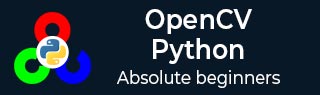
- OpenCV Python 教程
- OpenCV Python - 主页
- OpenCV Python - 概述
- OpenCV Python - 环境
- OpenCV Python - 读取图像
- OpenCV Python - 写入图像
- OpenCV Python - 使用 Matplotlib
- OpenCV Python - 图像属性
- OpenCV Python - 位运算
- OpenCV Python - 形状和文本
- OpenCV Python - 鼠标事件
- OpenCV Python - 添加轨迹栏
- OpenCV Python - 调整大小和旋转
- OpenCV Python - 图像阈值
- OpenCV Python - 图像过滤
- OpenCV Python - 边缘检测
- OpenCV Python - 直方图
- OpenCV Python - 颜色空间
- OpenCV Python - 转换
- OpenCV Python - 图像轮廓
- OpenCV Python - 模板匹配
- OpenCV Python - 图像Pyramid
- OpenCV Python - 图像相加
- OpenCV Python - 图像混合
- OpenCV Python - 傅里叶变换
- OpenCV Python - 捕获视频
- OpenCV Python - 播放视频
- OpenCV Python - 视频图像
- OpenCV Python - 来自图像的视频
- OpenCV Python - 人脸检测
- OpenCV Python - Meanshift/Camshift
- OpenCV Python - 特征检测
- OpenCV Python - 特征匹配
- OpenCV Python - 数字识别
- OpenCV Python 资源
- OpenCV Python - 快速指南
- OpenCV Python - 资源
- OpenCV Python - 讨论
OpenCV Python - 模板匹配
模板匹配技术用于检测图像中与样本或模板图像匹配的一个或多个区域。
OpenCV 中的Cv.matchTemplate()函数就是为此目的而定义的,其命令如下:
cv.matchTemplate(image, templ, method)
其中 image 是 templ(模板)图案所在的输入图像。方法参数采用以下值之一 -
- CV.TM_CCOEFF,
- CV.TM_CCOEFF_NORMED、CV.TM_CCORR、
- CV.TM_CCORR_NORMED,
- CV.TM_SQDIFF,
- CV.TM_SQDIFF_NORMED
此方法将模板图像滑动到输入图像上。这是与卷积类似的过程,比较模板图像和模板图像下的输入图像的块。
它返回一个灰度图像,其每个像素表示它与模板的匹配程度。如果输入图像的尺寸为 (WxH),模板图像的尺寸为 (wxh),则输出图像的尺寸将为 (W-w+1, H-h+1)。因此,该矩形就是您的模板区域。
例子
在下面的示例中,具有印度板球运动员 Virat Kohli 脸部的图像被用作模板,以与描绘他与另一位印度板球运动员 MSDhoni 的照片的另一张图像进行匹配。
以下程序使用 80% 的阈值并在匹配的脸部周围绘制一个矩形 -
import cv2 import numpy as np img = cv2.imread('Dhoni-and-Virat.jpg',1) cv2.imshow('Original',img) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) template = cv2.imread('virat.jpg',0) cv2.imshow('Template',template) w,h = template.shape[0], template.shape[1] matched = cv2.matchTemplate(gray,template,cv2.TM_CCOEFF_NORMED) threshold = 0.8 loc = np.where( matched >= threshold) for pt in zip(*loc[::-1]): cv2.rectangle(img, pt, (pt[0] + w, pt[1] + h), (0,255,255), 2) cv2.imshow('Matched with Template',img)
输出
原始图像、模板和结果的匹配图像如下 -
原图
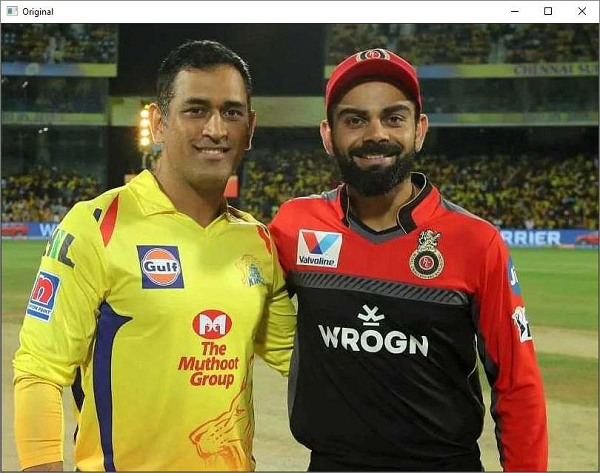
模板如下-

与模板匹配时的图像如下 -
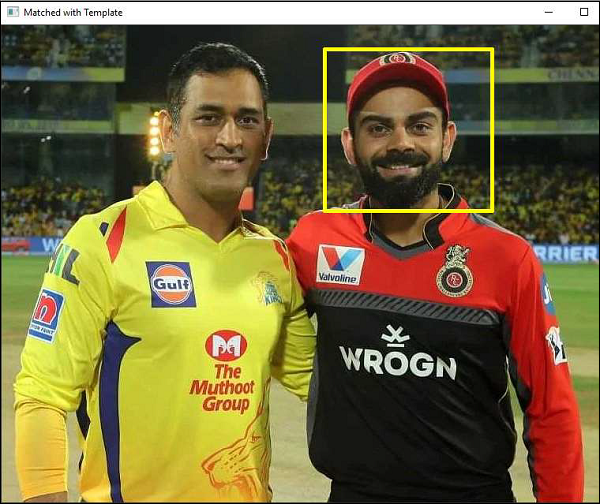