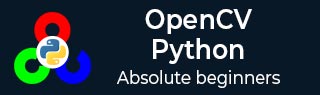
- OpenCV Python 教程
- OpenCV Python - 主页
- OpenCV Python - 概述
- OpenCV Python - 环境
- OpenCV Python - 读取图像
- OpenCV Python - 写入图像
- OpenCV Python - 使用 Matplotlib
- OpenCV Python - 图像属性
- OpenCV Python - 位运算
- OpenCV Python - 形状和文本
- OpenCV Python - 鼠标事件
- OpenCV Python - 添加轨迹栏
- OpenCV Python - 调整大小和旋转
- OpenCV Python - 图像阈值
- OpenCV Python - 图像过滤
- OpenCV Python - 边缘检测
- OpenCV Python - 直方图
- OpenCV Python - 颜色空间
- OpenCV Python - 转换
- OpenCV Python - 图像轮廓
- OpenCV Python - 模板匹配
- OpenCV Python - 图像Pyramid
- OpenCV Python - 图像相加
- OpenCV Python - 图像混合
- OpenCV Python - 傅里叶变换
- OpenCV Python - 捕获视频
- OpenCV Python - 播放视频
- OpenCV Python - 视频图像
- OpenCV Python - 来自图像的视频
- OpenCV Python - 人脸检测
- OpenCV Python - Meanshift/Camshift
- OpenCV Python - 特征检测
- OpenCV Python - 特征匹配
- OpenCV Python - 数字识别
- OpenCV Python 资源
- OpenCV Python - 快速指南
- OpenCV Python - 资源
- OpenCV Python - 讨论
OpenCV Python - 位运算
位运算用于图像处理和提取图像中的基本部分。
OpenCV 中实现了以下运算符 -
- 按位与
- 按位或
- 按位异或
- 按位非
实施例1
为了演示这些运算符的使用,我们拍摄了两张带有实心圆和空心圆的图像。
以下程序演示了 OpenCV-Python 中按位运算符的使用 -
import cv2 import numpy as np img1 = cv2.imread('a.png') img2 = cv2.imread('b.png') dest1 = cv2.bitwise_and(img2, img1, mask = None) dest2 = cv2.bitwise_or(img2, img1, mask = None) dest3 = cv2.bitwise_xor(img1, img2, mask = None) cv2.imshow('A', img1) cv2.imshow('B', img2) cv2.imshow('AND', dest1) cv2.imshow('OR', dest2) cv2.imshow('XOR', dest3) cv2.imshow('NOT A', cv2.bitwise_not(img1)) cv2.imshow('NOT B', cv2.bitwise_not(img2)) if cv2.waitKey(0) & 0xff == 27: cv2.destroyAllWindows()
输出



实施例2
在另一个涉及按位运算的示例中,opencv 徽标叠加在另一张图像上。在这里,我们通过对徽标调用threshold()函数获得一个掩码数组,并在它们之间进行AND运算。
类似地,通过 NOT 运算,我们得到一个反掩码。另外,我们得到与背景图像的“与”。
以下是确定按位运算的使用的程序 -
import cv2 as cv import numpy as np img1 = cv.imread('lena.jpg') img2 = cv.imread('whitelogo.png') rows,cols,channels = img2.shape roi = img1[0:rows, 0:cols] img2gray = cv.cvtColor(img2,cv.COLOR_BGR2GRAY) ret, mask = cv.threshold(img2gray, 10, 255, cv.THRESH_BINARY) mask_inv = cv.bitwise_not(mask) # Now black-out the area of logo img1_bg = cv.bitwise_and(roi,roi,mask = mask_inv) # Take only region of logo from logo image. img2_fg = cv.bitwise_and(img2,img2,mask = mask) # Put logo in ROI dst = cv.add(img2_fg, img1_bg) img1[0:rows, 0:cols ] = dst cv.imshow(Result,img1) cv.waitKey(0) cv.destroyAllWindows()
输出
蒙版图像给出以下结果 -
