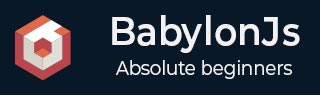
- BabylonJS 教程
- BabylonJS - 主页
- BabylonJS - 简介
- BabylonJS - 环境设置
- BabylonJS - 概述
- BabylonJS - 基本元素
- BabylonJS - 材料
- BabylonJS - 动画
- BabylonJS - 相机
- BabylonJS - 灯
- BabylonJS - 参数化形状
- BabylonJS - 网格
- 矢量位置和旋转
- BabylonJS - 贴花
- BabylonJS - Curve3
- BabylonJS - 动态纹理
- BabylonJS - 视差映射
- BabylonJS - 镜头光晕
- BabylonJS - 创建屏幕截图
- BabylonJS - 反射探针
- 标准渲染管线
- BabylonJS - 着色器材质
- BabylonJS - 骨骼和骨骼
- BabylonJS - 物理引擎
- BabylonJS - 播放声音和音乐
- BabylonJS 有用资源
- BabylonJS - 快速指南
- BabylonJS - 有用的资源
- BabylonJS - 讨论
BabylonJS - Curve3
BabylonJS 内置了 api 来创建一些复杂的数学曲线。我们之前看到过丝带,即使用复杂方程创建的线条来绘制图案并计算给予网格的路径的坐标。我们这里有一个内置的 API,以避免进行复杂的计算,就像在 Curves API 中一样。
解释的曲线如下 -
- 二次贝塞尔曲线
- 三次贝塞尔曲线
- 厄米样条
- Catmull-Rom 样条线
二次贝塞尔曲线
在本节中,我们将了解二次贝塞尔曲线。
句法
var bezier = BABYLON.Curve3.CreateQuadraticBezier(origin, control, destination, nb_of_points);
参数
考虑以下与二次贝塞尔曲线相关的参数。
原点- 曲线的原点。
Control - 曲线的控制点。
目的地- 目的地点。
Noofpoints - 数组中的点。
三次贝塞尔曲线
在本节中,我们将了解三次贝塞尔曲线。
句法
var bezier3 = BABYLON.Curve3.CreateCubicBezier(origin, control1, control2, destination, nb_of_points)
参数
考虑以下与三次贝塞尔曲线相关的参数。
原点- 原点。
control1 - 矢量形式的第一个控制点。
control2 - 矢量形式的第二个控制点。
目的地- 矢量形式的目的地点。
no_of_points - 数组形式的点数。
Hermite样条曲线
在本节中,我们将了解 Hermite 样条曲线。
句法
var hermite = BABYLON.Curve3.CreateHermiteSpline(p1, t1, p2, t2, nbPoints);
参数
考虑以下与 Hermite 样条曲线相关的参数 -
p1 - 曲线的原点。
t1 - 原点切向量点。
p2 - 目标点。
t2 - 目标切向量。
NbPoints - 最终曲线的点数组。
Catmull-Rom 样条曲线
在本节中,我们将了解 Catmull-Rom 样条曲线。
句法
var nbPoints = 20; // the number of points between each Vector3 control points var points = [vec1, vec2, ..., vecN]; // an array of Vector3 the curve must pass through : the control points var catmullRom = BABYLON.Curve3.CreateCatmullRomSpline(points, nbPoints);
参数
考虑以下与 Catmull-Rom 样条曲线相关的参数 -
Points - Vector3 数组,曲线必须通过控制点。
NbPoints - 每个 Vector3 控制点之间的点数。
var path = catmullRom.getPoints(); // getPoints() returns an array of successive Vector3. var l = catmullRom.length(); // method returns the curve length.
演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); // camera var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(5, 3, 0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -100)); camera.attachControl(canvas, true); // lights var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(1, 0.5, 0), scene); light.intensity = 0.8; var spot = new BABYLON.SpotLight( "spot", new BABYLON.Vector3(25, 15, -10), new BABYLON.Vector3(-1, -0.8, 1), 15, 1, scene); spot.diffuse = new BABYLON.Color3(1, 1, 1); spot.specular = new BABYLON.Color3(0, 0, 0); spot.intensity = 0.2; // material var mat = new BABYLON.StandardMaterial("mat1", scene); mat.alpha = 1.0; mat.diffuseColor = new BABYLON.Color3(0.5, 0.5, 1.0); mat.backFaceCulling = false; //mat.wireframe = true; var makeTextPlane = function(text, color, size) { var dynamicTexture = new BABYLON.DynamicTexture("DynamicTexture", 50, scene, true); dynamicTexture.hasAlpha = true; dynamicTexture.drawText(text, 5, 40, "bold 36px Arial", color , "transparent", true); var plane = new BABYLON.Mesh.CreatePlane("TextPlane", size, scene, true); plane.material = new BABYLON.StandardMaterial("TextPlaneMaterial", scene); plane.material.backFaceCulling = false; plane.material.specularColor = new BABYLON.Color3(0, 0, 0); plane.material.diffuseTexture = dynamicTexture; return plane; }; // show axis var showAxis = function(size) { var axisX = BABYLON.Mesh.CreateLines("axisX", [ new BABYLON.Vector3(-size * 0.95, 0.05 * size, 0), new BABYLON.Vector3(-size, 0, 0), new BABYLON.Vector3(-size * 0.95, -0.05 * size, 0), new BABYLON.Vector3(-size, 0, 0), new BABYLON.Vector3.Zero(), new BABYLON.Vector3(size, 0, 0), new BABYLON.Vector3(size * 0.95, 0.05 * size, 0), new BABYLON.Vector3(size, 0, 0), new BABYLON.Vector3(size * 0.95, -0.05 * size, 0) ], scene); axisX.color = new BABYLON.Color3(1, 0, 0); var xChar = makeTextPlane("X", "red", size / 10); xChar.position = new BABYLON.Vector3(0.9 * size, -0.05 * size, 0); var xChar1 = makeTextPlane("-X", "red", size / 10); xChar1.position = new BABYLON.Vector3(-0.9 * size, 0.05 * size, 0); var xcor = []; for (i =- 20; i <= 20; i++) { xcor[i] = makeTextPlane(i, "red", size / 10); xcor[i].position = new BABYLON.Vector3(i, 0, 0); } var axisY = BABYLON.Mesh.CreateLines("axisY", [ new BABYLON.Vector3( -0.05 * size, -size * 0.95, 0), new BABYLON.Vector3(0, -size, 0), new BABYLON.Vector3(0.05 * size, -size * 0.95, 0), new BABYLON.Vector3(0, -size, 0), new BABYLON.Vector3.Zero(), new BABYLON.Vector3(0, size, 0), new BABYLON.Vector3( -0.05 * size, size * 0.95, 0), new BABYLON.Vector3(0, size, 0), new BABYLON.Vector3( 0.05 * size, size * 0.95, 0) ], scene); axisY.color = new BABYLON.Color3(0, 1, 0); var yChar = makeTextPlane("Y", "green", size / 10); yChar.position = new BABYLON.Vector3(0, 0.9 * size, -0.05 * size); var yChar1 = makeTextPlane("-Y", "green", size / 10); yChar1.position = new BABYLON.Vector3(0, -0.9 * size, 0.05 * size); var ycor = []; for (y =- 20; y <= 20; y++) { xcor[y] = makeTextPlane(y, "green", size / 10); xcor[y].position = new BABYLON.Vector3(0, y, 0); } var axisZ = BABYLON.Mesh.CreateLines("axisZ", [ new BABYLON.Vector3( 0 , -0.05 * size, -size * 0.95), new BABYLON.Vector3(0, 0, -size), new BABYLON.Vector3( 0 , 0.05 * size, -size * 0.95), new BABYLON.Vector3(0, 0, -size), new BABYLON.Vector3.Zero(), new BABYLON.Vector3(0, 0, size), new BABYLON.Vector3( 0 , -0.05 * size, size * 0.95), new BABYLON.Vector3(0, 0, size), new BABYLON.Vector3( 0, 0.05 * size, size * 0.95) ], scene); axisZ.color = new BABYLON.Color3(0, 0, 1); var zChar = makeTextPlane("Z", "blue", size / 10); zChar.position = new BABYLON.Vector3(0, 0.05 * size, 0.9 * size); var zChar1 = makeTextPlane("-Z", "blue", size / 10); zChar1.position = new BABYLON.Vector3(0, 0.05 * size, -0.9 * size); var zcor = []; for (z =- 20; z <= 20; z++) { xcor[z] = makeTextPlane(z, "green", size / 10); xcor[z].position = new BABYLON.Vector3(0, 0, z); } }; var quadraticBezierVectors = BABYLON.Curve3.CreateQuadraticBezier( BABYLON.Vector3.Zero(), new BABYLON.Vector3(10, 5, 5), new BABYLON.Vector3(5, 10, 0), 15); var quadraticBezierCurve = BABYLON.Mesh.CreateLines("qbezier", quadraticBezierVectors.getPoints(), scene); quadraticBezierCurve.color = new BABYLON.Color3(1, 1, 0.5); var cubicBezierVectors = BABYLON.Curve3.CreateCubicBezier( BABYLON.Vector3.Zero(), new BABYLON.Vector3(10, 5, 20), new BABYLON.Vector3(-50, 5, -20), new BABYLON.Vector3( -10, 20, 10), 60); var cubicBezierCurve = BABYLON.Mesh.CreateLines("cbezier", cubicBezierVectors.getPoints(), scene); cubicBezierCurve.color = new BABYLON.Color3(1, 0, 0); var continued = cubicBezierVectors.continue(cubicBezierVectors).continue(quadraticBezierVectors); var points = continued.getPoints(); var nbPoints = 60; var l = continued.length() / 2; var p1 = points[points.length - 1]; var t1 = (p1.subtract(points[points.length - 2])).scale(l); var p2 = points[0]; var t2 = (points[1].subtract(p2)).scale(l); var hermite = BABYLON.Curve3.CreateHermiteSpline(p1, t1, p2, t2, nbPoints); continued = continued.continue(hermite); var points = continued.getPoints(); var continuedCurve = BABYLON.Mesh.CreateLines("continued", points, scene); continuedCurve.position = new BABYLON.Vector3(20, -20, 20); continuedCurve.color = new BABYLON.Color3(0, 0, 0); var nbPoints = 20; // the number of points between each Vector3 control points var points = [new BABYLON.Vector3(10, 5, 20), new BABYLON.Vector3(-20, 5, -20), new BABYLON.Vector3(-25, 5, -20), new BABYLON.Vector3( -30, 20, 10),]; // an array of Vector3 the curve must pass through : the control points var catmullRom = BABYLON.Curve3.CreateCatmullRomSpline(points, nbPoints); var path = catmullRom.getPoints(); var l = catmullRom.length(); var finalcatmullCurve = BABYLON.Mesh.CreateLines("continued", path, scene); var mySinus = []; for (var i = 0; i < 30; i++) { mySinus.push( new BABYLON.Vector3(i, Math.sin(i / 10), 0) ); } var mySinusCurve3 = new BABYLON.Curve3(mySinus); var myFullCurve = mySinusCurve3.continue(cubicBezierVectors).continue(quadraticBezierVectors); var points1 = myFullCurve.getPoints(); var curve3d = BABYLON.Mesh.CreateLines("continued", points1, scene); curve3d.color = new BABYLON.Color3(0.9, 1, 0.2); showAxis(20); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
上面的代码行将生成以下输出 -
