 Matplotlib 中显示以弧度表示的角度与正弦值的简单线图。首先,导入 Matplotlib 包中的 Pyplot 模块,按照约定使用别名 plt。
import matplotlib.pyplot as plt
接下来我们需要一组数字来绘制。NumPy 库中定义了各种数组函数,并使用 np 别名导入。
import numpy as np
现在,我们使用 NumPy 库中的 arange() 函数获取角度在 0 到 2π 之间的 ndarray 对象。
x = np.arange(0, math.pi*2, 0.05)
ndarray 对象充当图表 x 轴上的值。要在 y 轴上显示的 x 角度的相应正弦值通过以下语句获得 -
y = np.sin(x)
使用plot() 函数绘制两个数组的值。
plt.plot(x,y)
您可以设置绘图标题以及 x 轴和 y 轴的标签。
You can set the plot title, and labels for x and y axes. plt.xlabel("angle") plt.ylabel("sine") plt.title('sine wave')
绘图查看器窗口由 show() 函数调用 -
plt.show()
完整的程序如下 -
from matplotlib import pyplot as plt import numpy as np import math #needed for definition of pi x = np.arange(0, math.pi*2, 0.05) y = np.sin(x) plt.plot(x,y) plt.xlabel("angle") plt.ylabel("sine") plt.title('sine wave') plt.show()
当执行上面的代码行时,将显示下图 -
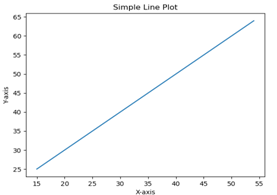
现在,将 Jupyter Notebook 与 Matplotlib 结合使用。
如前所述,从 Anaconda 导航器或命令行启动 Jupyter 笔记本。在输入单元格中,输入 Pyplot 和 NumPy 的导入语句 -
from matplotlib import pyplot as plt import numpy as np
要在笔记本本身内部(而不是在单独的查看器中)显示绘图输出,请输入以下魔术语句 -
%matplotlib inline
获取 x 作为包含 0 到 2π 之间弧度的角度的 ndarray 对象,以及 y 作为每个角度的正弦值 -
import math x = np.arange(0, math.pi*2, 0.05) y = np.sin(x)
设置 x 和 y 轴的标签以及绘图标题 -
plt.xlabel("angle") plt.ylabel("sine") plt.title('sine wave')
最后执行plot()函数在笔记本中生成正弦波显示(无需运行show()函数) -
plt.plot(x,y)
执行最后一行代码后,将显示以下输出 -
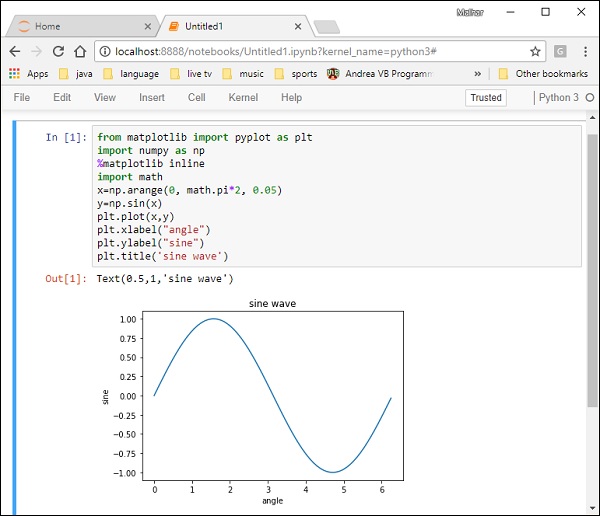