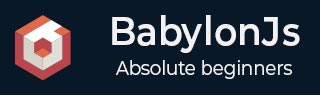
- BabylonJS 教程
- BabylonJS - 主页
- BabylonJS - 简介
- BabylonJS - 环境设置
- BabylonJS - 概述
- BabylonJS - 基本元素
- BabylonJS - 材料
- BabylonJS - 动画
- BabylonJS - 相机
- BabylonJS - 灯
- BabylonJS - 参数化形状
- BabylonJS - 网格
- 矢量位置和旋转
- BabylonJS - 贴花
- BabylonJS - Curve3
- BabylonJS - 动态纹理
- BabylonJS - 视差映射
- BabylonJS - 镜头光晕
- BabylonJS - 创建屏幕截图
- BabylonJS - 反射探针
- 标准渲染管线
- BabylonJS - 着色器材质
- BabylonJS - 骨骼和骨骼
- BabylonJS - 物理引擎
- BabylonJS - 播放声音和音乐
- BabylonJS 有用资源
- BabylonJS - 快速指南
- BabylonJS - 有用的资源
- BabylonJS - 讨论
BabylonJS - 动态纹理
BabylonJS 的动态纹理创建了一个画布,您可以轻松地在纹理上书写文本。它还允许您使用画布并使用 html5 画布可用的所有功能来与动态纹理一起使用。
我们将研究一个示例,它将展示如何在纹理上写入文本,并在我们创建的网格上绘制贝塞尔曲线。
句法
以下是创建动态纹理的语法 -
var myDynamicTexture = new BABYLON.DynamicTexture(name, option, scene);
参数
以下是创建动态纹理所需的参数 -
name - 动态纹理的名称
选项- 将具有动态纹理的宽度和高度
场景- 创建的场景
句法
以下是在纹理上写入文本的语法 -
myDynamicTexture.drawText(text, x, y, font, color, canvas color, invertY, update);
参数
以下是在纹理上写入文本所需的参数 -
文本- 要写入的文本;
x − 距左侧边缘的距离;
Y − 距顶部或底部边缘的距离,取决于 invertY;
font - 字体定义,格式为 font-style, font-size, font_name;
invertY - 默认情况下为 true,此时 y 是距顶部的距离,当为 false 时,y 是距底部的距离且字母反转;
update - 默认情况下为 true,动态纹理将立即更新。
演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>MDN Games: Babylon.js demo - shapes</title> <script src = "https://end3r.github.io/MDN-Games-3D/Babylon.js/js/babylon.js"></script> <style> html,body,canvas { margin: 0; padding: 0; width: 100%; height: 100%; font-size: 0; } </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); var camera = new BABYLON.ArcRotateCamera("Camera", -Math.PI/2, Math.PI / 3, 25, BABYLON.Vector3.Zero(), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var box = BABYLON.Mesh.CreateBox("box", 3.0, scene); box.position = new BABYLON.Vector3(0, 0, -5); //Create dynamic texture var textureGround = new BABYLON.DynamicTexture("dynamic texture", {width:512, height:256}, scene); var textureContext = textureGround.getContext(); var materialGround = new BABYLON.StandardMaterial("Mat", scene); materialGround.diffuseTexture = textureGround; box.material = materialGround; //Add text to dynamic texture var font = "bold 60px Arial"; textureGround.drawText("Box", 200, 150, font, "green", "white", true, true); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出

动态纹理还允许在动态纹理上使用 html5 canvas 方法和属性,如下所示 -
句法
var ctx = myDynamicTexture.getContext();
演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title> Babylon.JS : Demo2</title> <script src = "babylon.js"></script> <style> canvas { width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function () { var scene = new BABYLON.Scene(engine); var camera = new BABYLON.ArcRotateCamera("Camera", -Math.PI/2, Math.PI / 3, 25, BABYLON.Vector3.Zero(), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 1, 0), scene); light.intensity = 0.7; var ground = BABYLON.MeshBuilder.CreateGround("ground1", {width: 20, height: 10, subdivisions: 25}, scene); //Create dynamic texture var textureGround = new BABYLON.DynamicTexture("dynamic texture", 512, scene); var textureContext = textureGround.getContext(); var materialGround = new BABYLON.StandardMaterial("Mat", scene); materialGround.diffuseTexture = textureGround; ground.material = materialGround; //Draw on canvas textureContext.beginPath(); textureContext.moveTo(75,40); textureContext.bezierCurveTo(75,37,70,25,50,25); textureContext.bezierCurveTo(20,25,20,62.5,20,62.5); textureContext.bezierCurveTo(20,80,40,102,75,120); textureContext.bezierCurveTo(110,102,130,80,130,62.5); textureContext.bezierCurveTo(130,62.5,130,25,100,25); textureContext.bezierCurveTo(85,25,75,37,75,40); textureContext.fillStyle = "red"; textureContext.fill(); textureGround.update(); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出

解释
我们创建了地面网格并为其添加了动态纹理。
//ground mesh var ground = BABYLON.MeshBuilder.CreateGround("ground1", {width: 20, height: 10, subdivisions: 25}, scene); //Create dynamic texture var textureGround = new BABYLON.DynamicTexture("dynamic texture", 512, scene); //adding dynamic texture to ground using standard material var materialGround = new BABYLON.StandardMaterial("Mat", scene); materialGround.diffuseTexture = textureGround; ground.material = materialGround;
要在动态纹理上使用画布,我们需要首先调用画布方法 -
var textureContext = textureGround.getContext()
在画布上,我们将添加贝塞尔曲线,如下所示 -
textureContext.beginPath(); textureContext.moveTo(75,40); textureContext.bezierCurveTo(75,37,70,25,50,25); textureContext.bezierCurveTo(20,25,20,62.5,20,62.5); textureContext.bezierCurveTo(20,80,40,102,75,120); textureContext.bezierCurveTo(110,102,130,80,130,62.5); textureContext.bezierCurveTo(130,62.5,130,25,100,25); textureContext.bezierCurveTo(85,25,75,37,75,40); textureContext.fillStyle = "red"; textureContext.fill(); textureGround.update();