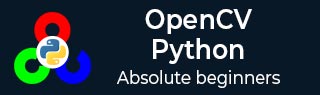
- OpenCV Python 教程
- OpenCV Python - 主页
- OpenCV Python - 概述
- OpenCV Python - 环境
- OpenCV Python - 读取图像
- OpenCV Python - 写入图像
- OpenCV Python - 使用 Matplotlib
- OpenCV Python - 图像属性
- OpenCV Python - 位运算
- OpenCV Python - 形状和文本
- OpenCV Python - 鼠标事件
- OpenCV Python - 添加轨迹栏
- OpenCV Python - 调整大小和旋转
- OpenCV Python - 图像阈值
- OpenCV Python - 图像过滤
- OpenCV Python - 边缘检测
- OpenCV Python - 直方图
- OpenCV Python - 颜色空间
- OpenCV Python - 转换
- OpenCV Python - 图像轮廓
- OpenCV Python - 模板匹配
- OpenCV Python - 图像Pyramid
- OpenCV Python - 图像相加
- OpenCV Python - 图像混合
- OpenCV Python - 傅里叶变换
- OpenCV Python - 捕获视频
- OpenCV Python - 播放视频
- OpenCV Python - 视频图像
- OpenCV Python - 来自图像的视频
- OpenCV Python - 人脸检测
- OpenCV Python - Meanshift/Camshift
- OpenCV Python - 特征检测
- OpenCV Python - 特征匹配
- OpenCV Python - 数字识别
- OpenCV Python 资源
- OpenCV Python - 快速指南
- OpenCV Python - 资源
- OpenCV Python - 讨论
OpenCV Python - 调整图像大小和旋转图像
在本章中,我们将学习如何借助 OpenCVPython 调整图像大小和旋转图像。
调整图像大小
可以使用 cv2.resize() 函数放大或缩小图像。
resize ()函数的用法如下 -
resize(src, dsize, dst, fx, fy, interpolation)
一般来说,插值是估计已知数据点之间的值的过程。
当图形数据包含间隙,但间隙两侧或间隙内的几个特定点有可用数据时。插值允许我们估计间隙内的值。
在上面的 resize() 函数中,插值标志确定用于计算目标图像大小的插值类型。
插值类型
插值的类型如下 -
INTER_NEAREST - 最近邻插值。
INTER_LINEAR - 双线性插值(默认使用)
INTER_AREA - 使用像素区域关系重新采样。它是图像抽取的首选方法,但当图像缩放时,它类似于 INTER_NEAREST 方法。
INTER_CUBIC - 4x4 像素邻域的双三次插值
INTER_LANCZOS4 - 8x8 像素邻域的 Lanczos 插值
首选插值方法是用于缩小的 cv2.INTER_AREA 和用于缩放的 cv2.INTER_CUBIC(慢)和 cv2.INTER_LINEAR。
例子
以下代码将“messi.jpg”图像的大小调整为其原始高度和宽度的一半。
import numpy as np import cv2 img = cv2.imread('messi.JPG',1) height, width = img.shape[:2] res = cv2.resize(img,(int(width/2), int(height/2)), interpolation = cv2.INTER_AREA) cv2.imshow('image',res) cv2.waitKey(0) cv2.destroyAllWindows()
输出

旋转图像
OpenCV 使用仿射变换函数对图像进行平移和旋转等操作。仿射变换是一种可以用矩阵乘法(线性变换)和向量加法(平移)的形式表示的变换。
cv2 模块提供了两个函数cv2.warpAffine和cv2.warpPerspective,您可以使用它们进行各种转换。cv2.warpAffine 采用 2x3 变换矩阵,而 cv2.warpPerspective 采用 3x3 变换矩阵作为输入。
为了找到用于旋转的变换矩阵,OpenCV 提供了一个函数cv2.getRotationMatrix2D,如下所示 -
getRotationMatrix2D(center, angle, scale)
然后,我们将warpAffine函数应用于 getRotationMatrix2D() 函数返回的矩阵以获得旋转图像。
以下程序将原始图像旋转 90 度而不改变尺寸 -
例子
import numpy as np import cv2 img = cv2.imread('OpenCV_Logo.png',1) h, w = img.shape[:2] center = (w / 2, h / 2) mat = cv2.getRotationMatrix2D(center, 90, 1) rotimg = cv2.warpAffine(img, mat, (h, w)) cv2.imshow('original',img) cv2.imshow('rotated', rotimg) cv2.waitKey(0) cv2.destroyAllWindows()
输出
原始图像

旋转图像
