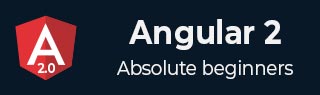
- Angular 2 教程
- Angular 2 - 主页
- Angular 2 - 概述
- Angular 2 - 环境
- Angular 2 - 你好世界
- Angular 2 - 模块
- Angular 2 - 架构
- Angular 2 - 组件
- Angular 2 - 模板
- Angular 2 - 指令
- Angular 2 - 元数据
- Angular 2 - 数据绑定
- 使用 HTTP 进行 CRUD 操作
- Angular 2 - 错误处理
- Angular 2 - 路由
- Angular 2 - 导航
- Angular 2 - 表单
- Angular 2 - CLI
- Angular 2 - 依赖注入
- Angular 2 - 高级配置
- Angular 2 - 第三方控件
- Angular 2 - 数据显示
- Angular 2 - 处理事件
- Angular 2 - 转换数据
- Angular 2 - 自定义管道
- Angular 2 - 用户输入
- Angular 2 - 生命周期挂钩
- Angular 2 - 嵌套容器
- Angular 2 - 服务
- Angular 2 有用资源
- Angular 2 - 问题与解答
- Angular 2 - 快速指南
- Angular 2 - 有用的资源
- Angular 2 - 讨论
Angular 2 - 转换数据
Angular 2 有很多可用于转换数据的过滤器和管道。
小写
这用于将输入转换为全部小写。
句法
Propertyvalue | lowercase
参数
没有任何
结果
属性值将转换为小写。
例子
首先确保 app.component.ts 文件中存在以下代码。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { TutorialName: string = 'Angular JS2'; appList: string[] = ["Binding", "Display", "Services"]; }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The name of this Tutorial is {{TutorialName}}<br> The first Topic is {{appList[0] | lowercase}}<br> The second Topic is {{appList[1] | lowercase}}<br> The third Topic is {{appList[2]| lowercase}}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
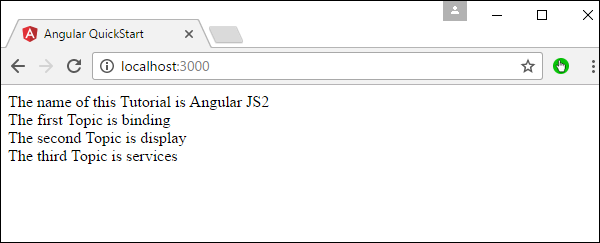
大写
这用于将输入转换为全部大写。
句法
Propertyvalue | uppercase
参数
没有任何。
结果
属性值将转换为大写。
例子
首先确保 app.component.ts 文件中存在以下代码。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { TutorialName: string = 'Angular JS2'; appList: string[] = ["Binding", "Display", "Services"]; }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The name of this Tutorial is {{TutorialName}}<br> The first Topic is {{appList[0] | uppercase }}<br> The second Topic is {{appList[1] | uppercase }}<br> The third Topic is {{appList[2]| uppercase }}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
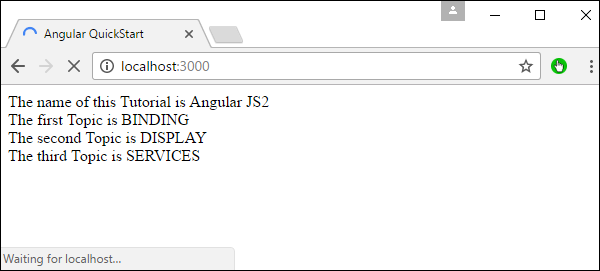
片
这用于从输入字符串中切片数据。
句法
Propertyvalue | slice:start:end
参数
start - 这是切片应该开始的起始位置。
end - 这是切片应结束的起始位置。
结果
属性值将根据开始和结束位置进行切片。
例子
首先确保 app.component.ts 文件中存在以下代码
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { TutorialName: string = 'Angular JS2'; appList: string[] = ["Binding", "Display", "Services"]; }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The name of this Tutorial is {{TutorialName}}<br> The first Topic is {{appList[0] | slice:1:2}}<br> The second Topic is {{appList[1] | slice:1:3}}<br> The third Topic is {{appList[2]| slice:2:3}}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
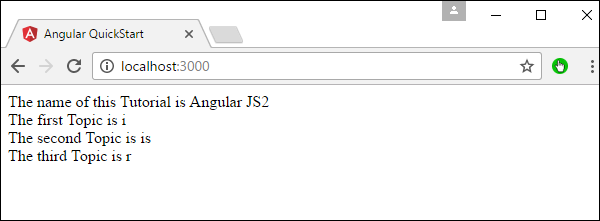
日期
这用于将输入字符串转换为日期格式。
句法
Propertyvalue | date:”dateformat”
参数
dateformat - 这是输入字符串应转换为的日期格式。
结果
属性值将转换为日期格式。
例子
首先确保 app.component.ts 文件中存在以下代码。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { newdate = new Date(2016, 3, 15); }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The date of this Tutorial is {{newdate | date:"MM/dd/yy"}}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
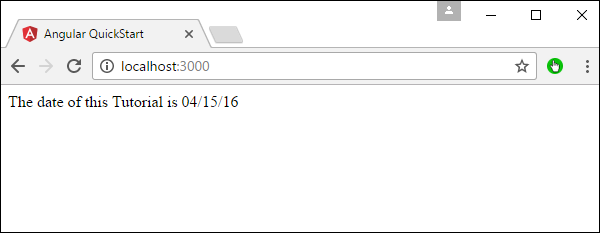
货币
这用于将输入字符串转换为货币格式。
句法
Propertyvalue | currency
参数
没有任何。
结果
属性值将转换为货币格式。
例子
首先确保 app.component.ts 文件中存在以下代码。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { newValue: number = 123; }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The currency of this Tutorial is {{newValue | currency}}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
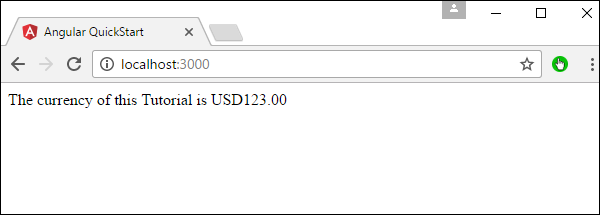
百分比
这用于将输入字符串转换为百分比格式。
句法
Propertyvalue | percent
参数
没有任何
结果
属性值将转换为百分比格式。
例子
首先确保 app.component.ts 文件中存在以下代码。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { newValue: number = 30; }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The percentage is {{newValue | percent}}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
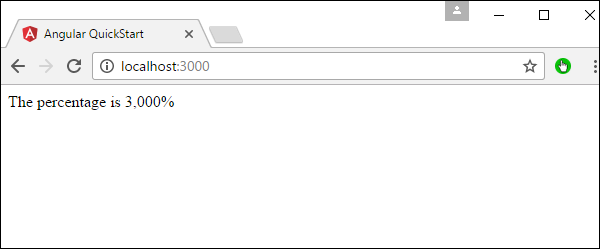
百分比管道还有另一种变化,如下所示。
句法
Propertyvalue | percent: ‘{minIntegerDigits}.{minFractionDigits}{maxFractionDigits}’
参数
minIntegerDigits - 这是整数位数的最小数量。
minFractionDigits - 这是小数位数的最小数量。
maxFractionDigits - 这是小数位数的最大数量。
结果
属性值将转换为百分比格式
例子
首先确保 app.component.ts 文件中存在以下代码。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', templateUrl: 'app/app.component.html' }) export class AppComponent { newValue: number = 0.3; }
接下来,确保 app/app.component.html 文件中存在以下代码。
<div> The percentage is {{newValue | percent:'2.2-5'}}<br> </div>
输出
保存所有代码更改并刷新浏览器后,您将获得以下输出。
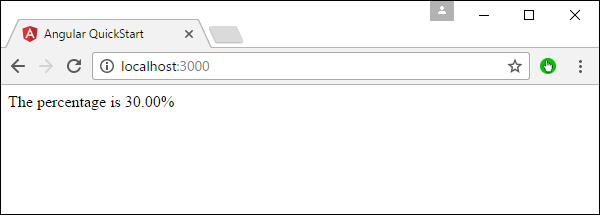