
- OpenCV 教程
- OpenCV - 主页
- OpenCV - 概述
- OpenCV - 环境
- OpenCV - 存储图像
- OpenCV - 读取图像
- OpenCV - 写入图像
- OpenCV-图形用户界面
- 绘图功能
- OpenCV - 画一个圆
- OpenCV - 画一条线
- OpenCV - 绘制矩形
- OpenCV - 绘制椭圆
- OpenCV - 绘制折线
- OpenCV - 绘制凸折线
- OpenCV - 绘制箭头线
- OpenCV - 添加文本
- 过滤
- OpenCV - 双边滤波器
- OpenCV - 盒式过滤器
- OpenCV - SQRBox 滤波器
- OpenCV - Filter2D
- OpenCV - 膨胀
- OpenCV - 侵蚀
- OpenCV - 形态运算
- OpenCV - 图像Pyramid
- 摄像头和人脸检测
- OpenCV - 使用相机
- OpenCV - 图片中的人脸检测
- 使用相机进行人脸检测
- OpenCV 有用资源
- OpenCV - 快速指南
- OpenCV - 有用的资源
- OpenCV - 讨论
OpenCV - 灰度到二进制
您可以使用上一章中提到的相同方法将灰度图像转换为二值图像。只需将灰度图像的路径作为该程序的输入传递即可。
例子
以下程序演示了如何将灰度图像读取为二进制图像并使用 JavaFX 窗口显示它。
import java.awt.image.BufferedImage; import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; import javafx.application.Application; import javafx.embed.swing.SwingFXUtils; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.image.ImageView; import javafx.scene.image.WritableImage; import javafx.stage.Stage; public class GrayScaleToBinary extends Application { @Override public void start(Stage stage) throws Exception { WritableImage writableImage = loadAndConvert(); // Setting the image view ImageView imageView = new ImageView(writableImage); // Setting the position of the image imageView.setX(10); imageView.setY(10); // Setting the fit height and width of the image view imageView.setFitHeight(400); imageView.setFitWidth(600); // Setting the preserve ratio of the image view imageView.setPreserveRatio(true); // Creating a Group object Group root = new Group(imageView); // Creating a scene object Scene scene = new Scene(root, 600, 400); // Setting title to the Stage stage.setTitle("Grayscale to binary image"); // Adding scene to the stage stage.setScene(scene); // Displaying the contents of the stage stage.show(); } public WritableImage loadAndConvert() throws Exception { // Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); // Instantiating the imagecodecs class Imgcodecs imageCodecs = new Imgcodecs(); String input = "E:/OpenCV/chap7/grayscale.jpg"; // Reading the image Mat src = imageCodecs.imread(input); // Creating the destination matrix Mat dst = new Mat(); // Converting to binary image... Imgproc.threshold(src, dst, 200, 500, Imgproc.THRESH_BINARY); // Extracting data from the transformed image (dst) byte[] data1 = new byte[dst.rows() * dst.cols() * (int)(dst.elemSize())]; dst.get(0, 0, data1); // Creating Buffered image using the data BufferedImage bufImage = new BufferedImage(dst.cols(),dst.rows(), BufferedImage.TYPE_BYTE_BINARY); // Setting the data elements to the image bufImage.getRaster().setDataElements(0, 0, dst.cols(), dst.rows(), data1); // Creating a Writable image WritableImage writableImage = SwingFXUtils.toFXImage(bufImage, null); System.out.println("Converted to binary"); return writableImage; } public static void main(String args[]) throws Exception { launch(args); } }
输入图像
假设以下是上述程序中指定的输入图像sample.jpg 。
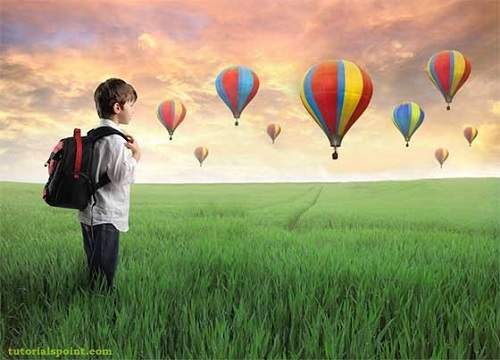
输出图像
执行程序时,您将得到以下输出。
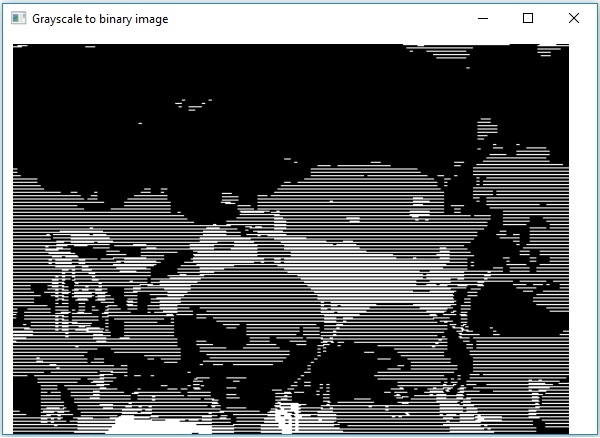